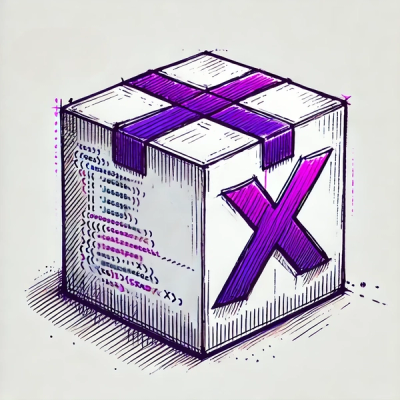
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@tinymce/tinymce-react
Advanced tools
@tinymce/tinymce-react is a React component for TinyMCE, a popular WYSIWYG (What You See Is What You Get) editor. It allows developers to integrate the TinyMCE editor into their React applications easily, providing a rich text editing experience with a wide range of features and customization options.
Basic Integration
This code demonstrates how to integrate the TinyMCE editor into a React application with basic configuration. The editor is initialized with some default content and a set of plugins and toolbar options.
import React from 'react';
import { Editor } from '@tinymce/tinymce-react';
function App() {
return (
<Editor
initialValue="<p>This is the initial content of the editor</p>"
init={{
height: 500,
menubar: false,
plugins: [
'advlist autolink lists link image charmap print preview anchor',
'searchreplace visualblocks code fullscreen',
'insertdatetime media table paste code help wordcount'
],
toolbar:
'undo redo | formatselect | bold italic backcolor | \
alignleft aligncenter alignright alignjustify | \
bullist numlist outdent indent | removeformat | help'
}}
/>
);
}
export default App;
Handling Editor Events
This code shows how to handle events from the TinyMCE editor. The `handleEditorChange` function logs the updated content to the console whenever the content of the editor changes.
import React from 'react';
import { Editor } from '@tinymce/tinymce-react';
function App() {
const handleEditorChange = (content, editor) => {
console.log('Content was updated:', content);
};
return (
<Editor
initialValue="<p>This is the initial content of the editor</p>"
init={{
height: 500,
menubar: false,
plugins: [
'advlist autolink lists link image charmap print preview anchor',
'searchreplace visualblocks code fullscreen',
'insertdatetime media table paste code help wordcount'
],
toolbar:
'undo redo | formatselect | bold italic backcolor | \
alignleft aligncenter alignright alignjustify | \
bullist numlist outdent indent | removeformat | help'
}}
onEditorChange={handleEditorChange}
/>
);
}
export default App;
Customizing the Toolbar
This code demonstrates how to customize the toolbar in the TinyMCE editor. A custom button is added to the toolbar, which shows an alert when clicked.
import React from 'react';
import { Editor } from '@tinymce/tinymce-react';
function App() {
return (
<Editor
initialValue="<p>This is the initial content of the editor</p>"
init={{
height: 500,
menubar: false,
plugins: [
'advlist autolink lists link image charmap print preview anchor',
'searchreplace visualblocks code fullscreen',
'insertdatetime media table paste code help wordcount'
],
toolbar:
'myCustomToolbarButton | undo redo | formatselect | bold italic backcolor | \
alignleft aligncenter alignright alignjustify | \
bullist numlist outdent indent | removeformat | help',
setup: (editor) => {
editor.ui.registry.addButton('myCustomToolbarButton', {
text: 'My Button',
onAction: () => alert('Button clicked!')
});
}
}}
/>
);
}
export default App;
React-Quill is a React component for Quill, a powerful, rich text editor. It offers a similar WYSIWYG editing experience and is known for its simplicity and ease of use. Compared to @tinymce/tinymce-react, React-Quill has fewer built-in plugins but is highly customizable through its API.
Draft.js is a framework for building rich text editors in React, developed by Facebook. It provides a lot of flexibility and control over the editor's behavior and appearance. Unlike @tinymce/tinymce-react, Draft.js is more of a low-level tool, requiring more setup and configuration to achieve similar functionality.
CKEditor 4 React is a React component for CKEditor 4, another popular WYSIWYG editor. It offers a wide range of features and plugins similar to TinyMCE. CKEditor 4 is known for its robust feature set and extensive documentation, making it a strong alternative to @tinymce/tinymce-react.
This package is a thin wrapper around TinyMCE to make it easier to use in a React application.
Have you found an issue with tinymce-react or do you have a feature request? Open up an issue and let us know or submit a pull request. Note: For issues concerning TinyMCE please visit the TinyMCE repository.
FAQs
Official TinyMCE React Component
The npm package @tinymce/tinymce-react receives a total of 213,069 weekly downloads. As such, @tinymce/tinymce-react popularity was classified as popular.
We found that @tinymce/tinymce-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.