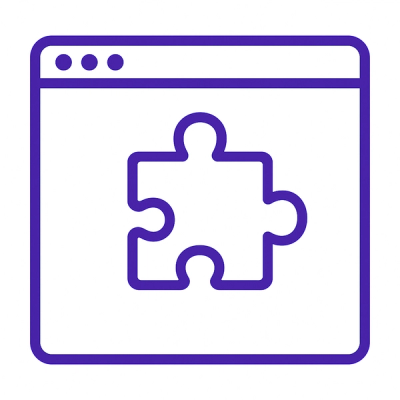
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
@toolz/allow-react
Advanced tools
allow-react
is a library that checks data types and allows the script to continue if they pass the check. If the check fails, the script can throw an Error
, or emit a warning, or invoke a custom callback. The package was written to ensure that only the "right" kind of data is allowed into the body of a function / method / component / etc. The intention is to provide effective runtime validation of data before it reaches application logic.
Another goal is to eliminate the need for unit tests that only test a function's / method's operation when it receives the "wrong" kind of data. By validating that data at runtime, we are ensuring that the internal logic of the function / method is only invoked if it has been supplied with data of the expected type.
NOTE: This library is an extension of @toolz/allow
, designed to handle specific cases where React elements need to be checked. For this reason, the react
library is a core dependency of this package. But it does not exist in @toolz/allow
. If you are not working in a React project, or if you don't feel the need to specifically check for React elements, it's recommended to use the core @toolz/allow
package.
After installation, import the package:
import { allow } from '@toolz/allow-react';
Once imported, the assumed usage is directly after the entry to any function / method / component / etc. The idea is to check the integrity of provided inputs before further computation proceeds. This would typically look like this:
const addSalesTax = originalPrice => {
allow.aNumber(originalPrice, 0);
/*
...proceed with the rest of the function
*/
}
In the above example, the assumption is that originalPrice
should always be a number. If any other data type is provided for originalPrice
, the allow
check will fail. This means that a value of '32.99'
will fail (because it's a string). null
will fail. Boolean values will fail. Anything that is not a number will fail. In this example, the second argument (which is optional), indicates the minimum acceptable value of the number. In this case, we don't want negative values for originalPrice
, so nothing below 0
will pass the check.
const doSomething = someValue => {
allow.aBoolean(someValue);
/*
This is NOT "truthy". It fails if anything other than a Boolean is
provided. This means that it fails on 'TRUE'/'FALSE' (because they're
strings), on 1/0 (because they're numbers), or any other value that is
not a pure TRUE/FALSE
*/
}
const doSomething = theCallback => {
allow.aFunction(theCallback);
/*
This will fail unless a function is provided as the value for
theCallback. It doesn't care what's inside the function. It can even be
blank, like:
() => {}
But it must be a function of some kind.
Please note that, in JavaScript, a class is virtually indistinguishable
from a function (since "class" is just syntactic sugar). For this reason,
a class will also pass this check.
*/
}
const doSomething = theArray => {
allow.anArray(theArray);
/*
This will fail unless an array is provided as the value for theArray.
The check doesn't examine the contents of the array. It can be an
empty array, like:
[]
But it must be an array of some kind.
*/
}
const doSomething = theArray => {
allow.anArray(theArray, 1);
/*
The second argument of anArray() is the minimum length of the array.
So, by setting this value to 1, it ensures that theArray is a
non-empty array.
*/
}
const doSomething = theArray => {
allow.anArray(theArray, 2, 50);
/*
This ensures that theArray is an array, that has no fewer than 2
elements, and no more than 50 elements.
*/
}
const doSomething = theArrays => {
allow.anArrayOfArrays(theArrays);
/*
This will fail unless an array is provided as the value for
theArrays. It will also fail if any of elements inside theArrays
are not also arrays. It does not inspect the contents of the arrays
inside theArrays. They can be empty arrays, like:
[[], [], []]
But they must be arrays of some kind.
*/
}
const doSomething = theArrays => {
allow.anArrayOfArrays(theArrays, 1);
/*
The second argument of anArrayOfArrays() is the minimum length of the
array. So, by setting this value to 1, it ensures that theArrays is
a non-empty array-of-arrays.
*/
}
const doSomething = theArrays => {
allow.anArrayOfArrays(theArrays, 2, 50);
/*
This ensures that theArrays is an array, that all of its elements
are arrays, that it has no fewer than 2 elements, and no more than 50
elements.
*/
}
const person = {
firstName: '',
lastName: '',
middleInitial: '',
}
const doSomething = thePeople => {
allow.anArrayOfInstances(thePeople, person);
/*
This will fail unless an array is provided as the value for
thePeople. It will also fail if any of the elements inside thePeople
are not objects, and if those objects do not have all the keys present
in the model object (in this case: person). It does not inspect the
types of data held in those keys, and it will allow additional keys
that do not exist in the model object. But it expects every object
in the array to have all of the keys present in the model.
*/
}
const person = {
firstName: '',
lastName: '',
middleInitial: '',
}
const doSomething = thePeople => {
allow.anArrayOfInstances(thePeople, person, 1);
/*
The third argument of anArrayOfInstances() is the minimum length of
the array. So, by setting this value to 1, it ensures that thePeople is
a non-empty array of "person" instances.
*/
}
const person = {
firstName: '',
lastName: '',
middleInitial: '',
}
const doSomething = thePeople => {
allow.anArrayOfInstances(thePeople, person, 2, 50);
/*
This ensures that thePeople is an array of "person" instances, that it
has no fewer than 2 elements, and no more than 50 elements.
*/
}
const doSomething = theNumbers => {
allow.anArrayOfIntegers(theNumbers);
/*
This will fail unless an array is provided as the value for
theNumbers. It will also fail if any of elements inside theNumbers
are not also integers. This means that it will fail on any non-numeric
value, and it will also fail on any number that is not a "whole"
number. It will accept decimal values, as long as those decimals
evaluate to a whole number. So this works:
[1.0, 3.00, 42.0]
But this does not:
[1.0, 3.14, 42.0]
*/
}
const doSomething = theNumbers => {
allow.anArrayOfIntegers(theNumbers, 1);
/*
The second argument of anArrayOfIntegers() is the minimum length of
the array. So, by setting this value to 1, it ensures that theNumbers
is a non-empty array of integers.
*/
}
const doSomething = theNumbers => {
allow.anArrayOfIntegers(theNumbers, 2, 50);
/*
This ensures that theNumbers is an array of integers, that it has no
fewer than 2 elements, and no more than 50 elements.
*/
}
const doSomething = theNumbers => {
allow.anArrayOfNumbers(theNumbers);
/*
This will fail unless an array is provided as the value for
theNumbers. It will also fail if any of elements inside theNumbers
are not also numbers.
*/
}
const doSomething = theNumbers => {
allow.anArrayOfNumbers(theNumbers, 1);
/*
The third argument of anArrayOfNumbers() is the minimum length of
the array. So, by setting this value to 1, it ensures that theNumbers
is a non-empty array of integers.
*/
}
const doSomething = theNumbers => {
allow.anArrayOfNumbers(theNumbers, 2, 50);
/*
This ensures that theNumbers is an array of integers, that it has no
fewer than 2 elements, and no more than 50 elements.
*/
}
const doSomething = theObjects => {
allow.anArrayOfObjects(theObjects);
/*
This will fail unless an array is provided as the value for
theObjects. It will also fail if any of the elements inside theObjects
are not also objects. It does not inspect the contents of the objects
inside theObjects. They can be empty objects, like:
[{}, {}, {}]
But they must be objects of some kind.
Technically speaking, React elements are objects but they may need to be
treated differently, due to their special nature. For that reason, this
check will fail if theObjects contains React elements.
*/
}
const doSomething = theObjects => {
allow.anArrayOfObjects(theObjects, 1);
/*
The second argument of anArrayOfObjects() is the minimum length of the
array. So, by setting this value to 1, it ensures that theObjects is
a non-empty array-of-objects.
*/
}
const doSomething = theObjects => {
allow.anArrayOfObjects(theObjects, 2, 50);
/*
This ensures that theObjects is an array, that all of its elements
are objects, that it has no fewer than 2 elements, and no more than 50
elements.
*/
}
const doSomething = theElements => {
allow.anArrayOfReactElements(theElements);
/*
This will fail unless an array is provided as the value for
theElements. It will also fail if any of the elements inside theElements
are not also React elements. It does not inspect the contents of the
elements inside theElements. They can be empty elements, like:
[<></>, <></>, <></>]
But they must be elements of some kind.
*/
}
const doSomething = theStrings => {
allow.anArrayOfStrings(theStrings);
/*
This will fail unless an array is provided as the value for
theStrings. It will also fail if any of the elements inside theStrings
are not also strings. It does not inspect the contents of the strings
inside theStrings. They can be empty strings, like:
['', '', '']
But they must be strings of some kind.
*/
}
const doSomething = theStrings => {
allow.anArrayOfStrings(theStrings, 1);
/*
The second argument of anArrayOfStrings() is the minimum length of the
array. So, by setting this value to 1, it ensures that theStrings is
a non-empty array-of-strings.
*/
}
const doSomething = theStrings => {
allow.anArrayOfStrings(theStrings, 2, 50);
/*
This ensures that theStrings is an array, that all of its elements
are strings, that it has no fewer than 2 elements, and no more than 50
elements.
*/
}
const person = {
firstName: '',
lastName: '',
middleInitial: '',
}
const doSomething = thePerson => {
allow.anInstanceOf(thePerson, person);
/*
This is a (purposely forgiving) check to ensure that the provided object
is of a similar type to a reference object. This only checks that the
provided object has all of the same keys that are present in the
reference object. It does not look at the types of data held in those
keys. It allows additional keys that don't exist in the reference
object. These objects will pass the check against the person reference:
{
firstName: 'Bob',
lastName: 'Doe',
middleInitial: null, // this does not check to ensure that
// middleInitial is a string
}
{
firstName: 'Bob',
lastName: 'Doe',
middleInitial: 'K',
favoriteIceCream: 'vanilla' // the check does not fail based upon any
// "extra" keys
}
This object will fail the check against the person reference:
{
firstName: 'Bob',
lastName: 'Doe',
// the middleInitial key is missing
}
*/
}
const person = {
firstName: '',
lastName: '',
middleInitial: '',
address: {},
children: [],
}
const doSomething = thePerson => {
allow.anInstanceOf(thePerson, person);
/*
Although anInstanceOf() does not check data types (string, number,
etc.), it does check to ensure that keys containing objects or
arrays in the model object also contain objects or arrays in the
supplied object. This object will fails the check against the person
reference:
{
firstName: 'Bob',
lastName: 'Doe',
middleInitial: 'I',
address: '101 Main Street',
children: 4,
}
This check is not recursive, meaning that anInstanceOf() only checks
to ensure that address is an object and children is an array. It makes
no attempt to ensure that any objects/arrays below the first level of
keys exist in the supplied object.
*/
}
const doSomething = theNumber => {
allow.anInteger(theNumber);
/*
This will fail unless an integer is provided as the value for
theNumber. It will fail on any non-numeric value. It will also
fail on any numbers that are not "whole" numbers. Values like
1.00 or 42.0 are fine. But 3.14 will fail, because it is not
an integer.
*/
}
const doSomething = theNumber => {
allow.anInteger(theNumber, 0);
/*
The second argument of anInteger() is the minimum value of theNumber.
So, by setting this value to 0, it ensures that theNumber is a
non-negative integer.
*/
}
const doSomething = theNumber => {
allow.anInteger(theNumber, 2, 50);
/*
This ensures that theNumber is an integer, that the value is no less
than 2, and no greater than 50.
*/
}
const doSomething = theObject => {
allow.anObject(theObject);
/*
This ensures that theObject is an object. In JavaScript, an array is
seen as having typeof 'object', but an array will fail this check.
Similarly, NULL is seen as having typeof 'object', but it will also
fail this check.
Technically speaking, a React element is an object but it may need to be
treated differently, due to its special nature. For that reason, this
check will fail if theObject is a React element.
*/
}
const doSomething = theObject => {
allow.anObject(theObject, 1);
/*
The second argument of anObject() is the minimum number of keys that
must be present in theObject. So, by setting this value to 1, it ensures
that theObject is a non-empty object.
*/
}
const doSomething = theObject => {
allow.anObject(theObject, 2, 50);
/*
This ensures that theObject is an object, with no fewer than two keys,
and no more than 50 keys.
*/
}
const doSomething = theElement => {
allow.aReactElement(theElement);
/*
This ensures that theElement is a React element. A React element has
typeof 'object', but a regular object will fail this check.
*/
}
const doSomething = theString => {
allow.aString(theString);
/*
This will fail unless a string is provided as the value for theString.
The check doesn't examine the contents of the string. It can be an
empty string, like:
''
But it must be a string of some kind.
*/
}
const doSomething = theString => {
allow.aString(theString, 1);
/*
The second argument of aString() is the minimum length of the string.
So, by setting this value to 1, it ensures that theString is a
non-empty string.
*/
}
const doSomething = theString => {
allow.aString(theString, 2, 50);
/*
This ensures that theString is a string, that has no fewer than 2
characters, and no more than 50 characters.
*/
}
console.log(allow.getAllowNull()); // false
/*
allowNull is Boolean. The default value is FALSE. When set to
TRUE, all of the checks will pass if the provided value is NULL.
*/
console.log(allow.getFailureBehavior()); // 'throw'
/*
The behavior has three possible values:
throw
warn
ignore
'throw' is the default value.
*/
allow.getOnFailure();
/*
returns whatever function has been set to be called onFailure
*/
This can be called two different ways - by passing in a reference array or a reference object.
const days = ['Sun', 'Mon', 'Tue', 'Wed', 'Thur', 'Fri', 'Sat'];
const doSomething = theDay => {
allow.oneOf(theDay, days);
/*
This ensures that theDay matches one of the values in the days array.
*/
}
const days = {
0: 'Sun',
1: 'Mon',
2: 'Tue',
3: 'Wed',
4: 'Thur',
5: 'Fri',
6: 'Sat',
}
const doSomething = theDay => {
allow.oneOf(theDay, days);
/*
This ensures that theDay matches one of the values in the days object.
It does not try to check against any of the keys in the key/value pairs.
It only checks against the values.
*/
}
The first value passed into oneOf()
must be a primitive. It cannot be an object, an array, a function, or a React element.
const thisIsNull = null;
allow.anArray(thisIsNull); // this throws an Error
allow.setAllowNull(true);
allow.anArray(thisIsNull); // this does NOT throw an Error
/*
setAllowNull() requires a Boolean as its only argument. By default, the
values checked in by the allow methods are not nullable. But this behavior
can be toggled with setAllowNull().
If localStorage is available, the allowNull value will be set there.
This allows the setting to be saved once in the lifecycle of the app.
*/
allow.setFailureBehavior(allow.failureBehavior.WARN);
/*
setFailureBehavior() requires one of the following three values:
'throw'
'warn'
'ignore'
'throw' is the default value. Throwing an Error will halt JavaScript
execution.
'warn' will spawn warnings to be displayed in the console.
'ignore' will turn off all warnings and cease the throwing of all Errors.
If localStorage is available, the failureBehavior value will be set there.
This allows the setting to be saved once in the lifecycle of the app.
*/
const myCustomErrorHandler = (value, message) => {
// do the custom error handling...
}
allow.setFailureBehavior(allow.failureBehavior.IGNORE);
allow.setOnFailure(myCustomErrorHandler);
/*
The onFailure handler is called before any warning is displayed and before
any Error is thrown. Setting an onFailure callback does not halt the
further error handling provide by allow. If you want to turn off
those features, you must use setFailureBehavior().
When the onFailure method is invoked, it will be called with two arguments:
The original value that failed validation
The message spawned by that failed validation
*/
A successful call to any of the allow
validation methods always returns allow
. This enables the chaining of multiple checks on a single line of code. That would look something like this:
const doSomething = (patient, isAlive, age) => {
allow.anObject(patient, 1).aBoolean(isAlive).aNumber(age, 0, 130);
/*
This ensures that patient is a non-empty object
AND that isAlive is a Boolean
AND that age is a non-negative number no greater than 130
*/
}
FAQs
Extends @toolz/allow to provide validation of React elements
The npm package @toolz/allow-react receives a total of 1 weekly downloads. As such, @toolz/allow-react popularity was classified as not popular.
We found that @toolz/allow-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.