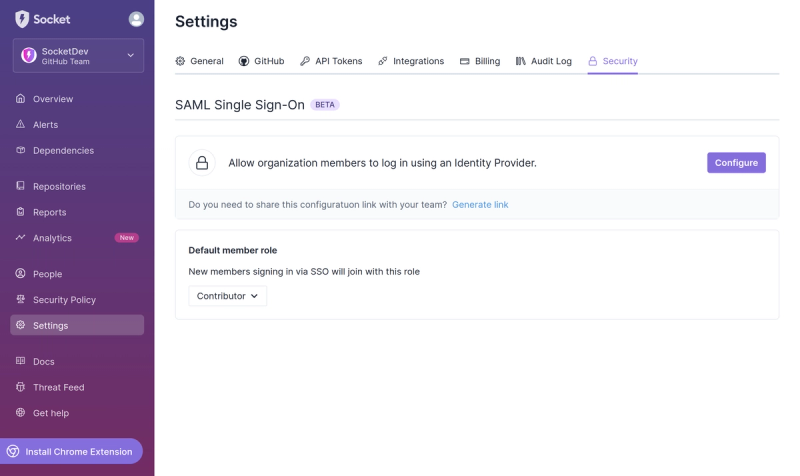
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@toolz/create-random-id
Advanced tools
Readme
create-random-id
is a tiny utility function to generate random IDs (strings). It uses Math.random()
, and as such, it provides no level of cryptographic security. But it should be perfectly fine whenever you simply need to generate a pseudo-random string.
After installation, import the package:
import { createRandomId } from '@toolz/create-random-id';
createRandomId()
generates a random string. The first character in the string will be a letter (unless useUppercaseLetters
and useLowercaseLetters
are both set to FALSE
). All subsequent characters will be uppercase letters (unless useUppercaseLetters
is set to FALSE
), lowercase letters (unless useLowercaseLetters
is set to FALSE
), and numbers (unless useNumbers
is set to FALSE
).
If useUppercaseLetters
, useLowercaseLetters
and useNumbers
are all set to FALSE
, the function will ignore the settings and generate a string containing uppercase letters, lowercase letters, and numbers.
const API = {
arguments: {
length: {
optional,
format: 'positive integer',
defaultValue: 32,
},
useUppercaseLetters: {
optional,
format: Boolean,
defaultValue: true,
},
useLowercaseLetters: {
optional,
format: Boolean,
defaultValue: true,
},
useNumbers: {
optional,
format: Boolean,
defaultValue: true,
},
},
returns: string,
}
Examples:
createRandomId(); // MJYeNOFFhx52AmBuzo5YC2yN2TPN3N1O
createRandomId(100); // wSLOH1nZ6WdCy7mHLwBOPoK08250fHN8xe8ipNkT07MjJFU5u55uPrIYs80OQZMmoUakM8Mfn1l8ue2AikKoRciTZFS2O74i1lQM
createRandomId(32, false); // udu3dhp2ht8dxtf38o5l1o8wspiymrgk
createRandomId(32, false, false); // 58388737442364071155558324301586
createRandomId(32, false, false, false); // ebWpxLSF7aQkGHIItKPqNNmOGZhFeNot
createRandomId(32, true, false); // XCZMJCYINK4UYIUABPIXX2I1DBX44R1C
createRandomId(32, true, true, false); // pndomtXQXjPlHbfixGKEchlgiqGWAGuQ
FAQs
A simple utility to generate random IDs
The npm package @toolz/create-random-id receives a total of 10 weekly downloads. As such, @toolz/create-random-id popularity was classified as not popular.
We found that @toolz/create-random-id demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.