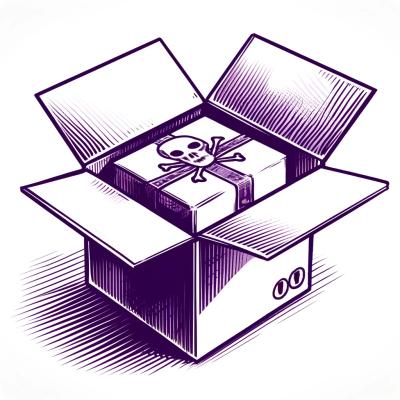
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@turf/bbox-clip
Advanced tools
@turf/bbox-clip is a module from the Turf.js library that allows you to clip a GeoJSON feature to a bounding box. This is useful for tasks such as extracting a specific area from a larger dataset, ensuring that features fit within a certain geographic region, or preparing data for visualization.
Clip a Polygon to a Bounding Box
This feature allows you to clip a polygon to a specified bounding box. The code sample demonstrates how to clip a polygon that spans a larger area to fit within the bounding box defined by the coordinates [-3, 53, 3, 55].
const turf = require('@turf/turf');
const polygon = turf.polygon([[
[-5, 52], [-5, 56], [5, 56], [5, 52], [-5, 52]
]]);
const bbox = [-3, 53, 3, 55];
const clipped = turf.bboxClip(polygon, bbox);
console.log(JSON.stringify(clipped));
Clip a LineString to a Bounding Box
This feature allows you to clip a LineString to a specified bounding box. The code sample demonstrates how to clip a LineString that spans a larger area to fit within the bounding box defined by the coordinates [-3, 53, 3, 55].
const turf = require('@turf/turf');
const line = turf.lineString([
[-5, 52], [-5, 56], [5, 56], [5, 52]
]);
const bbox = [-3, 53, 3, 55];
const clipped = turf.bboxClip(line, bbox);
console.log(JSON.stringify(clipped));
Clip a MultiPolygon to a Bounding Box
This feature allows you to clip a MultiPolygon to a specified bounding box. The code sample demonstrates how to clip a MultiPolygon that spans a larger area to fit within the bounding box defined by the coordinates [-3, 53, 3, 55].
const turf = require('@turf/turf');
const multiPolygon = turf.multiPolygon([[
[[-5, 52], [-5, 56], [5, 56], [5, 52], [-5, 52]]
], [
[[-10, 50], [-10, 54], [0, 54], [0, 50], [-10, 50]]
]]);
const bbox = [-3, 53, 3, 55];
const clipped = turf.bboxClip(multiPolygon, bbox);
console.log(JSON.stringify(clipped));
The jsts (JavaScript Topology Suite) library offers a wide range of geospatial operations, including clipping geometries to a bounding box. While it provides more comprehensive geospatial processing capabilities, it is also more complex and has a steeper learning curve compared to @turf/bbox-clip.
Takes a Feature and a bbox and clips the feature to the bbox using lineclip. May result in degenerate edges when clipping Polygons.
feature
Feature<(LineString | MultiLineString | Polygon | MultiPolygon)> feature to clip to the bboxbbox
BBox extent in [minX, minY, maxX, maxY] ordervar bbox = [0, 0, 10, 10];
var poly = turf.polygon([[[2, 2], [8, 4], [12, 8], [3, 7], [2, 2]]]);
var clipped = turf.bboxClip(poly, bbox);
//addToMap
var addToMap = [bbox, poly, clipped]
Returns Feature<(LineString | MultiLineString | Polygon | MultiPolygon)> clipped Feature
This module is part of the Turfjs project, an open source module collection dedicated to geographic algorithms. It is maintained in the Turfjs/turf repository, where you can create PRs and issues.
Install this single module individually:
$ npm install @turf/bbox-clip
Or install the all-encompassing @turf/turf module that includes all modules as functions:
$ npm install @turf/turf
FAQs
turf bbox-clip module
We found that @turf/bbox-clip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.