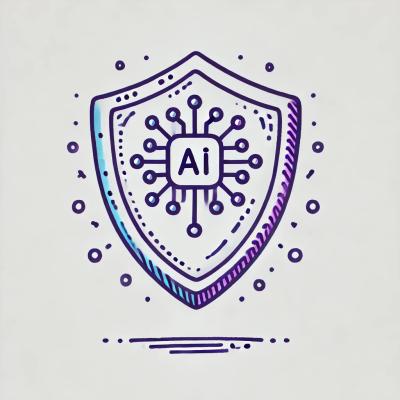
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@types/body-parser
Advanced tools
TypeScript definitions for body-parser
The @types/body-parser package contains TypeScript definitions for the body-parser package. Body-parser is a middleware used in Express applications to parse incoming request bodies before your handlers, available under the req.body property. It supports parsing of JSON, raw, text, and URL-encoded form bodies. The @types/body-parser package doesn't provide functionality by itself but offers type definitions to enable TypeScript developers to use body-parser in a type-safe manner.
JSON Body Parsing
Parses incoming request bodies in a middleware before your handlers, available under the req.body property, assuming the Content-Type header of the request is application/json.
import * as bodyParser from 'body-parser';
import express from 'express';
const app = express();
app.use(bodyParser.json());
app.post('/json', (req, res) => {
res.send(req.body);
});
URL-Encoded Form Body Parsing
Parses incoming request bodies with URL-encoded data (like form submissions) before your handlers, making the form data available under the req.body property.
import * as bodyParser from 'body-parser';
import express from 'express';
const app = express();
app.use(bodyParser.urlencoded({ extended: true }));
app.post('/form', (req, res) => {
res.send(req.body);
});
npm install --save @types/body-parser
This package contains type definitions for body-parser (https://github.com/expressjs/body-parser).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/body-parser.
These definitions were written by Santi Albo, Vilic Vane, Jonathan Häberle, Gevik Babakhani, Tomasz Łaziuk, Jason Walton, and Piotr Błażejewicz.
FAQs
TypeScript definitions for body-parser
The npm package @types/body-parser receives a total of 14,918,129 weekly downloads. As such, @types/body-parser popularity was classified as popular.
We found that @types/body-parser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.