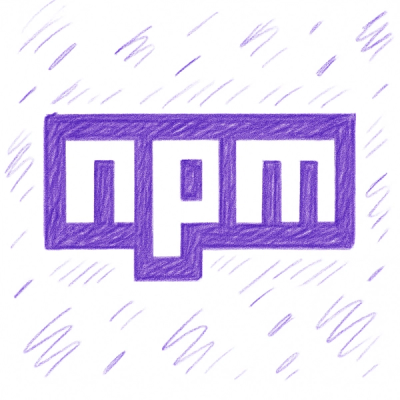
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
@types/cors
Advanced tools
TypeScript definitions for cors
The @types/cors package provides TypeScript type definitions for the cors middleware used in Express/Connect applications. It allows TypeScript developers to use cors in their projects with the benefits of type checking and IntelliSense in their IDE. This package does not contain functionality by itself but offers type definitions to enhance development experience when using the cors package.
CORS Configuration
This code sample demonstrates how to configure CORS policy for an Express application. It specifies that only requests from 'http://example.com' are allowed and sets a successful status code for preflight requests.
{ "origin": "http://example.com", "optionsSuccessStatus": 200 }
Enabling CORS for a Single Route
This example shows how to enable CORS for a specific route in an Express application. It uses the cors middleware directly in the route definition.
app.get('/products/:id', cors(), function (req, res, next) { res.json({msg: 'This is CORS-enabled for a Single Route'}); })
Helmet is a middleware for Express applications that helps secure your apps by setting various HTTP headers, not directly related to CORS but contributes to overall security. Unlike @types/cors, which provides type definitions for CORS configuration, Helmet focuses on a broader range of security features.
Express-rate-limit is a middleware to help protect your app from brute-force attacks, which is different from what @types/cors offers. While @types/cors focuses on type definitions for handling cross-origin requests, express-rate-limit provides functionality to limit repeated requests to public APIs and endpoints.
npm install --save @types/cors
This package contains type definitions for cors (https://github.com/expressjs/cors/).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/cors.
/// <reference types="node" />
import { IncomingHttpHeaders } from "http";
type StaticOrigin = boolean | string | RegExp | Array<boolean | string | RegExp>;
type CustomOrigin = (
requestOrigin: string | undefined,
callback: (err: Error | null, origin?: StaticOrigin) => void,
) => void;
declare namespace e {
interface CorsRequest {
method?: string | undefined;
headers: IncomingHttpHeaders;
}
interface CorsOptions {
/**
* @default '*'
*/
origin?: StaticOrigin | CustomOrigin | undefined;
/**
* @default 'GET,HEAD,PUT,PATCH,POST,DELETE'
*/
methods?: string | string[] | undefined;
allowedHeaders?: string | string[] | undefined;
exposedHeaders?: string | string[] | undefined;
credentials?: boolean | undefined;
maxAge?: number | undefined;
/**
* @default false
*/
preflightContinue?: boolean | undefined;
/**
* @default 204
*/
optionsSuccessStatus?: number | undefined;
}
type CorsOptionsDelegate<T extends CorsRequest = CorsRequest> = (
req: T,
callback: (err: Error | null, options?: CorsOptions) => void,
) => void;
}
declare function e<T extends e.CorsRequest = e.CorsRequest>(
options?: e.CorsOptions | e.CorsOptionsDelegate<T>,
): (
req: T,
res: {
statusCode?: number | undefined;
setHeader(key: string, value: string): any;
end(): any;
},
next: (err?: any) => any,
) => void;
export = e;
These definitions were written by Alan Plum, Gaurav Sharma, and Sebastian Beltran.
FAQs
TypeScript definitions for cors
The npm package @types/cors receives a total of 8,944,494 weekly downloads. As such, @types/cors popularity was classified as popular.
We found that @types/cors demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.