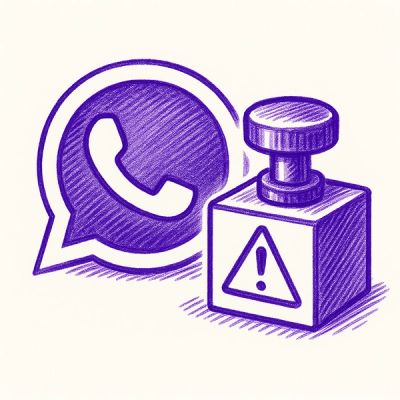
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
@types/form-data
Advanced tools
Stub TypeScript definitions entry for form-data, which provides its own types definitions
@types/form-data provides TypeScript type definitions for the form-data library, which is used to create and manipulate form data, especially for HTTP requests. It allows developers to handle form data in a type-safe manner.
Creating Form Data
This feature allows you to create a new FormData instance and append key-value pairs to it. This is useful for constructing form data to be sent in HTTP requests.
const FormData = require('form-data');
const form = new FormData();
form.append('my_field', 'my_value');
Appending Files
This feature allows you to append files to the form data. This is particularly useful for file upload scenarios where you need to send files as part of an HTTP request.
const fs = require('fs');
const FormData = require('form-data');
const form = new FormData();
form.append('file', fs.createReadStream('/path/to/file'));
Setting Headers
This feature allows you to get the headers that should be included in the HTTP request when sending the form data. This is important for ensuring that the server correctly interprets the form data.
const FormData = require('form-data');
const form = new FormData();
const headers = form.getHeaders();
The form-data package is the underlying library for which @types/form-data provides type definitions. It allows you to create and manipulate form data for HTTP requests. It is widely used in Node.js applications for handling multipart/form-data.
Axios is a promise-based HTTP client for the browser and Node.js. It can handle form data by using the FormData API. While it is not specifically focused on form data, it provides a comprehensive solution for making HTTP requests, including handling form data.
The request package is a simplified HTTP client for Node.js. It can handle form data by using the form-data library internally. Although the request package is deprecated, it is still widely used in legacy codebases.
This is a stub types definition for @types/form-data (https://github.com/form-data/form-data#readme).
form-data provides its own type definitions, so you don't need @types/form-data installed!
FAQs
Stub TypeScript definitions entry for form-data, which provides its own types definitions
The npm package @types/form-data receives a total of 994,195 weekly downloads. As such, @types/form-data popularity was classified as popular.
We found that @types/form-data demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.