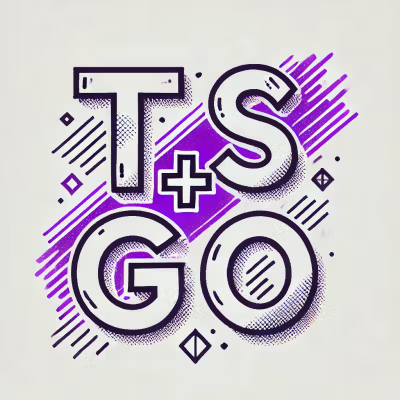
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@types/pino-std-serializers
Advanced tools
Stub TypeScript definitions entry for pino-std-serializers, which provides its own types definitions
@types/pino-std-serializers provides TypeScript type definitions for the pino-std-serializers package, which is used to serialize standard Node.js objects such as HTTP requests, responses, and errors for logging purposes.
HTTP Request Serializer
This feature allows you to serialize HTTP request objects for logging. The `asReqValue` function converts the request object into a format suitable for logging.
const pino = require('pino');
const { asReqValue } = require('pino-std-serializers');
const logger = pino({
serializers: {
req: asReqValue
}
});
const req = { method: 'GET', url: '/test', headers: { host: 'localhost' } };
logger.info({ req }, 'Request received');
HTTP Response Serializer
This feature allows you to serialize HTTP response objects for logging. The `asResValue` function converts the response object into a format suitable for logging.
const pino = require('pino');
const { asResValue } = require('pino-std-serializers');
const logger = pino({
serializers: {
res: asResValue
}
});
const res = { statusCode: 200, headers: { 'content-type': 'application/json' } };
logger.info({ res }, 'Response sent');
Error Serializer
This feature allows you to serialize error objects for logging. The `asErrValue` function converts the error object into a format suitable for logging.
const pino = require('pino');
const { asErrValue } = require('pino-std-serializers');
const logger = pino({
serializers: {
err: asErrValue
}
});
const error = new Error('Something went wrong');
logger.error({ err: error }, 'Error occurred');
@types/bunyan provides TypeScript type definitions for the Bunyan logging library. Bunyan is another popular logging library for Node.js that offers similar functionalities, including serializers for HTTP requests, responses, and errors.
@types/winston provides TypeScript type definitions for the Winston logging library. Winston is a versatile logging library for Node.js that also supports custom serializers for various objects, including HTTP requests and responses.
@types/log4js provides TypeScript type definitions for the Log4js logging library. Log4js is another logging library for Node.js that offers customizable logging and serialization capabilities similar to pino-std-serializers.
This is a stub types definition for @types/pino-std-serializers (https://github.com/pinojs/pino-std-serializers#readme).
pino-std-serializers provides its own type definitions, so you don't need @types/pino-std-serializers installed!
FAQs
Stub TypeScript definitions entry for pino-std-serializers, which provides its own types definitions
The npm package @types/pino-std-serializers receives a total of 388,003 weekly downloads. As such, @types/pino-std-serializers popularity was classified as popular.
We found that @types/pino-std-serializers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.