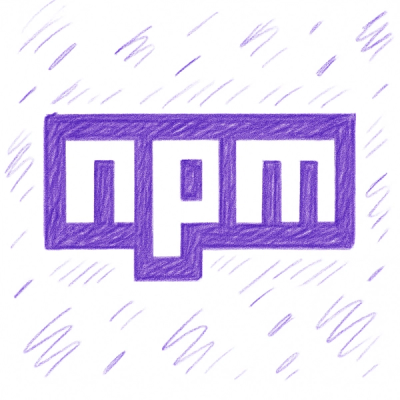
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
@types/react-copy-to-clipboard
Advanced tools
TypeScript definitions for react-copy-to-clipboard
@types/react-copy-to-clipboard provides TypeScript definitions for the react-copy-to-clipboard package, which is a React component for copying text to the clipboard.
Copy Text to Clipboard
This feature allows you to copy text to the clipboard. The example demonstrates a simple input field where the user can type text, and a button to copy that text to the clipboard. When the text is copied, a message is displayed.
import React, { useState } from 'react';
import { CopyToClipboard } from 'react-copy-to-clipboard';
const CopyExample = () => {
const [text, setText] = useState('Hello, World!');
const [copied, setCopied] = useState(false);
return (
<div>
<input value={text} onChange={(e) => setText(e.target.value)} />
<CopyToClipboard text={text} onCopy={() => setCopied(true)}>
<button>Copy to Clipboard</button>
</CopyToClipboard>
{copied ? <span style={{ color: 'red' }}>Copied.</span> : null}
</div>
);
};
export default CopyExample;
react-copy-to-clipboard is the underlying package that @types/react-copy-to-clipboard provides TypeScript definitions for. It offers the same functionality of copying text to the clipboard but without TypeScript support.
clipboard.js is a popular library for copying text to the clipboard. It is not specific to React and can be used in any JavaScript project. It provides more control and customization options compared to react-copy-to-clipboard.
react-clipboard.js is another React component for copying text to the clipboard. It is similar to react-copy-to-clipboard but offers additional features like copying rich text and images.
npm install --save @types/react-copy-to-clipboard
This package contains type definitions for react-copy-to-clipboard (https://github.com/nkbt/react-copy-to-clipboard).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/react-copy-to-clipboard.
import * as React from "react";
export as namespace CopyToClipboard;
declare class CopyToClipboard extends React.PureComponent<CopyToClipboard.Props> {}
declare namespace CopyToClipboard {
class CopyToClipboard extends React.PureComponent<Props> {}
interface Options {
debug?: boolean | undefined;
message?: string | undefined;
format?: string | undefined; // MIME type
}
interface Props {
children?: React.ReactNode;
text: string;
onCopy?(text: string, result: boolean): void;
options?: Options | undefined;
}
}
export = CopyToClipboard;
These definitions were written by Meno Abels, Bernabe, and Ward Delabastita.
FAQs
TypeScript definitions for react-copy-to-clipboard
The npm package @types/react-copy-to-clipboard receives a total of 439,774 weekly downloads. As such, @types/react-copy-to-clipboard popularity was classified as popular.
We found that @types/react-copy-to-clipboard demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.