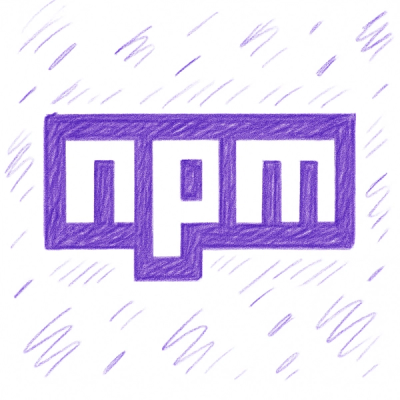
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
@types/react-input-mask
Advanced tools
TypeScript definitions for react-input-mask
@types/react-input-mask provides TypeScript definitions for the react-input-mask library, which is used to create input masks for form fields in React applications. Input masks are used to format user input in a specific way, such as phone numbers, dates, or credit card numbers.
Basic Input Mask
This feature allows you to create a basic input mask for a phone number. The mask format '(999) 999-9999' ensures that the user input follows the specified pattern.
```tsx
import React from 'react';
import InputMask from 'react-input-mask';
const PhoneInput: React.FC = () => {
return (
<InputMask mask="(999) 999-9999" placeholder="Enter phone number" />
);
};
export default PhoneInput;
```
Custom Mask Character
This feature allows you to specify a custom mask character. In this example, the mask format '99/99/9999' is used for a date input, and the mask character is set to '_'.
```tsx
import React from 'react';
import InputMask from 'react-input-mask';
const CustomMaskInput: React.FC = () => {
return (
<InputMask mask="99/99/9999" maskChar="_" placeholder="Enter date" />
);
};
export default CustomMaskInput;
```
Mask with Always Visible Placeholder
This feature allows you to create an input mask where the placeholder is always visible. The mask format '9999 9999 9999 9999' is used for a credit card number input, and the 'alwaysShowMask' property ensures that the mask is always visible.
```tsx
import React from 'react';
import InputMask from 'react-input-mask';
const AlwaysVisiblePlaceholderInput: React.FC = () => {
return (
<InputMask mask="9999 9999 9999 9999" alwaysShowMask={true} placeholder="Enter credit card number" />
);
};
export default AlwaysVisiblePlaceholderInput;
```
react-text-mask is a library for creating input masks in React applications. It provides similar functionality to react-input-mask but offers more flexibility with custom mask definitions and supports both React and Angular. It also has better support for dynamic mask changes.
cleave.js is a JavaScript library for formatting input fields. It supports a wide range of input formats, including credit card numbers, phone numbers, dates, and more. Cleave.js can be used with React through the react-cleave component, providing similar functionality to react-input-mask but with more built-in format options.
react-maskedinput is another library for creating input masks in React applications. It offers similar functionality to react-input-mask but is simpler and more lightweight. It is suitable for basic input masking needs without the additional features provided by react-input-mask.
npm install --save @types/react-input-mask
This package contains type definitions for react-input-mask (https://github.com/sanniassin/react-input-mask).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/react-input-mask.
import * as React from "react";
export interface Selection {
start: number;
end: number;
}
export interface InputState {
value: string;
selection: Selection | null;
}
export interface BeforeMaskedStateChangeStates {
previousState: InputState;
currentState: InputState;
nextState: InputState;
}
export type Props = Omit<React.InputHTMLAttributes<HTMLInputElement>, "children"> & {
/**
* Mask string. Format characters are:
* * `9`: `0-9`
* * `a`: `A-Z, a-z`
* * `\*`: `A-Z, a-z, 0-9`
*
* Any character can be escaped with backslash, which usually will appear as double backslash in JS strings.
* For example, German phone mask with unremoveable prefix +49 will look like `mask="+4\\9 99 999 99"` or `mask={"+4\\\\9 99 999 99"}`
*/
mask: string | Array<(string | RegExp)>;
/**
* Character to cover unfilled editable parts of mask. Default character is "_". If set to null, unfilled parts will be empty, like in ordinary input.
*/
maskChar?: string | null | undefined;
maskPlaceholder?: string | null | undefined;
/**
* Show mask even in empty input without focus.
*/
alwaysShowMask?: boolean | undefined;
/**
* Use inputRef instead of ref if you need input node to manage focus, selection, etc.
*/
inputRef?: React.Ref<HTMLInputElement> | undefined;
/**
* In case you need to implement more complex masking behavior, you can provide
* beforeMaskedStateChange function to change masked value and cursor position
* before it will be applied to the input.
*
* * previousState: Input state before change. Only defined on change event.
* * currentState: Current raw input state. Not defined during component render.
* * nextState: Input state with applied mask. Contains value and selection fields.
*/
beforeMaskedStateChange?(states: BeforeMaskedStateChangeStates): InputState;
children?: (inputProps: any) => React.ReactNode;
};
export class ReactInputMask extends React.Component<Props> {
}
export default ReactInputMask;
These definitions were written by Alexandre Paré, Dima Danylyuk, and Lucas Rêgo.
FAQs
TypeScript definitions for react-input-mask
The npm package @types/react-input-mask receives a total of 244,288 weekly downloads. As such, @types/react-input-mask popularity was classified as popular.
We found that @types/react-input-mask demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.