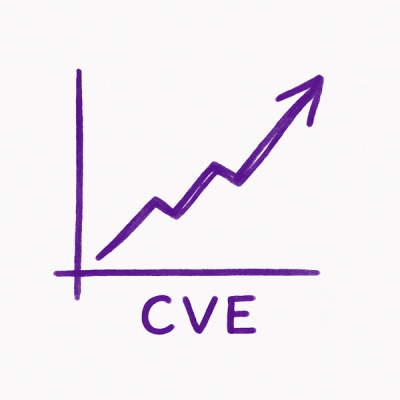
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
@types/react-select
Advanced tools
Stub TypeScript definitions entry for react-select, which provides its own types definitions
@types/react-select provides TypeScript type definitions for the react-select library, which is a flexible and customizable select input control for React applications.
Basic Select
This feature allows you to create a basic select input with predefined options.
import Select from 'react-select';
const options = [
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
];
const MyComponent = () => (
<Select options={options} />
);
Async Select
This feature allows you to create a select input that loads options asynchronously, useful for fetching data from an API.
import AsyncSelect from 'react-select/async';
const loadOptions = (inputValue: string, callback: (options: any[]) => void) => {
setTimeout(() => {
callback([
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
]);
}, 1000);
};
const MyComponent = () => (
<AsyncSelect loadOptions={loadOptions} />
);
Creatable Select
This feature allows users to create new options in addition to selecting from predefined ones.
import CreatableSelect from 'react-select/creatable';
const options = [
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
];
const MyComponent = () => (
<CreatableSelect options={options} />
);
@types/react-autosuggest provides TypeScript type definitions for the react-autosuggest library, which is used for creating autosuggest input fields. It is similar to react-select in that it provides a way to select from a list of suggestions, but it focuses more on autosuggestions rather than dropdown selections.
@types/react-virtualized-select provides TypeScript type definitions for the react-virtualized-select library, which combines react-select with react-virtualized for rendering large lists efficiently. It is useful when dealing with a large number of options that need to be rendered efficiently.
This is a stub types definition for @types/react-select (undefined).
react-select provides its own type definitions, so you don't need @types/react-select installed!
FAQs
Stub TypeScript definitions entry for react-select, which provides its own types definitions
The npm package @types/react-select receives a total of 564,220 weekly downloads. As such, @types/react-select popularity was classified as popular.
We found that @types/react-select demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.