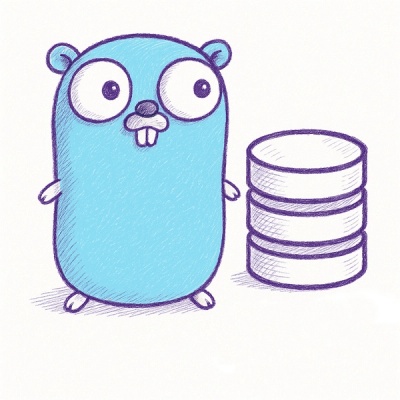
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
@types/react-slick
Advanced tools
TypeScript definitions for react-slick
@types/react-slick provides TypeScript definitions for the react-slick package, which is a popular carousel component for React applications.
Basic Carousel
This code demonstrates a basic carousel setup using react-slick with TypeScript. The settings object configures the carousel to show dots, loop infinitely, and have a transition speed of 500ms.
import Slider from 'react-slick';
const settings = {
dots: true,
infinite: true,
speed: 500,
slidesToShow: 1,
slidesToScroll: 1
};
const BasicCarousel = () => (
<Slider {...settings}>
<div><h3>Slide 1</h3></div>
<div><h3>Slide 2</h3></div>
<div><h3>Slide 3</h3></div>
</Slider>
);
Responsive Carousel
This code demonstrates a responsive carousel setup using react-slick with TypeScript. The settings object includes a responsive array that adjusts the number of slides shown based on the screen width.
import Slider from 'react-slick';
const settings = {
dots: true,
infinite: true,
speed: 500,
slidesToShow: 1,
slidesToScroll: 1,
responsive: [
{
breakpoint: 1024,
settings: {
slidesToShow: 3,
slidesToScroll: 3,
infinite: true,
dots: true
}
},
{
breakpoint: 600,
settings: {
slidesToShow: 2,
slidesToScroll: 2
}
},
{
breakpoint: 480,
settings: {
slidesToShow: 1,
slidesToScroll: 1
}
}
]
};
const ResponsiveCarousel = () => (
<Slider {...settings}>
<div><h3>Slide 1</h3></div>
<div><h3>Slide 2</h3></div>
<div><h3>Slide 3</h3></div>
</Slider>
);
Custom Arrows
This code demonstrates how to use custom arrows in a react-slick carousel with TypeScript. The NextArrow and PrevArrow components are used to customize the appearance and behavior of the navigation arrows.
import Slider from 'react-slick';
const NextArrow = (props) => {
const { className, style, onClick } = props;
return (
<div
className={className}
style={{ ...style, display: 'block', background: 'red' }}
onClick={onClick}
/>
);
};
const PrevArrow = (props) => {
const { className, style, onClick } = props;
return (
<div
className={className}
style={{ ...style, display: 'block', background: 'green' }}
onClick={onClick}
/>
);
};
const settings = {
dots: true,
infinite: true,
speed: 500,
slidesToShow: 1,
slidesToScroll: 1,
nextArrow: <NextArrow />,
prevArrow: <PrevArrow />
};
const CustomArrowsCarousel = () => (
<Slider {...settings}>
<div><h3>Slide 1</h3></div>
<div><h3>Slide 2</h3></div>
<div><h3>Slide 3</h3></div>
</Slider>
);
react-responsive-carousel is another popular carousel component for React. It offers similar functionality to react-slick but focuses more on responsiveness and ease of use. It also provides TypeScript definitions out of the box.
swiper is a modern mobile touch slider with hardware-accelerated transitions. It is highly customizable and supports a wide range of features, including parallax effects, lazy loading, and more. Swiper also provides TypeScript definitions and is widely used in both web and mobile applications.
pure-react-carousel is a highly customizable and lightweight carousel component for React. It provides a more modular approach compared to react-slick, allowing developers to build their own navigation and pagination components. It also includes TypeScript definitions.
npm install --save @types/react-slick
This package contains type definitions for react-slick (https://github.com/akiran/react-slick).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/react-slick.
These definitions were written by Giedrius Grabauskas, Andrew Makarov, and Shannor Trotty.
FAQs
TypeScript definitions for react-slick
The npm package @types/react-slick receives a total of 627,552 weekly downloads. As such, @types/react-slick popularity was classified as popular.
We found that @types/react-slick demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.