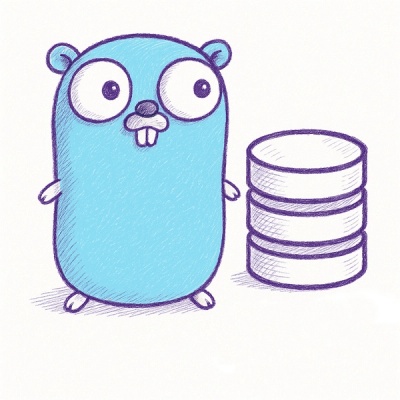
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
@types/webgl2
Advanced tools
TypeScript definitions for webgl2
npm install --save @types/webgl2
This package contains type definitions for webgl2 (https://www.khronos.org/registry/webgl/specs/latest/2.0/).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/webgl2.
interface HTMLCanvasElement extends HTMLElement {
getContext(
contextId: "webgl2" | "experimental-webgl2",
contextAttributes?: WebGLContextAttributes,
): WebGL2RenderingContext | null;
}
interface ImageBitmap {
readonly width: number;
readonly height: number;
close(): void;
}
interface WebGLQuery {
}
declare var WebGLQuery: {
prototype: WebGLQuery;
new(): WebGLQuery;
};
interface WebGLSampler {
}
declare var WebGLSampler: {
prototype: WebGLSampler;
new(): WebGLSampler;
};
interface WebGLSync {
}
declare var WebGLSync: {
prototype: WebGLSync;
new(): WebGLSync;
};
interface WebGLTransformFeedback {
}
declare var WebGLTransformFeedback: {
prototype: WebGLTransformFeedback;
new(): WebGLTransformFeedback;
};
interface WebGLVertexArrayObject {
}
declare var WebGLVertexArrayObject: {
prototype: WebGLVertexArrayObject;
new(): WebGLVertexArrayObject;
};
These definitions were written by Nico Kemnitz, and Adrian Blumer.
FAQs
TypeScript definitions for webgl2
The npm package @types/webgl2 receives a total of 122,412 weekly downloads. As such, @types/webgl2 popularity was classified as popular.
We found that @types/webgl2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.