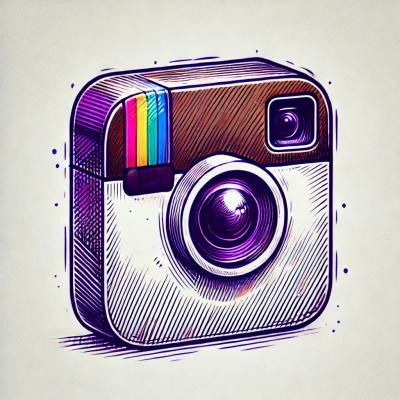
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
@ucast/mongo2js
Advanced tools
@ucast/mongo2js is a utility library that converts MongoDB query objects into JavaScript functions. This can be particularly useful for filtering data in JavaScript applications using the same query syntax as MongoDB.
Convert MongoDB Query to JavaScript Function
This feature allows you to convert a MongoDB query object into a JavaScript function that can be used to filter arrays of objects. In this example, the query filters out objects where the age is greater than 18.
const { compile } = require('@ucast/mongo2js');
const query = { age: { $gt: 18 } };
const filterFunction = compile(query);
const data = [
{ name: 'Alice', age: 17 },
{ name: 'Bob', age: 20 },
{ name: 'Charlie', age: 22 }
];
const filteredData = data.filter(filterFunction);
console.log(filteredData); // Output: [{ name: 'Bob', age: 20 }, { name: 'Charlie', age: 22 }]
Support for Complex Queries
This feature supports complex MongoDB queries, including logical operators like $and. In this example, the query filters out objects where the age is greater than 18 and the name contains the letter 'B'.
const { compile } = require('@ucast/mongo2js');
const query = { $and: [ { age: { $gt: 18 } }, { name: { $regex: 'B' } } ] };
const filterFunction = compile(query);
const data = [
{ name: 'Alice', age: 17 },
{ name: 'Bob', age: 20 },
{ name: 'Charlie', age: 22 }
];
const filteredData = data.filter(filterFunction);
console.log(filteredData); // Output: [{ name: 'Bob', age: 20 }]
mongo-parse is a library that parses MongoDB queries and provides a way to manipulate and analyze them. Unlike @ucast/mongo2js, which converts queries into JavaScript functions, mongo-parse is more focused on query analysis and manipulation.
json-query is a library for querying JSON objects using a simple query language. While it provides a way to filter JSON data, it does not use MongoDB query syntax and is not specifically designed to convert MongoDB queries into JavaScript functions like @ucast/mongo2js.
This package is a part of ucast ecosystem. It combines @ucast/mongo and @ucast/js into a package that allows to evaluate MongoDB query conditions in JavaScript runtime.
npm i @ucast/mongo2js
# or
yarn add @ucast/mongo2js
# or
pnpm add @ucast/mongo2js
To check that POJO can be matched by Mongo Query:
import { guard } from '@ucast/mongo2js';
const test = guard({
lastName: 'Doe',
age: { $gt: 18 }
});
console.log(test({
firstName: 'John',
lastName: 'Doe',
age: 19
})); // true
You can also get access to parsed Mongo Query AST:
console.log(test.ast); /*
{
operator: 'and',
value: [
{ operator: 'eq', field: 'lastName', value: 'Doe' },
{ operator: 'gt', field: 'age', value: 18 }
]
}
*/
For cases, when you need to test primitive elements, you can use squire
function:
import { squire } from '@ucast/mongo2js';
const test = squire({
$lt: 10,
$gt: 18
});
test(11) // true
test(9) // false
In order to implement a custom operator, you need to create a custom parsing instruction for MongoQueryParser
and custom JsInterpreter
to interpret this operator in JavaScript runtime.
This package re-exports all symbols from @ucast/mongo
and @ucast/js
, so you don't need to install them separately. For example, to add support for json-schema operator:
import {
createFactory,
DocumentCondition,
ParsingInstruction,
JsInterpreter,
} from '@ucast/mongo2js';
import Ajv from 'ajv';
type JSONSchema = object;
const ajv = new Ajv();
const $jsonSchema: ParsingInstruction<JSONSchema> = {
type: 'document',
validate(instruction, value) {
if (!value || typeof value !== 'object') {
throw new Error(`"${instruction.name}" expects to receive an object`)
}
},
parse(instruction, schema) {
return new DocumentCondition(instruction.name, ajv.compile(schema));
}
};
const jsonSchema: JsInterpreter<DocumentCondition<Ajv.ValidateFunction>> = (
condition,
object
) => condition.value(object) as boolean;
const customGuard = createFactory({
$jsonSchema,
}, {
jsonSchema
});
const test = customGuard({
$jsonSchema: {
type: 'object',
properties: {
firstName: { type: 'string' },
lastName: { type: 'string' },
},
required: ['firstName', 'lastName'],
}
});
console.log(test({ firstName: 'John' })); // false, `lastName` is not defined
To create a custom operator which tests primitives (as squire
does), use the
forPrimitives
option:
const customSquire = createFactory({
$custom: {
type: 'field',
}
}, {
custom: (condition, value) => value === (condition.value ? 'on' : 'off')
}, {
forPrimitives: true
});
const test = customGuard({ $custom: true });
console.log(test('on')) // true
This package is written in TypeScript and supports type inference for MongoQuery:
import { guard } from '@ucast/mongo2js';
interface Person {
firstName: string
lastName: string
age: number
}
const test = guard<Person>({ lastName: 'Doe' });
You can also use dot notation to set conditions on deeply nested fields:
import { guard } from '@ucast/mongo2js';
interface Person {
firstName: string
lastName: string
age: number
address: {
city: string
country: string
}
}
type ExtendedPerson = Person & {
'address.city': Person['address']['city']
}
const test = guard<ExtendedPerson>({ lastName: 'Doe' });
Want to file a bug, contribute some code, or improve documentation? Excellent! Read up on guidelines for contributing
FAQs
git@github.com:stalniy/ucast.git
We found that @ucast/mongo2js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.