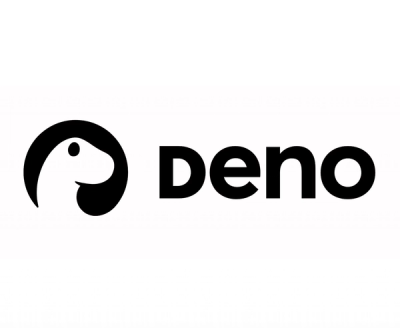
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
@userback/react
Advanced tools
The official NPM module for embedding the Userback.io widget into your React application.
npm i @userback/react
or yarn add @userback/react
The easiest way to get started with Userback is to simply use the Provider near the top of you React Tree like so:
import { UserbackProvider } from '@userback/react';
ReactDOM.createRoot(document.getElementById('root')).render(
<UserbackProvider token="**USERBACK_TOKEN**">
<App />
</UserbackProvider>
);
With a valid token provided, the Userback Widget will automatically load and be ready to use!
Along with token
, you can also provide an options
prop:
import { UserbackProvider } from '@userback/react';
// identify your logged-in users (optional)
const user_data = {
id: "123456", // example data
info: {
name: "someone", // example data
email: "someone@example.com" // example data
}
}
ReactDOM.createRoot(document.getElementById('root')).render(
<UserbackProvider token="**USERBACK_TOKEN**" options={{user_data: user_data}}>
<App />
</UserbackProvider>
);
You can access Userback hooks in child components of the <UserbackProvider>
with useUserback()
:
import { useUserback } from '@userback/react'
function App() {
const { open, hide, show } = useUserback()
return (
<div id="app">
<button type="button" onClick={() => open('general', 'screenshot')}>Take a screenshot</button>
<button type="button" onClick={show}>Show Userback</button>
<button type="button" onClick={hide}>Hide me :(</button>
</div>
)
}
export default App
If you are using class based components and need an alternative to hooks, you can use the withUserback
Higher Order Component:
import { withUserback } from '@userback/react'
class App extends React.Component {
render() {
return (
<div id="app">
<button type="button" onClick={() => this.props.userback.open('general', 'screenshot')}>Take a screenshot</button>
<button type="button" onClick={() => this.props.userback.hide()}>Hide me :(</button>
</div>
)
}
}
export default withUserback(App)
For more information about available configuration settings and and functions available, see our Javascript API
FAQs
Userback.io widget for React
The npm package @userback/react receives a total of 3,672 weekly downloads. As such, @userback/react popularity was classified as popular.
We found that @userback/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.