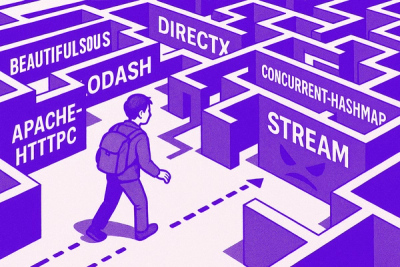
Research
NPM targeted by malware campaign mimicking familiar library names
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
@vercel/kv
Advanced tools
A client that works with Vercel KV.
npm install @vercel/kv
import { kv } from '@vercel/kv';
// string
await kv.set('key', 'value');
let data = await kv.get('key');
console.log(data); // 'value'
await kv.set('key2', 'value2', { ex: 1 });
// sorted set
await kv.zadd(
'scores',
{ score: 1, member: 'team1' },
{ score: 2, member: 'team2' },
);
data = await kv.zrange('scores', 0, 0);
console.log(data); // [ 'team1' ]
// list
await kv.lpush('elements', 'magnesium');
data = await kv.lrange('elements', 0, 100);
console.log(data); // [ 'magnesium' ]
// hash
await kv.hset('people', { name: 'joe' });
data = await kv.hget('people', 'name');
console.log(data); // 'joe'
// sets
await kv.sadd('animals', 'cat');
data = await kv.spop('animals', 1);
console.log(data); // [ 'cat' ]
// scan for keys
for await (const key of kv.scanIterator()) {
console.log(key);
}
By default @vercel/kv
reads the KV_REST_API_URL
and KV_REST_API_TOKEN
environment variables. Use the following function in case you need to define custom values
import { createClient } from '@vercel/kv';
const kv = createClient({
url: 'https://<hostname>.redis.vercel-storage.com',
token: '<token>',
});
await kv.set('key', 'value');
The default kv
client automatically deserializes values returned from the database via JSON.parse
. If this behaviour is undesired, create a custom KV client via the createClient
method with automaticDeserialization: false
. All data will be returned as strings.
import { kv, createClient } from '@vercel/kv';
const customKvClient = createClient({
url: process.env.KV_REST_API_URL,
token: process.env.KV_REST_API_TOKEN,
automaticDeserialization: false,
});
await customKvClient.set('object', { hello: 'world' });
console.log(await kv.get('object')); // { hello: 'world' }
console.log(await customKvClient.get('object')); // '{"hello":"world"}'
See the documentation for details.
@vercel/kv
reads database credentials from the environment variables on process.env
. In general, process.env
is automatically populated from your .env
file during development, which is created when you run vc env pull
. However, Vite does not expose the .env
variables on process.env.
You can fix this in one of following two ways:
process.env
yourself using something like dotenv-expand
:pnpm install --save-dev dotenv dotenv-expand
// vite.config.js
import dotenvExpand from 'dotenv-expand';
import { loadEnv, defineConfig } from 'vite';
export default defineConfig(({ mode }) => {
// This check is important!
if (mode === 'development') {
const env = loadEnv(mode, process.cwd(), '');
dotenvExpand.expand({ parsed: env });
}
return {
...
};
});
$env/static/private
:import { createClient } from '@vercel/kv';
+ import { KV_REST_API_URL, KV_REST_API_TOKEN } from '$env/static/private';
const kv = createClient({
- url: 'https://<hostname>.redis.vercel-storage.com',
- token: '<token>',
+ url: KV_REST_API_URL,
+ token: KV_REST_API_TOKEN,
});
await kv.set('key', 'value');
@vercel/kv
package support Redis Streams?No, the @vercel/kv
package does not support Redis Streams. To use Redis Streams with Vercel KV, you must connect directly to the database server via packacges like io-redis
or node-redis
.
import { createClient } from 'redis';
const client = createClient({
url: process.env.KV_URL,
});
await client.connect();
await client.xRead({ key: 'mystream', id: '0' }, { COUNT: 2 });
FAQs
Durable Redis
The npm package @vercel/kv receives a total of 149,347 weekly downloads. As such, @vercel/kv popularity was classified as popular.
We found that @vercel/kv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket uncovered npm malware campaign mimicking popular Node.js libraries and packages from other ecosystems; packages steal data and execute remote code.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.