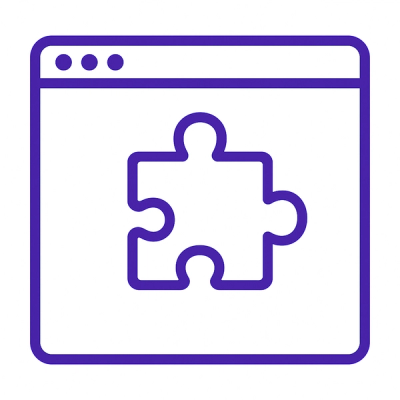
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
@web3-name-sdk/core
Advanced tools
Web3 Name SDK is an universal web3 identity solution for domain name resolution. Developers can easily get access to .eth, .bnb, .arb, .lens, .crypto names and more.
Developers can resolve web3 domain name or reverse resolve address with web3 name SDK with zero configuration.
npm install @web3-name-sdk/core viem@^2.23.12
If you are using next.js
, please add the following configuration in your next.config.js
in order to transpile commonjs dependencies:
const nextConfig = {
transpilePackages: ['@web3-name-sdk/core'],
}
import { createWeb3Name } from '@web3-name-sdk/core'
const web3Name = createWeb3Name()
You can get address from domain name with a single request:
const address = await web3name.getAddress('spaceid.bnb')
// expect: '0xb5932a6b7d50a966aec6c74c97385412fb497540'
const address = await web3name.getAddress('bts_official.lens')
// expect: '0xd80efa68b50d21e548b9cdb092ebc6e5bca113e7'
const address = await web3name.getAddress('beresnev.crypto')
// expect: '0x6ec0deed30605bcd19342f3c30201db263291589'
const address = await web3name.getAddress('registry.gno')
// expect: '0x2886D6792503e04b19640C1f1430d23219AF177F'
Domain resolution for other chains can be provided by adding coinType
param to getAddress()
.
import { convertEVMChainIdToCoinType } from '@ensdomains/address-encoder'
const address = await web3name.getAddress('gnome.gno', { coinType: convertEVMChainIdToCoinType(1) })
// expect: 0x4348d45967552d0176d465170b7375ed22dc627b
There are optional parameters in the method to select your target chain or TLD (top-level domain).
By providing chain IDs, you can resolve addresses on selected chains and get an available domain name from all TLDs deployed on these chains.
// Resolve an address from Gnosis Chiado
const name = await web3name.getDomainName({
address: '0x2886D6792503e04b19640C1f1430d23219AF177F',
queryChainIdList: [10200],
})
// expect: lydia.gno
By providing TLDs, address can be resolved from the selected TLDs and get an available TLD primary name.
// Resolve an address from .gno TLD
const name = await web3name.getDomainName({
address: '0x2886D6792503e04b19640C1f1430d23219AF177F',
queryTldList: ['gno'],
})
// expect: genome.gno
You need to provide your target chain client and then provide optional parameters in the method. The method returns an list containing the address and its corresponding domain.
const res = await web3Name.batchGetDomainNameByTld({
addressList: ['0x2886d6792503e04b19640c1f1430d23219af177f', '0xb5932a6b7d50a966aec6c74c97385412fb497540'],
queryTld: 'bnb',
})
// expect: [{address: '0x2886d6792503e04b19640c1f1430d23219af177f', domain: 'goodh.bnb'}, {address: '0xb5932a6b7d50a966aec6c74c97385412fb497540', domain: 'spaceid.bnb'}]
const res = await web3Name.batchGetDomainNameByChainId({
addressList: ['0x77777775b611f0f3d90ccb69ef425a62b35afa7c', '0x3506fbe85e19bf025b228ec58f143ba342c3c608'],
queryChainId: 42_161,
})
// expect: [{address: '0x77777775b611f0f3d90ccb69ef425a62b35afa7c', domain: 'megantrhopus.arb'}, {address: '0x3506fbe85e19bf025b228ec58f143ba342c3c608', domain: 'idgue.arb'}]
Domain text records can be fetched by providing domain name and the key. For example, the avatar record of spaceid.bnb
is returned from this method given key name avatar
:
const record = await sid.getDomainRecord({ name: 'spaceid.bnb', key: 'avatar' })
Domain metadata can be fetched by SDK directly.
// requesting
const metadata = await web3Name.getMetadata({ name: 'public.gno' })
As an all-in-one domain name SDK, non-EVM web3 domain name services are also included. Now we support SNS (Solana Name Service, .sol), Sei Name Service (.sei) and Injective Name Service (.inj).
Install additional corresponding dependencies for Solana environment:
npm install @solana/web3 @bonfida/spl-name-service@^3.0.10
Create client and query domains:
import { createSolName } from '@web3-name-sdk/core/solName'
// recommended to provide a private Solana RPC, as the official one is prone to restrictions.
const web3Name = createSolName({ rpcUrl: 'http://....' })
const domain = await web3Name.getDomainName({
address: 'HKKp49qGWXd639QsuH7JiLijfVW5UtCVY4s1n2HANwEA',
}) // expect: bonfida
Install additional corresponding dependencies for Sei environment:
npm install @sei-js/core@^3.1.0 @siddomains/sei-sidjs@^0.0.4
Create client and query domains:
import { createSeiName } from '@web3-name-sdk/core/seiName'
const web3Name = createSeiName()
const domain = await web3Name.getDomainName({
address: 'sei1tmew60aj394kdfff0t54lfaelu3p8j8lz93pmf',
}) // expect: allen.sei
Install additional corresponding dependencies for Injective environment:
npm install @siddomains/injective-sidjs@0.0.2-beta @injectivelabs/networks @injectivelabs/ts-types
Create client and query domains:
import { createInjName } from '@web3-name-sdk/core/injName'
const web3Name = createInjName()
const domain = await web3Name.getDomainName({
address: 'inj10zvhv2a2mam8w7lhy96zgg2v8d800xcs7hf2tf',
}) // expect: testtest.inj
When using INJ or SEI name services with Next.js, you'll need additional webpack configuration to handle dynamic imports and polyfills. Due to the complexity of these dependencies, extra configuration is required.
Required dependencies:
npm install crypto-browserify stream-browserify buffer babel-loader @babel/preset-env @babel/plugin-transform-private-methods @babel/plugin-transform-private-property-in-object @babel/plugin-transform-runtime
View the complete Next.js configuration example: next.config.example.js
Web3 Name SDK also supports PaymentID domains (using @tld format). These domains are resolved through the Gravity blockchain.
const address = await web3name.getAddress('jerry@binance')
const ethereumAddress = await web3name.getAddress('jerry@binance', { chainId: 1 })
// Returns the Ethereum address (chain ID 1) associated with the PaymentID domain
When resolving PaymentID domains, you can specify a chainId parameter to get addresses for specific blockchains:
Type | ID |
---|---|
Bitcoin | 0 |
Evm | 1 |
Solana | 2 |
Tron | 3 |
Aptos | 4 |
Sui | 5 |
// Example: Get Bitcoin address from PaymentID domain
const bitcoinAddress = await web3name.getAddress('username@paymentid', { chainId: 0 })
// Example: Get Solana address from PaymentID domain
const solanaAddress = await web3name.getAddress('username@paymentid', { chainId: 2 })
We are using popular public RPC services by default to make it easier to use. But in some cases developers may prefer to use arbitrary RPC, so we provide optional parameter rpcUrl
for each function that allows developers to use their own RPC to make requests.
For example, you can put custom rpcUrl as a parameter in getAddress
function.
// Use custom RPC url (https://arb1.arbitrum.io/rpc)
const address = await web3name.getAddress('registry.arb', {
rpcUrl: 'https://arb1.arbitrum.io/rpc',
})
// expect: '0x8d27d6235d9d8EFc9Eef0505e745dB67D5cD2918'
For other functions, it's also possible to have a custom rpcUrl
in the request.
// Use custom RPC url (https://arb1.arbitrum.io/rpc)
const address = await web3name.getMetaData('registry.arb', {
rpcUrl: 'https://arb1.arbitrum.io/rpc',
})
// expect: '0x8d27d6235d9d8EFc9Eef0505e745dB67D5cD2918'
The SDK provides timeout control for all network requests. You can set timeouts in two ways:
Set a global timeout when creating the client:
// Set a 5-second timeout for all requests
const web3Name = createWeb3Name({ timeout: 5000 })
const solName = createSolName({ timeout: 5000 })
const seiName = createSeiName({ timeout: 5000 })
const injName = createInjName({ timeout: 5000 })
Override the global timeout for specific requests:
// Set a 10-second timeout for this specific request
const address = await web3name.getAddress('vitalik.eth', { timeout: 10000 })
// Timeout can be combined with other options
const address = await web3name.getAddress('registry.arb', {
rpcUrl: 'https://arb1.arbitrum.io/rpc',
timeout: 5000,
})
// Works with all methods
const name = await web3name.getDomainName({
address: '0x2886D6792503e04b19640C1f1430d23219AF177F',
queryChainIdList: [10200],
timeout: 5000,
})
const metadata = await web3name.getMetadata({
name: 'spaceid.bnb',
timeout: 5000,
})
If a request exceeds the timeout duration, it will throw an error with a message indicating the timeout. The timeout applies to all network operations, including name resolution, reverse lookups, and metadata fetches.
FAQs
One stop Web3 domain name resolution
The npm package @web3-name-sdk/core receives a total of 1,123 weekly downloads. As such, @web3-name-sdk/core popularity was classified as popular.
We found that @web3-name-sdk/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.