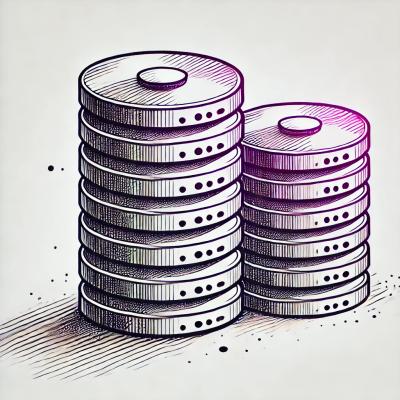
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@webassemblyjs/ast
Advanced tools
The @webassemblyjs/ast package is a toolchain for WebAssembly manipulation which allows you to parse, validate, and generate WebAssembly code. It provides an Abstract Syntax Tree (AST) representation of WebAssembly modules, enabling programmatic analysis and transformation of Wasm code.
Parsing WebAssembly binary
This feature allows you to parse a WebAssembly binary into an AST. The code sample demonstrates how to use the wasm-parser submodule to decode a binary representation of a WebAssembly module.
"use strict";\nconst { decode } = require('@webassemblyjs/wasm-parser');\nconst binary = new Uint8Array([0x00, 0x61, 0x73, 0x6d, ...]);\nconst ast = decode(binary);\nconsole.log(ast);
Generating WebAssembly binary
This feature enables you to generate a WebAssembly binary from an AST. The code sample shows how to create an empty WebAssembly module using the ast submodule and then encode it into a binary with wasm-gen.
"use strict";\nconst { encode } = require('@webassemblyjs/wasm-gen');\nconst t = require('@webassemblyjs/ast');\nconst module = t.module([], []);\nconst binary = encode(module);\nconsole.log(new Uint8Array(binary));
Traversing and modifying the AST
This feature is for traversing the AST and applying modifications. The code sample illustrates how to traverse an existing AST and log each ModuleImport node encountered.
"use strict";\nconst { traverse } = require('@webassemblyjs/ast');\nconst ast = ...; // Assume this is an existing AST\ntraverse(ast, {\n ModuleImport(path) {\n console.log(path.node);\n }\n});
The wast-parser package is similar to @webassemblyjs/ast in that it can parse WebAssembly text format (WAT) into an AST. However, it focuses on the text format rather than the binary format and does not provide binary generation capabilities.
Binaryen is a compiler and toolchain infrastructure library for WebAssembly, written in C++. It has bindings for JavaScript and provides similar functionalities such as parsing, optimization, and generation of WebAssembly code. Compared to @webassemblyjs/ast, Binaryen offers a broader range of optimization tools and is implemented in a different programming language.
WABT (The WebAssembly Binary Toolkit) is a suite of tools for WebAssembly, including a decoder, an interpreter, and a binary format validator. It is similar to @webassemblyjs/ast in that it allows manipulation of WebAssembly binaries but is a standalone toolkit rather than an npm package.
AST utils for webassemblyjs
yarn add @webassemblyjs/ast
import { traverse } from "@webassemblyjs/ast";
traverse(ast, {
Module(path) {
console.log(path.node);
}
});
import { signatures } from "@webassemblyjs/ast";
console.log(signatures);
findParent: NodeLocator
replaceWith: Node => void
remove: () => void
insertBefore: Node => void
insertAfter: Node => void
stop: () => void
module(id, fields, metadata)
moduleMetadata(sections, functionNames, localNames)
moduleNameMetadata(value)
functionNameMetadata(value, index)
localNameMetadata(value, localIndex, functionIndex)
binaryModule(id, blob)
quoteModule(id, string)
sectionMetadata(section, startOffset, size, vectorOfSize)
loopInstruction(label, resulttype, instr)
instruction(id, args, namedArgs)
objectInstruction(id, object, args, namedArgs)
ifInstruction(testLabel, test, result, consequent, alternate)
stringLiteral(value)
numberLiteralFromRaw(value, raw)
longNumberLiteral(value, raw)
floatLiteral(value, nan, inf, raw)
elem(table, offset, funcs)
indexInFuncSection(index)
valtypeLiteral(name)
typeInstruction(id, functype)
start(index)
globalType(valtype, mutability)
leadingComment(value)
blockComment(value)
data(memoryIndex, offset, init)
global(globalType, init, name)
table(elementType, limits, name, elements)
memory(limits, id)
funcImportDescr(id, signature)
moduleImport(module, name, descr)
moduleExportDescr(exportType, id)
moduleExport(name, descr)
limit(min, max)
signature(params, results)
program(body)
identifier(value, raw)
blockInstruction(label, instr, result)
callInstruction(index, instrArgs)
callIndirectInstruction(signature, intrs)
byteArray(values)
func(name, signature, body, isExternal, metadata)
isModule
isModuleMetadata
isModuleNameMetadata
isFunctionNameMetadata
isLocalNameMetadata
isBinaryModule
isQuoteModule
isSectionMetadata
isLoopInstruction
isInstruction
isObjectInstruction
isIfInstruction
isStringLiteral
isNumberLiteral
isLongNumberLiteral
isFloatLiteral
isElem
isIndexInFuncSection
isValtypeLiteral
isTypeInstruction
isStart
isGlobalType
isLeadingComment
isBlockComment
isData
isGlobal
isTable
isMemory
isFuncImportDescr
isModuleImport
isModuleExportDescr
isModuleExport
isLimit
isSignature
isProgram
isIdentifier
isBlockInstruction
isCallInstruction
isCallIndirectInstruction
isByteArray
isFunc
assertModule
assertModuleMetadata
assertModuleNameMetadata
assertFunctionNameMetadata
assertLocalNameMetadata
assertBinaryModule
assertQuoteModule
assertSectionMetadata
assertLoopInstruction
assertInstruction
assertObjectInstruction
assertIfInstruction
assertStringLiteral
assertNumberLiteral
assertLongNumberLiteral
assertFloatLiteral
assertElem
assertIndexInFuncSection
assertValtypeLiteral
assertTypeInstruction
assertStart
assertGlobalType
assertLeadingComment
assertBlockComment
assertData
assertGlobal
assertTable
assertMemory
assertFuncImportDescr
assertModuleImport
assertModuleExportDescr
assertModuleExport
assertLimit
assertSignature
assertProgram
assertIdentifier
assertBlockInstruction
assertCallInstruction
assertCallIndirectInstruction
assertByteArray
assertFunc
FAQs
AST utils for webassemblyjs
The npm package @webassemblyjs/ast receives a total of 0 weekly downloads. As such, @webassemblyjs/ast popularity was classified as not popular.
We found that @webassemblyjs/ast demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.