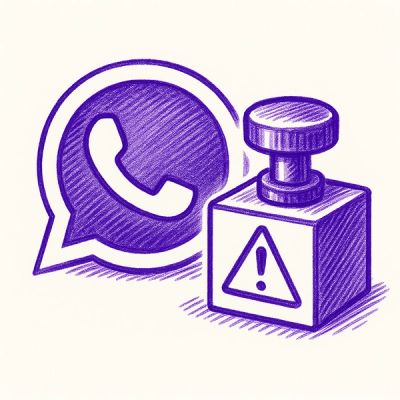
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
@wordpress/components
Advanced tools
@wordpress/components is a library of reusable UI components for building WordPress plugins and themes. It provides a wide range of components that adhere to the WordPress design language, making it easier to create consistent and accessible user interfaces.
Button
The Button component is used to create various types of buttons, such as primary, secondary, and tertiary buttons. It supports different states and styles.
import { Button } from '@wordpress/components';
const MyButton = () => (
<Button isPrimary onClick={() => alert('Button clicked!')}>Click Me</Button>
);
Panel
The Panel component is used to create collapsible panels, which can contain various types of content. It is useful for organizing settings and options in a structured way.
import { Panel, PanelBody, PanelRow } from '@wordpress/components';
const MyPanel = () => (
<Panel>
<PanelBody title="My Panel" initialOpen={true}>
<PanelRow>
<p>Panel content goes here.</p>
</PanelRow>
</PanelBody>
</Panel>
);
TextControl
The TextControl component is used to create text input fields. It supports labels, placeholders, and change handlers, making it easy to capture user input.
import { TextControl } from '@wordpress/components';
const MyTextControl = () => (
<TextControl
label="Name"
value={name}
onChange={(value) => setName(value)}
/>
);
CheckboxControl
The CheckboxControl component is used to create checkbox input fields. It supports labels and change handlers, making it easy to capture boolean input.
import { CheckboxControl } from '@wordpress/components';
const MyCheckboxControl = () => (
<CheckboxControl
label="Accept Terms"
checked={isChecked}
onChange={(isChecked) => setIsChecked(isChecked)}
/>
);
ColorPicker
The ColorPicker component is used to create a color picker input. It allows users to select colors and provides the selected color value.
import { ColorPicker } from '@wordpress/components';
const MyColorPicker = () => (
<ColorPicker
color={color}
onChangeComplete={(value) => setColor(value.hex)}
/>
);
React-Bootstrap is a popular library that provides Bootstrap components as React components. It offers a wide range of UI components similar to @wordpress/components but follows the Bootstrap design language.
Material-UI is a comprehensive library of React components that implement Google's Material Design. It offers a wide range of UI components and utilities, similar to @wordpress/components, but follows the Material Design guidelines.
Ant Design (antd) is a React UI library that provides a set of high-quality components and design guidelines. It offers a wide range of components similar to @wordpress/components but follows the Ant Design specifications.
Semantic UI React is the official React integration for Semantic UI. It provides a wide range of UI components similar to @wordpress/components but follows the Semantic UI design principles.
This package includes a library of generic WordPress components to be used for creating common UI elements shared between screens and features of the WordPress dashboard.
npm install @wordpress/components --save
This package assumes that your code will run in an ES2015+ environment. If you're using an environment that has limited or no support for such language features and APIs, you should include the polyfill shipped in @wordpress/babel-preset-default
in your code.
Within Gutenberg, these components can be accessed by importing from the components
root directory:
/**
* WordPress dependencies
*/
import { Button } from '@wordpress/components';
export default function MyButton() {
return <Button>Click Me!</Button>;
}
Many components include CSS to add styles, which you will need to load in order for them to appear correctly. Within WordPress, add the wp-components
stylesheet as a dependency of your plugin's stylesheet. See wp_enqueue_style documentation for how to specify dependencies.
In non-WordPress projects, link to the build-style/style.css
file directly, it is located at node_modules/@wordpress/components/build-style/style.css
.
By default, the Popover
component will render within an extra element appended to the body of the document.
If you want to precisely control where the popovers render, you will need to use the Popover.Slot
component.
The following example illustrates how you can wrap a component using a
Popover
and have those popovers render to a single location in the DOM.
/**
* External dependencies
*/
import { Popover, SlotFillProvider } from '@wordpress/components';
/**
* Internal dependencies
*/
import { MyComponentWithPopover } from './my-component';
const Example = () => {
<SlotFillProvider>
<MyComponentWithPopover />
<Popover.Slot />
</SlotFillProvider>;
};
This package exposes its own types for the components it exports, however it doesn't export its own types for component props. If you need to extract the props type, please use React.ComponentProps
to get the types from the element.
import type { ComponentProps } from 'react';
import { Button } from '@wordpress/components';
export default function MyButton( props: ComponentProps< typeof Button > ) {
return <Button { ...props }>Click Me!</Button>;
}
You can browse the components docs and examples at https://wordpress.github.io/gutenberg/
This is an individual package that's part of the Gutenberg project. The project is organized as a monorepo. It's made up of multiple self-contained software packages, each with a specific purpose. The packages in this monorepo are published to npm and used by WordPress as well as other software projects.
To find out more about contributing to this package or Gutenberg as a whole, please read the project's main contributor guide.
This package also has its own contributing information where you can find additional details.
FAQs
UI components for WordPress.
The npm package @wordpress/components receives a total of 155,118 weekly downloads. As such, @wordpress/components popularity was classified as popular.
We found that @wordpress/components demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 23 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.