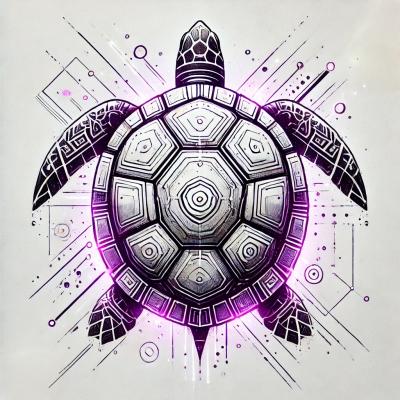
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
@xiphoo/react-native-nfc-manager
Advanced tools
Modified NFC module for react native. Original v.3.1.0 adapted for Android 12
Bring NFC feature to React Native. Inspired by phonegap-nfc and react-native-ble-manager
Contributions are welcome!
We also have a slack channel, you're welcome to chat with us for any issue or idea! join us here
npm i --save react-native-nfc-manager
This library use native-modules, so you will need to do pod install
for iOS:
cd ios && pod install && cd ..
For Android, it should be properly auto-linked, so you don't need to do anything.
Please see here
Please see this project: React Native NFC ReWriter App
v2
to v3
is primarily a refactor, to let long-term maintain easier. During the refactor, there're also several major enhancements:
getter
from main NfcManager
object to access them. This way we can avoid namespace corrupting due to individual tech methods.compatibility layer
for common NFC tech handler, such as NfcA
or IsoDep
, so we don't need to do lots of if/else according to Platform.OS
.If all you want to do is to read NDEF
data, you can use this example:
import NfcManager, {NfcEvents} from 'react-native-nfc-manager';
// Pre-step, call this before any NFC operations
async function initNfc() {
await NfcManager.start();
}
function readNdef() {
const cleanUp = () => {
NfcManager.setEventListener(NfcEvents.DiscoverTag, null);
NfcManager.setEventListener(NfcEvents.SessionClosed, null);
};
return new Promise((resolve) => {
let tagFound = null;
NfcManager.setEventListener(NfcEvents.DiscoverTag, (tag) => {
tagFound = tag;
resolve(tagFound);
NfcManager.setAlertMessageIOS('NDEF tag found');
NfcManager.unregisterTagEvent().catch(() => 0);
});
NfcManager.setEventListener(NfcEvents.SessionClosed, () => {
cleanUp();
if (!tagFound) {
resolve();
}
});
NfcManager.registerTagEvent();
});
}
Anything else, ex: write NDEF, send custom command, please read next section.
In high level, there're 3 steps to perform advanced NFC operations:
NfcManager
object, including:
For example, here's an example to write NDEF:
import NfcManager, {NfcTech, Ndef} from 'react-native-nfc-manager';
// Pre-step, call this before any NFC operations
async function initNfc() {
await NfcManager.start();
}
async function writeNdef({type, value}) {
let result = false;
try {
// Step 1
await NfcManager.requestTechnology(NfcTech.Ndef, {
alertMessage: 'Ready to write some NDEF',
});
const bytes = Ndef.encodeMessage([Ndef.textRecord('Hello NFC')]);
if (bytes) {
await NfcManager.ndefHandler // Step2
.writeNdefMessage(bytes); // Step3
if (Platform.OS === 'ios') {
await NfcManager.setAlertMessageIOS('Successfully write NDEF');
}
}
result = true;
} catch (ex) {
console.warn(ex);
}
// Step 4
NfcManager.cancelTechnologyRequest().catch(() => 0);
return result;
}
To see more examples, please see React Native NFC ReWriter App
Please see here
Please see here
Please see v2 branch
FAQs
Modified NFC module for react native. Original v.3.1.0 adapted for Android 12
The npm package @xiphoo/react-native-nfc-manager receives a total of 0 weekly downloads. As such, @xiphoo/react-native-nfc-manager popularity was classified as not popular.
We found that @xiphoo/react-native-nfc-manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.