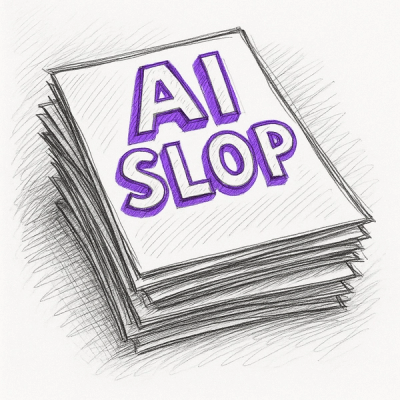
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
@zenginehq/backend-http
Advanced tools
Helper library for working with znHttp in Zengine backend plugins.
Helper module for working with znHttp in Zengine backend Plugins.
npm i @zenginehq/backend-http
var $api = require('@zenginehq/backend-http')();
// Example Response success and failure handlers.
znHttp().get(path)
.then($api.formatResponse)
.then(data => {
// data will be the Zengine object(s) you requested without all the metadata distractions
})
.catch($api.errHandler);
// Fetch *all* records across multiple pages if available, with any special parameters included in the second arg.
$api.fetchBatched(path, { limit: 100, 'not-field123': 'some value' })
.then(results => {
console.log(results.length);
});
// Load an existing record.
$api.getRecord(formId, recordId).then(record => {
// do something with `record`
});
// Update an existing record.
// data should be a single object.
// options should be an object and can include specific headers and a specific amount of retry attempts.
// delay should be provided in milliseconds.
// options and delay are optional.
$api.updateRecord(formId, recordId, data, options, delay);
// Query form records.
// options should be an object and can include a results limit, sorting options, and a Zengine filter.
$api.queryRecords = (formId, options);
// Delete an existing record.
$api.deleteRecord(formId, recordId);
// Create a new record. data can be an array of objects or a single object
$api.createRecord(formId, data).then(recordId => {
// the record ID(s) from the successful create (or update) operation.
// Important: it will always be the ID(s), not the entire record(s)
});
// Move a record to a folder.
$api.moveRecord(formId, recordId, folderId);
Special Note
To update multiple records on the same form, use the createRecord
method and include the IDs of each object to ensure they are updated, rather than having new records created.
// Loads an activity by id, useful for working with webhooks.
const activity = await $api.getActivity(activityId);
// Loads a form by id.
const form = await $api.getForm(formId);
3.2.1 (2019-09-16)
<a name="3.2.0"></a>
FAQs
Helper library for working with znHttp in Zengine backend plugins.
We found that @zenginehq/backend-http demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.