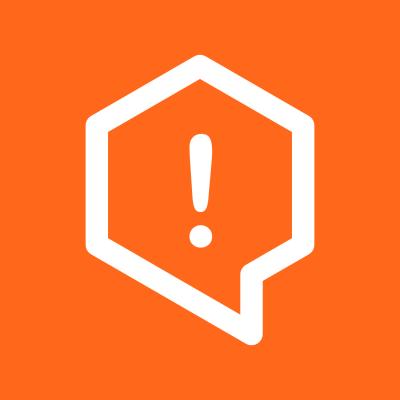
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
##About
This is a simple Node.JS API for accessing PSN data.
This is heavily based on work by psnapi.org and gumer-psn.
Note: v0.1.x is a completely new API that is incompatible with 0.0.x. Please take care when writing your dependancies.
##Installing
You can install it with the package manager
npm install PSNjs
Or clone the repository and install the dependencies
git clone https://github.com/cubehouse/PSNjs.git
cd PSNjs/
npm install
##API Setup
var PSNjs = require('PSNjs');
var psn = new PSNjs({
// PSN email and password
email: "PSNEMAIL",
password: "PSNPASSWORD",
// debug printing
debug: true,
// optionally store session tokens in a file to speed up future connection
authfile: ".psnAuth"
});
// get the above user's trophies
psn.getUserTrophies(function(error, data) {
// check for an error
if (error)
{
console.log("Error fetching trophies: " + error);
return;
}
// success! print out trophy data
console.log(JSON.stringify(data, null, 2));
});
###Other Init variables
{
email: "PSNEMAIL", // your email
password: "PSNPASSWORD", // your password
debug: true, // enable debug logging?
requestDebug: false, // enable the request library's debug output?
autoconnect: false, // make a PSN request immediately (make sure you use onReady if you do this)
authfile: ".psnAuth", // optionally store PSN session tokens in this file
onReady: function() {
// this function will be called when the API is ready (mainly used when autoconnect is true)
}
}
##Custom Save and Load Callbacks
If you don't want to use the authfile option, you can manually write save/load functions. For example, using Redis or something instead of the filesystem.
var psn = new PSNjs({
// PSN email and password
email: "PSNEMAIL",
password: "PSNPASSWORD"
});
// load example (data should be a Base64 string)
psn.Load("SAVED DATA", function(error) {
if (error)
{
console.log("Error loading data: " + error);
return;
}
// load successful!
});
// save example
psn.OnSave(function(data, callback) {
// save data
// data will be a Base64 string
mySaveSystem.save(data, function() {
// all done!
// always call the callback so the API knows you're done saving!
// handle your own error reporting and debugging
callback();
});
});
Get a PSN user's profile
Get current user's message groups
Get data from a specific message. All this data can be found in getMessageGroups
Get the signed-in user's activity feed
Like an activity from the activity feed
Dislike an activity from the activity feed
Get notifications of currently authenticated user
Add a friend to PSN (must have received a friend request from the user)
Remove a friend from PSN
Send a friend request to a user
Get the user's friend list
Generate a friend URL you can give to people to add you as a friend.
Fetch trophy data for the logged in user (and optionally compare to another user)
Get list of trophy groups for a title (eg. base game + DLC packs)
Get a title's trophies (supplying a trophy group), optionally comparing to another user.
Get data on a specific trophy in a title with supplied trophyId. Optionally compare to a username.
FAQs
API Framework for PSN
We found that PSNjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.