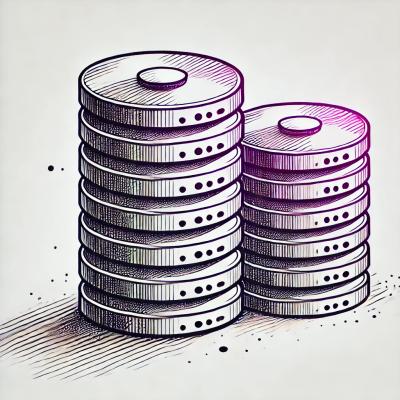
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
SenseOrm is cross-db ORM, providing common interface to access most popular database formats. Currently supported are: mongodb, redis, mysql and js-memory-storage. You can add your favorite database adapter, checkout one of the existing adapters to learn how, it's super-easy, I guarantee.
npm install senseorm
var Schema = require('senseorm').Schema;
var s = new Schema('mongoose');
// define models
var Post = schema.define('Post', {
title: { type: String, length: 255 },
content: { type: Schema.Text },
date: { type: Date, default: Date.now },
published: { type: Boolean, default: false }
});
// simplier way to describe model
var User = schema.define('User', {
name: String,
bio: Schema.Text,
approved: Boolean,
joinedAt: Date,
age: Number
});
// setup relationships
User.hasMany(Post, {as: 'posts', foreignKey: 'user_id'});
// creates instance methods:
// user.posts(conds)
// user.posts.build(data) // like new Post({user_id: user.id});
// user.posts.create(data) // build and save
Post.belongsTo(User, {as: 'author', foreignKey: 'user_id'});
// creates instance methods:
// post.author(callback) -- getter when called with function
// post.author() -- sync getter when called without params
// post.author(user) -- setter when called with object
s.automigrate(); // required only for mysql NOTE: it will drop User and Post tables
// work with models:
var user = new User;
user.save(function (err) {
var post = user.posts.build({title: 'Hello world'});
post.save(console.log);
});
// Common API methods
// just instantiate model
new Post
// save model (of course async)
Post.create(cb);
// all posts
Post.all(cb)
// all posts by user
Post.all({where: {userId: user.id}});
// the same as prev
user.posts(cb)
// same as new Post({userId: user.id});
user.posts.build
// save as Post.create({userId: user.id}, cb);
user.posts.create(cb)
// find instance by id
User.find(1, cb)
// count instances
User.count(cb)
// destroy instance
user.destroy(cb);
// destroy all instances
User.destroyAll(cb);
// Setup validations
User.validatesPresenceOf('name', 'email')
User.validatesLengthOf('password', {min: 5, message: {min: 'Password is too short'}});
User.validatesInclusionOf('gender', {in: ['male', 'female']});
User.validatesExclusionOf('domain', {in: ['www', 'billing', 'admin']});
User.validatesNumericalityOf('age', {int: true});
User.validatesUniquenessOf('email', {message: 'email is not unique'});
user.isValid(function (valid) {
if (!valid) {
user.errors // hash of errors {attr: [errmessage, errmessage, ...], attr: ...}
}
})
The following callbacks supported:
- afterInitialize
- beforeCreate
- afterCreate
- beforeSave
- afterSave
- beforeUpdate
- afterUpdate
- beforeDestroy
- afterDestroy
- beforeValidation
- afterValidation
Each callback is class method of the model, it should accept single argument: next
, this is callback which
should be called after end of the hook. Except afterInitialize
because this method is syncronous (called after new Model
).
```javascript
var user = new User;
// afterInitialize
user.save(callback);
// beforeValidation
// afterValidation
// beforeSave
// beforeCreate
// afterCreate
// afterSave
// callback
user.updateAttribute('email', 'email@example.com', callback);
// beforeValidation
// afterValidation
// beforeUpdate
// afterUpdate
// callback
user.destroy(callback);
// beforeDestroy
// afterDestroy
// callback
User.create(data, callback);
// beforeValidate
// afterValidate
// beforeCreate
// afterCreate
// callback
```
Read the tests for usage examples: ./test/common_test.js
All tests are written using nodeunit:
nodeunit test/common_test.js
If you run this line, of course it will fall, because it requres different databases to be up and running, but you can use js-memory-engine out of box! Specify ONLY env var:
ONLY=memory nodeunit test/common_test.js
of course, if you have mongoose running, you can run
ONLY=mongoose nodeunit test/common_test.js
Now all common logic described in ./lib/*.js
, and database-specific stuff in ./lib/adapters/*.js
. It's super-tiny, right?
FAQs
ORM for every database: mongodb, mysql, redis
The npm package SenseOrm receives a total of 1 weekly downloads. As such, SenseOrm popularity was classified as not popular.
We found that SenseOrm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.