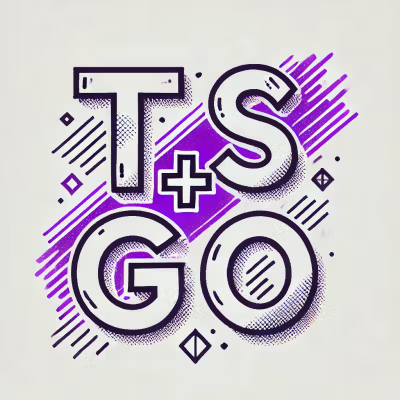
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
abstract-type
Advanced tools
The abstract-type library includes the abstract `Type` class and `Value` class for streamable type info and validating value.
The abstract-type library includes the abstract Type
class and Value
class for streamable type info and validating value.
name
and required
attributes.
name
(string): the type name.
required
(boolean): the attribute whether is required(must be exists, not optional).
default to false.The type has a name and can verify whether a value belongs to that type. We can draw the two concepts related to the type, from here:
Attributes: the attributes(meta data) of this type.
Value: the value of this type.
The Type Class
_initialize(aOptions)
: initialize the type object._assign(options)
: assign an options of type to itself._validate(aValue, aOptions)
: validate a value whether is valid.valueToString(aValue)
: (optional) convert the value to string, it's used to convert to json.toValue(aString)
: (optional) convert the string to the value, it's used to convert from json and assign from value.ValueType
property: (optional) defaults to Value
Class. unless implement your own Value class.The Value Class
value
: store the value here.$type
(Type): point to the type of this value.tryGetTypeName(Value)
: try to guess the type name of the value.constructor(value[, type[, options]])
: create a value instance.
value
: the assigned value. it will guess the type of the value if no type provided.type
(Type): the type of the valueoptions
(object): the optional type of value options. it will create a new type if exists.clone()
: clone this value object.assign(value, options)
: assign the value.
aOptions
(object):
checkValidity
(boolean): defaults to true.fromJson(json)
: assign a value from json string.createFromJson(json)
: create a new value object from json string.isValid()
: whether the value is valid.toObject(aOptions)
: return a parametric object of the value. it wont include type info.
unless set the withType
is true.
withType
(boolean): whether includes the type info. default to false_toObject(aOptions)
: return the parametric object of this value.valueOf()
: return the value._assign(value)
: assign the value to itself.The Attributes class: describe the attributes of a type. an attribute could include these properties:
name
(string): the attribute name. you can specify a non-english name.
name
only used on export(toObject
) or import(assign
).type
(string): the attribute type.enumerable
(boolean): the attribute whether is a hidden attribute, defaults to true.
required
(boolean): the attribute whether it's required(MUST HAVE).value
: the default value of the attribute.assign(value, dest, src, key)
(function): optional special function to assign the attribute's value
from src[key
] to dest[key
].
TypeAttributes = require 'abstract-type/lib/attributes'
Type = require 'abstract-type'
register = Type.register
aliases = Type.aliases
class NumberType
register NumberType
aliases NumberType, 'number'
$attributes: TypeAttributes
min:
name: 'min'
type: 'Number'
max:
name: 'max'
type: 'Number'
#valueToString: (aValue)->
# aValue = String(aValue)
stringToValue: (aString)->
if isInt aString
aString = parseInt(aString)
else if isFloat aString
aString = parseFloat(aString)
else
aString = undefined
aString
_validate: (aValue, aOptions)->
aValue = @stringToValue(aValue) if isString aValue
result = isNumber aValue
if result
if aOptions
vMin = aOptions.min
vMax = aOptions.max
if vMin?
result = aValue >= vMin
if not result
@error "should be equal or greater than minimum value: " + vMin
if result and vMax?
result = aValue <= vMax
if not result
@error "should be equal or less than maximum value: " + vMax
result
var cacheable = require('cache-factory')
var Type = cacheable(require('abstract-type')) // apply the cache-able ability to Type
require('number-type') //register the number type to Type.
var number = Type('Number') // get the number type object.
assert.equal(number, Type('Number'))
var num = Type('Number', {min:1, max:6}) // create non-name a virutal type object.
assert.notEqual(number, num)
var NumberType = Type.registeredClass('Number') // get Number Type Class
// create a virtual type object
var TPositiveNumber = Type('Number', {min:0, cached: 'PositiveNumber'})
assert.notOk(TPositiveNumber.isValid(-1))
assert.ok(TPositiveNumber.isValid(1)) // validate a value
var n = TPositiveNumber.create(123) // create the value
n = TPositiveNumber.createValue(123)
assert.ok(n.isValid())
assert.equal(Number(n) + 3, 126)
//get the virtual type from cache:
var N = Type('/type/AbstractNumber/Number/PositiveNumber')
//N = NumberType('PositiveNumber')
assert.equals(N, TPositiveNumber);
It's the abstract type info class and the type info manager.
constructor([typeName, ]options)
: get a the default type info instance object from global cache or create a new virutal type object.
arguments
typeName
(string): the type name.options
(object): optional type options to apply. different types have different options.
name
(string): the type name.validate
(function): assign the custom validate function.
function(value)
the first argument is the value to validate.
customValidate
property internal....
: the type's specified options to create a new virtual type object.return
eg:
// get the default type object
var TNumber = Type('number')
// other way to get the default type object
TNumber = Type({name: 'number'})
// another way to get the default type object
TNumber = NumberType()
// create a new virtual type object.
var TPositiveNumber = Type('number', {min:0})
assert.notEqual(TPositiveNumber, TNumber)
// create a new virtual type object.
TPositiveNumber = Type('number', {validate: function(v){return v>=0}})
assert.notEqual(TPositiveNumber, TNumber)
Type.create(typeName, options)
:This class method is used to create a new Type instance object.
typeName
(string): the type name.options
(object): optional type options. different types have different options.Type.createFrom(aObject)
: the class method to create a type object or value object from a parametric type object.
aObject
(object): the encoding string should be decoded to an object.
name
(string): the type name required.value
: the optional value. return value object if exists.Type.createFromJson(json)
:the class method to create a type object or value object from a json string.
json
(string): the json string with type info.
name
(string): the type name required.value
: the optional value. return value object if exists.Type.registerValidator(aValidator)
:This class method is used to register a custom validator.
aValidator
(object): the validator.
name
(string): the validator option name.validate
(function): the validator function: function(value, options)
Type.registerValidator(name, validate)
:This class method is used to register a custom validator.
name
(string): the validator option name.validate
(function): the validator function: function(value, options)
Type.unregisterValidator(name)
:This class method is used to unregister a custom validator.
name
(string): the validator name to unregister.cloneType()
:the instance method to clone the type object itself.
alias: clone
return
eg:
var num = Type('Number', min:1)
var num1 = num.cloneType()
assert.ok(num.isSame(num1))
createType(options)
: create a new the type object of this type with the type options.
options
(object): optional type options. different types have different options.
cloneType()
if no optionscreateValue(value, options)
:create a value from the type.
value
(Type): the value of this type to createoptions
(object): optional type options
toObject(aObject, aNameRequired = true)
:convert the type info into aObject(an parametric type object).
It could be streamable your type.
options
(object): optional options
value
(Type): optional value, when value exists, the following options used:typeOnly
(boolean): just type info if true. defaults to false.aNameRequired
(boolean): write the name to aObject. defaults to true.toJson(options)
:convert the type info to a json string. It could be streamable your type.
It is almost equivalent to JSON.stringify(theTypeObject).
options
(object): optional options
value
(Type): optional value, when value exists, the following options used:typeOnly
(boolean): just type info if true. defaults to false.validate(value, raiseError, options)
:validate a specified value whether is valid.
value
(Type): the value to validateraiseError
(boolean): whether throw error if validate failed. defaults to true.options
(object): optional type options to override. defaults to this type options.the value class.
You should implement the valueToString(aValue)
and stringToValue(aString)
method in your derived type class
to make the value streamable.
constructor(value[[, type], options])
: create a value object.
value
(Type): the value to be created.
type
(Object): the optional type object.options
(object): optional type options.
$type
: point to a type object. It can not be enumerable.clone()
: clone the value object.
create(value, options)
:create a new the value object.
value
(Type): the value to be created. MUST BE the same type.options
(object): optional type options.
assign(value, options)
:assign a value to itself.
value
(Type): the value to be assigned. MUST BE the same type.options
(object): optional type options.
this
object.isValid()
: validate the value whether is valid.
toObject(options)
:convert the value to an object. It wont include type info via defaults.
It could be streamable your value.
arguments
options
(object): optional options
withType
(Boolean): whether with type info, defaults to false.return
withType
).eg:
var Type = require('abstract-type')
var Value = Type.Value
var val = Value(1, Type 'Number')
assert.equal val.toObject(), 1
MIT
FAQs
The abstract-type library includes the abstract `Type` class and `Value` class for streamable type info and validating value.
The npm package abstract-type receives a total of 4 weekly downloads. As such, abstract-type popularity was classified as not popular.
We found that abstract-type demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.