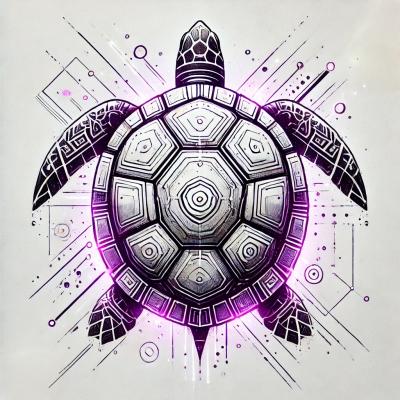
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
action-typed
Advanced tools
Better type-safety, with less actual typing for Redux actions
npm i action-typed
# or
yarn add action-typed
Video walkthrough if you prefer: https://www.youtube.com/watch?v=v263zMyVv6k
{type, payload}
s, it feels more like working with type constructorsuser.actions.ts
import {ActionHandler, msgCreator} from "action-typed";
// this replaces any action-creators you may have 😍
const messages = {
SignedIn: (firstname: string, lastname: string) => ({firstname, lastname}),
Token: (token: string) => token,
SignOut: () => undefined,
};
export const Msg = msgCreator(messages);
export type Handler = ActionHandler<typeof messages>
index.ts
import {combineReducers, createStore} from "redux";
import {userReducer} from "./user.reducer";
import {Msg} from "./user.actions";
const root = combineReducers({
user: userReducer
});
const store = createStore(root);
store.dispatch(
// you can't make a mistake here - the string "SignedIn" is type-safe, and it
// dictates what the remaining parameters should be 👌
Msg("SignedIn", "shane", "osbourne")
);
user.reducer.ts
import {Handler} from "./user.actions";
type State = {
token: string
};
const initialState: State = { token: "" };
//
// this uses the helper union type that's inferred from the JS object
// ↓
export function userReducer(state = initialState, action: Handler): State {
switch (action.type) {
// matching "Token" here narrows the type of `action`
// that means you get full type-safety on the value of 'payload' 👌
case "Token": {
return { ...state, token: action.payload }
}
}
return state;
}
component example mapDispatchToProps
import React, { Component } from 'react';
import {connect} from "react-redux";
import {Msg} from "./actions/counter.actions";
import {StoreState} from "./configureStore";
type AppProps = {
Msg: typeof Msg,
counter: number,
}
class App extends Component<AppProps> {
render() {
const {Msg, counter} = this.props;
return (
<div className="App">
{counter}
<button onClick={() => Msg("Increment")}>Increment</button>
<button onClick={() => Msg("Decrement", 20)}>Decrement by 20</button>
</div>
);
}
}
export default connect(
(x: StoreState) => ({ counter: x.counter }),
// don't map all your separate action-creators here
{Msg}
)(App);
FAQs
Better type *safety*, with less actual *typing* for Redux actions
The npm package action-typed receives a total of 260 weekly downloads. As such, action-typed popularity was classified as not popular.
We found that action-typed demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.