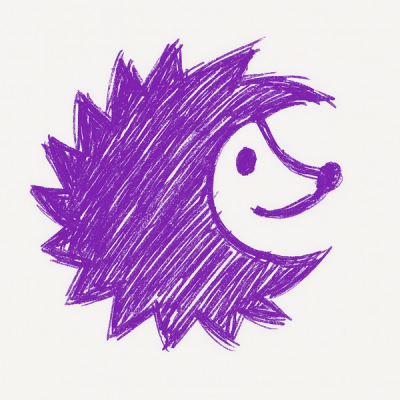
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
adonisjs-websocket
Advanced tools
node ace add adonisjs-websocket
Simple example:
// start/routes.ts
router.ws('/ws', ({ ws }) => {
ws.on('message', (message) => {
ws.send('Received: ' + message.toString())
})
ws.on('close', () => {
console.log('Connection closed')
})
ws.send('Hello! Your id is ' + ws.id)
})
npx wscat -c "ws://localhost:3333/ws"
Middleware and broadcasting:
// start/routes.ts
router.ws(
'/rooms/:roomId',
({ ws, params, auth }) => {
const roomId = params.roomId
const user = auth.user
if (user.isBanned) {
return ws.close()
}
ws.on('message', (message) => {
ws.send('Received: ' + message.toString())
})
// broadcast to all clients of the same url path
// you can enable redis in `config/websocket.ts` to broadcast on all web server instances
await ws.broadcast('Hello everyone!')
},
[
// you can enable them globally in `config/websocket.ts`
() => import('#middleware/container_bindings_middleware'),
() => import('@adonisjs/auth/initialize_auth_middleware'),
middleware.auth(),
]
)
npx wscat -c 'ws://localhost:3333/rooms/1' -H 'Authorization: Bearer oat_MjU.Z25o...'
npx wscat -c 'ws://localhost:3333/rooms/2?token=oat_MjU.Z25o...'
npx wscat -c 'ws://localhost:3334/rooms/2?token=oat_MjU.Z25o...'
Using controllers:
// start/routes.ts
const WsChatController = () => import('#controllers/ws/chat_controller')
router.ws('/chat', [WsChatController, 'handle'])
// app/controllers/ws/chat_controller.ts
import type { WebSocketContext } from 'adonisjs-websocket'
export default class ChatController {
public async handle({ ws }: WebSocketContext) {
ws.on('message', (message) => {
ws.send('Received: ' + message.toString())
})
ws.send('Hello! Your id is ' + ws.id)
}
}
For browsers, it's common practice to send a small message (heartbeat) for every given time passed (e.g every 30sec) to keep the connection active.
// frontend
const ws = new WebSocket('wss://localhost:3333/ws')
const HEARTBEAT_INTERVAL = 30000
let heartbeatInterval
ws.onopen = () => {
heartbeatInterval = setInterval(() => {
ws.send('ping')
}, HEARTBEAT_INTERVAL)
}
ws.onclose = () => {
clearInterval(heartbeatInterval)
}
// backend
router.ws('/ws', ({ ws }) => {
ws.on('message', (message) => {
if (message.toString() === 'ping') {
ws.send('pong')
}
})
})
FAQs
Websocket provider for AdonisJS
The npm package adonisjs-websocket receives a total of 54 weekly downloads. As such, adonisjs-websocket popularity was classified as not popular.
We found that adonisjs-websocket demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.