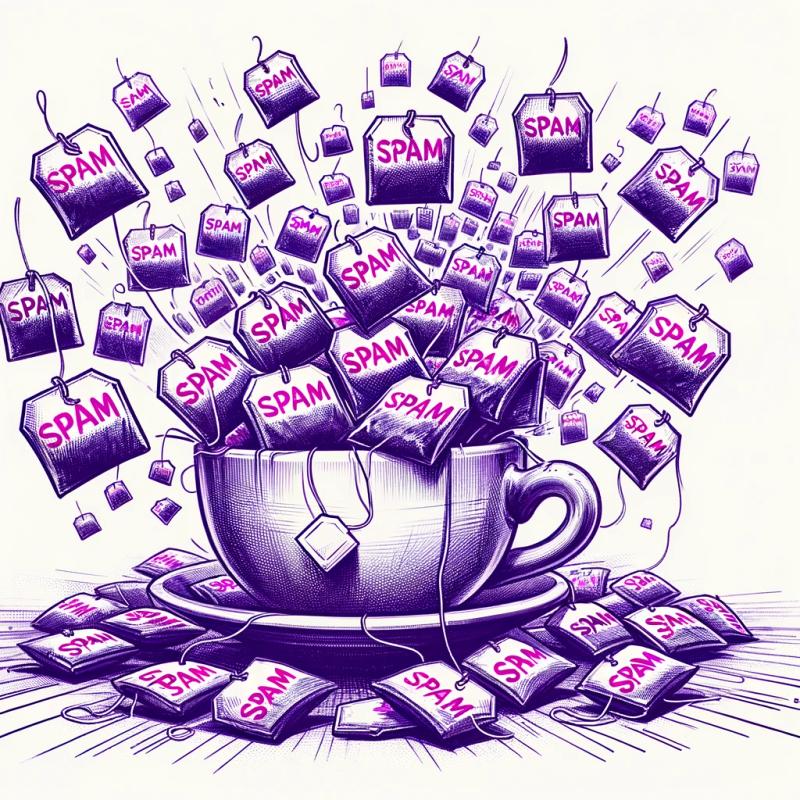
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
afterfn
Advanced tools
Readme
afterfn
Simply executes after the function is called.
afterfn.return
Allows manipulation of return value.
function doubleNumber(x) {
return x * 2
}
// calls 'Math.abs' after 'doubleNumber'
// after.return allows manipulation of return value
var doubleNumberAbs = after.return(doubleNumber, Math.abs)
var calledWith = []
var results = []
doubleNumberAbs = after(doubleNumberAbs, function fn() {
calledWith = calledWith.concat(fn.args)
results = results.concat(fn.value)
// after ignores return value of after function.
})
console.log(doubleNumberAbs(10)) // => 20
console.log(doubleNumberAbs(-10)) // => 20
console.log(doubleNumberAbs(-100)) // => 200
console.log(calledWith) // => [ 10, -10, -100 ]
console.log(results) // => [ 20, 20, 200 ]
var myPackage = {
name: 'my-package',
version: 1,
build: function() {
// build routine
},
bumpVersion: function() {
this.version++
}
}
// always calls 'myPackage.build' *after* 'myPackage.bumpVersion'
myPackage.build = after(myPackage.build, myPackage.bumpVersion)
console.log('%s@v%s', myPackage.name, myPackage.version) // => my-package@v1
myPackage.build()
console.log('%s@v%s', myPackage.name, myPackage.version) // => my-package@v2
afterfn
returns a new Function.afterfn
ignores return value of after functionafterfn.return
allows manipulation of return valuethis
context is maintained.MIT
FAQs
Invoke a function after a function.
The npm package afterfn receives a total of 15 weekly downloads. As such, afterfn popularity was classified as not popular.
We found that afterfn demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.