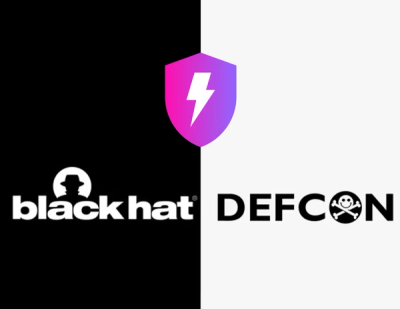
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
aggregate-base
Advanced tools
Base class for aggregate operation, sush as http request, write file.
Base class for aggregate operation, sush as http request, write file.
npm i aggregate-base --save
You can wrap Class for aggregate operation, if you have a Logger that write log to file.
class Logger {
info(log) {
this.writeToFile(log);
}
writeToFile() {
// your implementation
}
}
However, it has bad performance that write file system every function call, so you can use this module to merge the operations.
const { wrap } = require('aggregate-base');
class Logger {
info(log) {
this.writeToFile(log);
}
flush(logs) {
this.writeToFile(logs.join('\n'));
}
writeToFile() {
// your implementation
}
}
const WrapLogger = wrap(Logger, {
interval: 1000,
intercept: 'info',
flush: 'flush',
});
The module will create a loop (configured by interval), it will collect data by intercepting the specified intercept
method, and call flush
method after interval
with all collected data.
FAQs
Base class for aggregate operation, sush as http request, write file.
The npm package aggregate-base receives a total of 39 weekly downloads. As such, aggregate-base popularity was classified as not popular.
We found that aggregate-base demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.