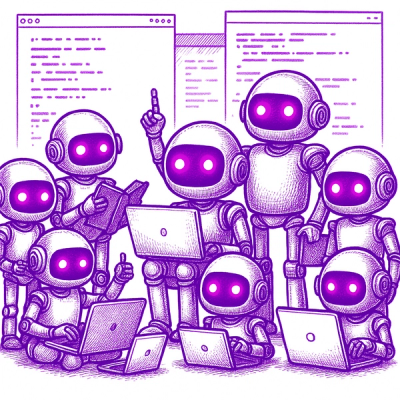
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
airtable-ts-codegen
Advanced tools
Autogenerate TypeScript definitions for your Airtable base
Run with:
AIRTABLE_API_KEY=pat1234.abcd AIRTABLE_BASE_ID=app1234 npx airtable-ts-codegen
This will output a file app1234.ts
that exports all the table definitions
You can also generate TypeScript definitions based on a specific view, which will only include the fields visible in those views:
AIRTABLE_API_KEY=pat1234.abcd AIRTABLE_BASE_ID=app1234 AIRTABLE_VIEW_IDS=viw1234,viw5678 npx airtable-ts-codegen
This will output a file app1234.ts
that exports table definitions with only the fields visible in the specified views.
/* DO NOT EDIT: this file was automatically generated by airtable-ts-codegen */
/* eslint-disable */
import { Item, Table } from 'airtable-ts';
export interface Task extends Item {
id: string,
name: string,
status: string,
dueAt: number,
isOptional: boolean,
}
export const tasksTable: Table<Task> = {
name: 'Tasks',
baseId: 'app1234',
tableId: 'tbl5678',
mappings: {
name: 'fld9012',
status: 'fld3456',
dueAt: 'fld7890',
isOptional: 'fld1234',
},
schema: {
name: 'string',
status: 'string',
dueAt: 'number',
isOptional: 'boolean',
},
};
You can then easily use this with airtable-ts, for example:
import { AirtableTs } from 'airtable-ts';
import { tasksTable } from './generated/app1234';
const db = new AirtableTs({ apiKey: 'pat1234.abcdef' });
const allTasks = await db.scan(tasksTable);
// You now have all the benefits of airtable-ts, without having to define schemas manually 🎉
Pull requests are welcomed on GitHub! To get started:
npm install
npm run test
to run testsnpm run build
npm start
Versions follow the semantic versioning spec.
To release:
npm version <major | minor | patch>
to bump the versiongit push --follow-tags
to push with tagsFAQs
Autogenerate TypeScript definitions for your Airtable base
The npm package airtable-ts-codegen receives a total of 98 weekly downloads. As such, airtable-ts-codegen popularity was classified as not popular.
We found that airtable-ts-codegen demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.