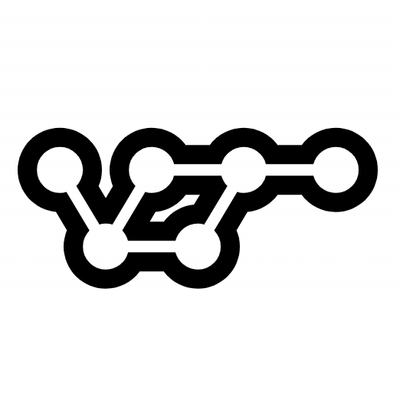
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
A node client for interacting with Adventure Land - The Code MMORPG. This package extends the functionality of 'alclient' by managing a mongo database.
This is a node client for the game Adventure Land - The Code MMORPG.
This code is NOT compatible with scripts written directly in the game. If you are just looking for a way to run your code headless, or with fewer resources, I recommend trying caracAL.
npm init
and enter prompts. Check out this link for more information.npm install typescript
. Save your files as .ts
files instead of .js
files.npx tsc
.In your tsconfig.json, make sure "esModuleInterop": true
is set.
In your package.json, make sure "type": "module"
is set.
npm install alclient
.credentials.json
file that looks like this:{
"email": "hyprkookeez@gmail.com",
"password": "thisisnotmyrealpasswordlol"
}
You can also optionally add a Mongo URI to track various data with a mongo database.
{
"email": "hyprkookeez@gmail.com",
"password": "thisisnotmyrealpasswordlol",
"mongo": "mongodb://localhost:27017/alclient"
}
import AL from "alclient"
async function run() {
await Promise.all([AL.Game.loginJSONFile("../credentials.json"), AL.Game.getGData()])
await AL.Pathfinder.prepare(AL.Game.G)
// Set `<<MERCHANT_NAME>>` to your merchant
const merchant = await AL.Game.startMerchant("<<MERCHANT_NAME>>", "ASIA", "I")
console.log("Moving to main")
await merchant.smartMove("main")
console.log("Moving to cyberland")
await merchant.smartMove("cyberland")
console.log("Moving to halloween")
await merchant.smartMove("halloween")
merchant.disconnect()
}
run()
move()
are on the Character
in alclient.import AL from "alclient"
async function run() {
await AL.Game.loginJSONFile("../credentials.json")
const ranger = await AL.Game.startRanger("earthiverse", "US", "I")
while (true) {
await ranger.move(50, 50)
await ranger.move(50, -50)
await ranger.move(-50, -50)
await ranger.move(-50, 50)
}
}
run()
attack()
is basicAttack()
.import AL from "alclient"
async function run() {
await Promise.all([AL.Game.loginJSONFile("../credentials.json"), AL.Game.getGData()])
await AL.Pathfinder.prepare(AL.Game.G)
const ranger = await AL.Game.startRanger("earthiverse", "US", "I")
await ranger.smartMove("hen")
while (true) {
if (ranger.canUse("attack") && ranger.isPVP()) {
// We can attack players
for (const [, player] of ranger.players) {
if (AL.Tools.distance(ranger, player) > ranger.range) continue // Too far to attack
// We found a player to attack!
await ranger.basicAttack(player.id)
break
}
}
if (ranger.canUse("attack")) {
for (const [, entity] of ranger.entities) {
if (AL.Tools.distance(ranger, entity) > ranger.range) continue // Too far to attack
// We found an entity to attack!
await ranger.basicAttack(entity.id)
break
}
}
}
}
run()
FAQs
A node client for interacting with Adventure Land - The Code MMORPG. This package extends the functionality of 'alclient' by managing a mongo database.
The npm package alclient receives a total of 21 weekly downloads. As such, alclient popularity was classified as not popular.
We found that alclient demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.