Comparing version 0.0.2 to 0.0.4
48
index.js
var util = require('util'), | ||
Transform = require('stream').Transform; | ||
const Transform = require('stream').Transform; | ||
util.inherits(Aline, Transform); | ||
class Aline extends Transform { | ||
constructor(options) { | ||
super(); | ||
function Aline(options) { | ||
if (!(this instanceof Aline)) | ||
return new Aline(options); | ||
Transform.call(this, options); | ||
this._tail = Buffer.alloc(0); | ||
this._separator = (options && options.separator) || '\n'; | ||
} | ||
Aline.prototype._transform = function (chunk, encoding, callback) { | ||
var index = chunk.lastIndexOf(this._separator); | ||
if (index === chunk.length) { | ||
this._tail = Buffer.alloc(0); | ||
return callback(null, chunk); | ||
this._separator = (options && options.separator) || '\n'; | ||
} | ||
_transform(chunk, encoding, callback) { | ||
const index = chunk.lastIndexOf(this._separator); | ||
if (index === chunk.length || index === -1) { | ||
callback(null, Buffer.concat([this._tail, chunk])); | ||
this._tail = Buffer.alloc(0); | ||
return; | ||
} | ||
var head = Buffer.concat([this._tail, chunk.slice(0, index)]); | ||
this._tail = Buffer.from(chunk.slice(index + 1)); | ||
const head = Buffer.concat([this._tail, chunk.slice(0, index)]); | ||
this._tail = Buffer.from(chunk.slice(index + 1)); | ||
callback(null, head); | ||
}; | ||
callback(null, head); | ||
} | ||
_flush(callback) { | ||
callback(null, this._tail); | ||
} | ||
} | ||
Aline.prototype._flush = function(callback) { | ||
callback(null, this._tail); | ||
}; | ||
module.exports = Aline; |
{ | ||
"name": "aline", | ||
"version": "0.0.2", | ||
"version": "0.0.4", | ||
"description": "Align stream chunks to bound of lines", | ||
"main": "index.js", | ||
"engines": { | ||
"node": ">=v6.4.0" | ||
}, | ||
"scripts": { | ||
"test": "echo \"Error: no test specified\" && exit 1" | ||
"test": "mocha" | ||
}, | ||
@@ -23,3 +26,6 @@ "repository": { | ||
}, | ||
"homepage": "https://github.com/tugrul/node-aline#readme" | ||
"homepage": "https://github.com/tugrul/node-aline#readme", | ||
"devDependencies": { | ||
"mocha": "^7.1.1" | ||
} | ||
} |
@@ -5,6 +5,31 @@ # node-aline | ||
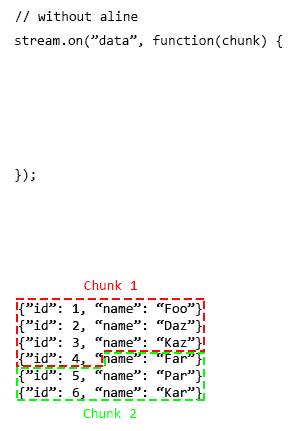 | ||
## Install | ||
### NPM | ||
``` | ||
npm install aline | ||
``` | ||
``` | ||
### Yarn | ||
``` | ||
yarn add aline | ||
``` | ||
## Sample | ||
```javascript | ||
const Aline = require('aline'); | ||
const {Readable} = require('stream'); | ||
const stream = Readable.from(async function* () { | ||
yield 'foo\nba'; | ||
yield 'ar\nbaz'; | ||
}).pipe(new Aline()); | ||
stream.on('data', function(chunk) { | ||
console.log(chunk.toString()); | ||
}); | ||
``` |
No tests
QualityPackage does not have any tests. This is a strong signal of a poorly maintained or low quality package.
Found 1 instance in 1 package
4759
78
1
35
1