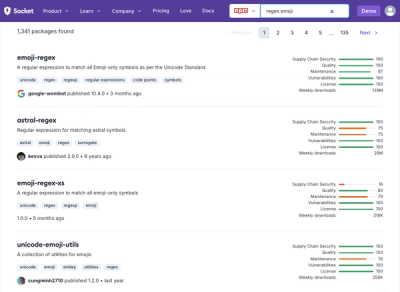
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Amazon Pay API Integration
Amazon Pay account:
To register for Amazon Pay, go to the Amazon Pay website
https://pay.amazon.com, choose your region from the
drop-down list in the upper right corner,
and then click Merchant Sign Up.
Node 8.0 or higher
Integration steps can be found below:
To use this module directly install it as a dependency:
npm install amazonpay
Instantiating the client: The sandbox parameter is defaulted to false if not specified:
const Client = require('amazonPayClient').amazonPayClient;
const configArgs = {
'merchantId' : 'MERCHANT1234567',
'accessKey' : 'ABCDEFGHI1JKLMN2O7',
'secretKey' : 'abc123Def456gHi789jKLmpQ987rstu6vWxyz/ds',
'clientId' : 'amzn1.application-oa2-client.45789c45a8f34927830be1d9e029f480',
'region' : 'us',
'currencyCode' : 'USD',
'sandbox' : true,
/**
* Below is a variable that can be passed that will convert
* the XML response to a JSON string or object instead.
* It is not part of the API values. If you specify this value in
* reqParam, this will overide configArgs value
* jsonResponse can be 'jsonString' for a JSON string
* or it can be 'jsonObject' for a JSON object
* @optional 'jsonResponse' - [String]
**/
'jsonResponse' : 'jsonString'
};
const client = new Client(configArgs);
Below is an example on how to make the SetOrderAtributes API call:
const reqParam = {
'amazonOrderReferenceId': amazonOrderReferenceId,
'amount': amount,
'orderItemCategory': orderItemCategories,
'storeName': storeName,
'sellerOrderId': sellerOrderId,
'sellerNote': sellerNote,
'customInformation': customInformation
};
const response = newClient.setOrderAttributes(reqParam);
Below is an example on how to make the ConfirmOrderReference API call:
const reqParam = {
'amazonOrderReferenceId': 'S01-8022464-2595273'
};
const response = client.confirmOrderReference(reqParam);
Below is an example on how to make the GetOrderReferenceDetails API call:
const reqParam = {
/** If you call this before Confirm, to get all the buyer
* address information you must pass 'accessToken'
* after Confirm, 'accessToken' is not used.
**/
'accessToken' : 'Atza|IwEBICpkg...',
'amazonOrderReferenceId': 'S01-8022464-2595273'
};
const response = newClient.getOrderReferenceDetails(reqParam);
Below is an example on how to make the Authorize API call:
/** For idempotency the AuthorizationReferenceId must be unique.
* To accomplish this we can generate a 32 character unique identifier
* using uuid/v4 https://www.npmjs.com/package/uuid and remove
* the dashes.
**/
const uuidv4 = require('uuid/v4');
const reqParam = {
'amazonOrderReferenceId' : 'S01-8022464-2595273',
'authorizationReferenceId' : uuidv4().toString().replace(/-/g, ''),
'amount' : '10',
'sellerNote' : 'Test note',
'transactionTimeout' : '0',
'captureNow' : 'false'
};
const response = client.authorize(reqParam);
Below is an example on how to make the GetAuthorizationDetails API call:
const reqParam = {
'amazonAuthorizationId': amazonAuthorizationId
};
const response = newClient.getAuthorizationDetails(reqParam);
Below is an example on how to make the Capture API call:
/** For idempotency the CaptureReferenceId must be unique.
* To accomplish this we can generate a 32 character unique identifier
* using uuid/v4 https://www.npmjs.com/package/uuid and remove
* the dashes.
**/
const uuidv4 = require('uuid/v4');
const reqParam = {
'amazonAuthorizationId': amazonAuthorizationId,
'captureReferenceId': uuidv4().toString().replace(/-/g, ''),
'amount': amount,
'sellerNote': 'Test note',
};
const response = newClient.capture(reqParam);
Below is an example on how to make the GetCaptureDetails API call:
const reqParam = {
'amazonCaptureId': amazonCaptureId
};
const response = newClient.getCaptureDetails(reqParam);
Below is an example on how to make the Refund API call:
/** For idempotency the RefundReferenceId must be unique.
* To accomplish this we can generate a 32 character unique identifier
* using uuid/v4 https://www.npmjs.com/package/uuid and remove
* the dashes.
**/
const uuidv4 = require('uuid/v4');
const reqParam = {
'amazonCaptureId': amazonCaptureId,
'refundReferenceId': uuidv4().toString().replace(/-/g, ''),
'amount': amount,
'sellerNote': 'Test note',
};
const response = newClient.refund(reqParam);
Below is an example on how to make the GetRefundDetails API call:
const reqParam = {
'amazonRefundId': amazonRefundId
};
const response = newClient.getRefundDetails(reqParam);
Below is an example on how to make the CreateOrderReferenceForId API call:
const reqParam = {
'id': amazonBillingAgreementId,
'amount': billingAmount,
'idType': 'BillingAgreement',
'storeName': storeName,
'sellerOrderId': sellerOrderId,
'sellerNote': sellerNote,
'customInformation': customInformation
};
const response = newClient.createOrderReferenceForId(reqParam);
Below is an example on how to make the GetMerchantAccountStatus API call:
const response = newClient.getMerchantAccountStatus(reqParam);
Below is an example on how to make the SetBillingAgreementDetails API call:
const reqParam = {
'amazonBillingAgreementId': amazonBillingAgreementId,
'storeName': storeName,
'sellerOrderId': sellerOrderId,
'sellerNote': sellerNote,
'customInformation': customInformation
};
const response = newClient.setBillingAgreementDetails(reqParam);
Below is an example on how to make the GetBillingAgreementDetails API call:
const reqParam = {
/** If you call this before ConfirmBillingAgreement,
* to get all the buyer address information you
* must pass 'accessToken'.
* After Confirm, 'accessToken' is not used.
**/
//'accessToken' : accessToken,
'amazonBillingAgreementId': amazonBillingAgreementId,
};
const response = newClient.getBillingAgreementDetails(reqParam);
Below is an example on how to make the ValidateBillingAgreement API call:
reqParam = {
'amazonBillingAgreementId': amazonBillingAgreementId,
};
const response = newClient.validateBillingAgreement(reqParam);
Below is an example on how to make the CloseBillingAgreement API call:
reqParam = {
'amazonBillingAgreementId': amazonBillingAgreementId,
};
const response = newClient.closeBillingAgreement(reqParam);
Below is an example on how to make the AuthorizeOnBillingAgreement API call:
/** For idempotency the AuthorizationReferenceId must be unique.
* To accomplish this we can generate a 32 character unique identifier
* using uuid/v4 https://www.npmjs.com/package/uuid and remove
* the dashes.
**/
const uuidv4 = require('uuid/v4');
reqParam = {
'amazonBillingAgreementId': amazonBillingAgreementId,
'authorizationReferenceId': uuidv4().toString().replace(/-/g, ''),
'amount': billingAmount,
'transactionTimeout': transactionTimeout,
'orderItemCategory': orderItemCategories,
'captureNow': captureNow,
'storeName': storeName,
'sellerOrderId': sellerOrderId,
'sellerNote': sellerNote,
'customInformation': customInformation
};
const response = newClient.authorizeOnBillingAgreement(reqParam);
Below is an example on how to make the GetUserInfo API call:
const accessToken = 'Atza|IwEBIAplM...';
const response = newClient.getUserInfo(accessToken);
The response will return a Promise containing a JSON object
response.then(function (result) {
console.log('User Info:');
console.log(result);
console.log('');
}).catch(err => {
console.log(err);
});
The response will return a Promise containing a XML string
// no additional processing
response.then(function (result) {
responseData = result;
console.log(responseData);
parseString(responseData, function (err, parseResult) {
parsedXML = parseResult;
const initialParse = JSON.parse(JSON.stringify(parsedXML));
if (initialParse.CreateOrderReferenceForIdResponse) {
jsonOutput = initialParse.CreateOrderReferenceForIdResponse.CreateOrderReferenceForIdResult[0];
const amazonCaptureIdRegion = (OrderReferenceDetails[0].AmazonOrderReferenceId[0]).toString();
console.log('CreateOrderReferenceForID response data:');
console.log(responseData);
console.log(`AmazonOrderReferenceId: ${amazonamazonOrderReferenceIdRegion}`);
}
});
}).catch(err => {
console.log(`Capture error data ${regionVal}:`);
console.log(err.body);
});
The response will return a Promise containing a JSON string
// Retrieve the AmazonOrderReferenceId from CreateOrderReferenceForId API response
response.then(function (result) {
responseData = result;
jsonOutput = JSON.parse(responseData).CreateOrderReferenceForIdResult;
const amazonOrderReferenceIdRegion = (jsonOutput.OrderReferenceDetails.AmazonOrderReferenceId).toString();
console.log('CreateOrderReferenceForID response data:');
console.log(responseData);
console.log(`AmazonOrderReferenceId: ${amazonamazonOrderReferenceIdRegion}`);
}).catch(err => {
console.log(err.body);
});
{"CreateOrderReferenceForIdResult":{"OrderReferenceDetails":{"OrderReferenceStatus":{"State":"Draft"},"ExpirationTimestamp":"2019-02-12T20:39:30.155Z","ParentDetails":{"Type":"BillingAgreement","Id":"C01-5824250-2443745"},"SellerOrderAttributes":{"StoreName":"This Store is Mine","CustomInformation":"custom stuff","SellerOrderId":"merchant_id:Test 4321"},"OrderTotal":{"CurrencyCode":"USD","Amount":"1.00"},"ReleaseEnvironment":"Sandbox","SellerNote":"This is a new note","AmazonOrderReferenceId":"S01-2810315-9040370","CreationTimestamp":"2018-08-16T20:39:30.155Z","RequestPaymentAuthorization":"false"}},"ResponseMetadata":{"RequestId":"b8337637-c45b-4b83-af75-f455d5eac8ca"}}
{ CreateOrderReferenceForIdResult: { OrderReferenceDetails: { OrderReferenceStatus: [Object], ExpirationTimestamp: '2019-02-21T03:43:10.305Z', ParentDetails: [Object], SellerOrderAttributes: [Object], OrderTotal: [Object], ReleaseEnvironment: 'Sandbox', SellerNote: 'This is a new note', AmazonOrderReferenceId: 'S01-8434721-2124216', CreationTimestamp: '2018-08-25T03:43:10.305Z', RequestPaymentAuthorization: 'false' } }, ResponseMetadata: { RequestId: 'd42adc19-c0ce-4aac-92b9-c84ce1653d1b' }, statusCode: 200 }
FAQs
Amazon Pay API Integration
We found that amazonpay demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.