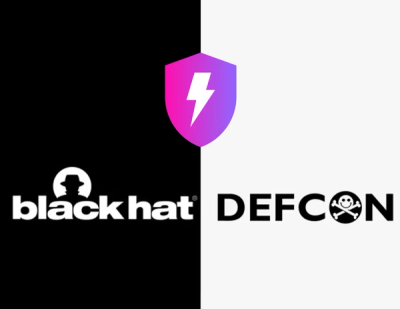
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
angular-soap
Advanced tools
An angular port of a Javascript SOAP Client into a factory that has a similar syntax to $http.
An Angular port of a JavaScript SOAP Client into a factory that has a similar syntax to $http.
Before using the factory, you must import the two scripts and its module:
<script src="soapclient.js"></script>
<script src="angular.soap.js"></script>
angular.module('myApp', ['angularSoap']);
Then, wherever you are going to consume it, make a reference to the $soap service for dependency injection:
.factory("testService", ['$soap',function($soap){
//use it here
}])
$soap has one method, post, which accepts the following paramaters:
Parameter | Description | Example |
---|---|---|
url | The base URL of the service | "http://www.cooldomain.com/SoapTest/webservicedemo.asmx" |
action | The action you want to call | "HelloWorld" |
params | An object of parameters to pass to the service | { name: "Andrew" } |
Syntax:
$soap.post(url,action,params);
Similar to $http methods, $soap.post returns a promise that you can act upon.
$soap.post(url,action,params).then(function(response){
//Do Stuff
});
NOTE: Response will be a javascript object containing the response mapped to objects. Do a console.log of response so you can see what you are working with.
A basic "Hello World" with no parameters.
angular.module('myApp', ['angularSoap'])
.factory("testService", ['$soap',function($soap){
var base_url = "http://www.cooldomain.com/SoapTest/webservicedemo.asmx";
return {
HelloWorld: function(){
return $soap.post(base_url,"HelloWorld");
}
}
}])
.controller('MainCtrl', function($scope, testService) {
testService.HelloWorld().then(function(response){
$scope.response = response;
});
})
A basic method call with parameters.
angular.module('myApp', ['angularSoap'])
.factory("testService", ['$soap',function($soap){
var base_url = "http://www.cooldomain.com/SoapTest/webservicedemo.asmx";
return {
CreateUser: function(firstName, lastName){
return $soap.post(base_url,"CreateUser", {firstName: firstName, lastName: lastName});
}
}
}])
.controller('MainCtrl', function($scope, testService) {
testService.CreateUser($scope.firstName, $scope.lastName).then(function(response){
$scope.response = response;
});
})
A basic method call to get a single object.
angular.module('myApp', ['angularSoap'])
.factory("testService", ['$soap',function($soap){
var base_url = "http://www.cooldomain.com/SoapTest/webservicedemo.asmx";
return {
GetUser: function(id){
return $soap.post(base_url,"GetUser", {id: id});
}
}
}])
.controller('MainCtrl', function($scope, testService) {
testService.GetUser($scope.id).then(function(user){
console.log(user.firstName);
console.log(user.lastName);
});
})
A basic method call to get a collection of objects.
angular.module('myApp', ['angularSoap'])
.factory("testService", ['$soap',function($soap){
var base_url = "http://www.cooldomain.com/SoapTest/webservicedemo.asmx";
return {
GetUsers: function(){
return $soap.post(base_url,"GetUsers");
}
}
}])
.controller('MainCtrl', function($scope, testService) {
testService.GetUsers().then(function(users){
for(i=0;i<users.length;i++){
console.log(users[i].firstName);
console.log(users[i].lastName);
}
});
})
Set the credentials for the connection before sending any requests.
angular.module('myApp', ['angularSoap'])
.factory("testService", ['$soap',function($soap){
var base_url = "http://www.cooldomain.com/SoapTest/webservicedemo.asmx";
$soap.setCredentials("username","password");
return {
GetUsers: function(){
return $soap.post(base_url,"GetUsers");
}
}
}])
.controller('MainCtrl', function($scope, testService) {
testService.GetUsers().then(function(users){
for(i=0;i<users.length;i++){
console.log(users[i].firstName);
console.log(users[i].lastName);
}
});
})
FAQs
An angular port of a Javascript SOAP Client into a factory that has a similar syntax to $http.
The npm package angular-soap receives a total of 38 weekly downloads. As such, angular-soap popularity was classified as not popular.
We found that angular-soap demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.