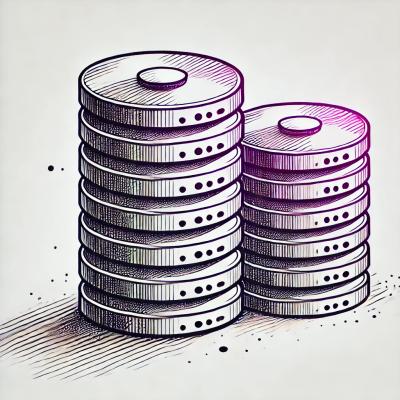
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
angular-spotify
Advanced tools
angular service to connect to the Spotify Web API
angular-spotify makes heavy use of promises throughout the service
Install angular-spotify via bower. Use the --save property to save into your bower.json file.
bower install angular-spotify --save
Also available on npm
npm install angular-spotify --save
Include spotify into your angular module
var app = angular.module('example', ['spotify']);
Most of the functions in Spotify do not require you to authenticate your application. However if you do need to gain access to playlists or a user's data then configure it like this:
app.config(function (SpotifyProvider) {
SpotifyProvider.setClientId('<CLIENT_ID>');
SpotifyProvider.setRedirectUri('<CALLBACK_URI>');
SpotifyProvider.setScope('<SCOPE>');
// If you already have an auth token
SpotifyProvider.setAuthToken('<AUTH_TOKEN>');
});
For example:
app.config(function (SpotifyProvider) {
SpotifyProvider.setClientId('ABC123DEF456GHI789JKL');
SpotifyProvider.setRedirectUri('http://www.example.com/callback.html');
SpotifyProvider.setScope('user-read-private playlist-read-private playlist-modify-private playlist-modify-public');
// If you already have an auth token
SpotifyProvider.setAuthToken('zoasliu1248sdfuiknuha7882iu4rnuwehifskmkiuwhjg23');
});
Inject Spotify into a controller to gain access to all the functions available
app.controller('MainCtrl', function (Spotify) {
});
Get Spotify catalog information for a single album.
Spotify.getAlbum('AlbumID or Spotify Album URI');
Example:
Spotify.getAlbum('0sNOF9WDwhWunNAHPD3Baj').then(function (data) {
console.log(data);
});
Get Spotify catalog information for multiple albums identified by their Spotify IDs.
Spotify.getAlbums('Array or comma separated list of Album IDs');
Example:
Spotify
.getAlbums('41MnTivkwTO3UUJ8DrqEJJ,6JWc4iAiJ9FjyK0B59ABb4,6UXCm6bOO4gFlDQZV5yL37')
.then(function (data) {
console.log(data);
});
Get Spotify catalog information about an album’s tracks. Optional parameters can be used to limit the number of tracks returned.
Spotify.getAlbumTracks('AlbumID or Spotify Album URI', options);
Example:
Spotify.getAlbumTracks('6akEvsycLGftJxYudPjmqK').then(function (data) {
console.log(data);
});
Get Spotify catalog information for a single artist identified by their unique Spotify ID or Spotify URI.
Spotify.getArtist('Artist Id or Spotify Artist URI');
Example
Spotify.getArtist('0LcJLqbBmaGUft1e9Mm8HV').then(function (data) {
console.log(data);
});
Get Spotify catalog information for several artists based on their Spotify IDs.
Spotify.getArtists('Comma separated string or array of Artist Ids');
Example:
Spotify
.getArtists('0oSGxfWSnnOXhD2fKuz2Gy,3dBVyJ7JuOMt4GE9607Qin')
.then(function (data) {
console.log(data);
});
Get Spotify catalog information about an artist’s albums. Optional parameters can be passed in to filter and sort the response.
Spotify.getArtistAlbums('Artist Id or Spotify Artist URI', options);
Example: { album_type: 'album,single' }
Example:
Spotify.getArtistAlbums('1vCWHaC5f2uS3yhpwWbIA6').then(function (data) {
console.log(data);
});
Get Spotify catalog information about an artist’s top tracks by country.
Spotify.getArtistTopTracks('Artist Id or Spotify Artist URI', 'Country Code');
Example:
Spotify
.getArtistTopTracks('1vCWHaC5f2uS3yhpwWbIA6', 'AU')
.then(function (data) {
console.log(data);
});
Get Spotify catalog information about artists similar to a given artist. Similarity is based on analysis of the Spotify community’s listening history.
Spotify.getRelatedArtists('Artist Id or Spotify Artist URI');
Example:
Spotify.getRelatedArtists('1vCWHaC5f2uS3yhpwWbIA6').then(function (data) {
console.log(data);
});
Discover new releases and featured playlists. User needs to be logged in to gain access to these features.
Get a list of Spotify featured playlists
Spotify.getFeaturedPlaylists(options);
Example:
Spotify
.getFeaturedPlaylists({ locale: "nl_NL", country: "NL" })
.then(function (data) {
console.log(data);
});
Get a list of new album releases featured in Spotify
Spotify.getNewReleases(options);
Example:
Spotify.getNewReleases({ country: "NL" }).then(function (data) {
console.log(data);
});
Get a list of categories used to tag items in Spotify (on, for example, the Spotify player’s “Browse” tab).
Spotify.getCategories(options);
limit
to get the next set of categories.Example:
Spotify.getCategories({ country: 'SG' }).then(function (data) {
console.log(data);
});
Get a single category used to tag items in Spotify (on, for example, the Spotify player’s “Browse” tab).
Spotify.getCategory(category_id, options);
Example:
Spotify.getCategory('party').then(function (data) {
console.log(data);
})
Get a list of Spotify playlists tagged with a particular category.
Spotify.getCategoryPlaylists(category_id, options);
limit
to get the next set of items.Example:
Spotify.getCategoryPlaylists('party').then(function (data) {
console.log(data);
})
Create a playlist-style listening experience based on seed artists, tracks and genres.
Spotify.getRecommendations(options);
Example:
Spotify.getRecommendations({ seed_artists: '4NHQUGzhtTLFvgF5SZesLK' }).then(function (data) {
console.log(data);
});
Retrieve a list of available genres seed parameter values for recommendations.
Spotify.getAvailableGenreSeeds();
Example:
Spotify.getAvailableGenreSeeds().then(function (data) {
console.log(data);
});
These endpoints allow you manage the list of artists and users that a logged in user follows. Following and unfollowing requires the user-follow-modify
scope. Check if Current User Follows requires the user-follow-read
scope.
Get the current user’s followed artists.
Spotify.following('type', options)
artist
is supported.Spotify.following('artists', { limit: 10 }).then(function (artists) {
console.log(artists);
})
Add the current user as a follower of one or more artists or other Spotify users.
Spotify.follow('type', 'ids');
artist
or user
Example:
Spotify.follow('user', 'exampleuser01').then(function () {
// no response from Spotify
});
Remove the current user as a follower of one or more artists or other Spotify users.
Spotify.unfollow('type', 'ids');
artist
or user
Example:
Spotify.unfollow('user', 'exampleuser01').then(function () {
// no response from Spotify
});
Check to see if the current user is following one or more artists or other Spotify users.
Spotify.userFollowingContains('type', 'ids');
artist
or user
Example:
Spotify.userFollowingContains('user', 'exampleuser01').then(function (data) {
console.log(data);
});
Add the current user as a follower of a playlist. Requires playlist-modify-public
or playlist-modify-private
scope to work.
Spotify.followPlaylist('owner_id', 'playlist_id', isPublic);
Example:
Spotify
.followPlaylist('jmperezperez', '2v3iNvBX8Ay1Gt2uXtUKUT', false)
.then(function (data) {
console.log(data);
});
Remove the current user as a follower of a playlist. Requires playlist-modify-public
or playlist-modify-private
scope to work.
Spotify.unfollowPlaylist('owner_id', 'playlist_id', isPublic);
Example:
Spotify
.unfollowPlaylist('jmperezperez', '2v3iNvBX8Ay1Gt2uXtUKUT')
.then(function (data) {
console.log(data);
});
Check to see if one or more Spotify users are following a specified playlist.Following a playlist can be done publicly or privately. Checking if a user publicly follows a playlist doesn't require any scopes; if the user is publicly following the playlist, this endpoint returns true.
Checking if the user is privately following a playlist is only possible for the current user when that user has granted access to the playlist-read-private
scope.
Spotify
.playlistFollowingContains('owner_id', 'playlist_id', 'comma separated string or array of user ids');
Example:
Spotify.playlistFollowingContains('jmperezperez', '2v3iNvBX8Ay1Gt2uXtUKUT', 'possan,elogain').then(function (data) {
console.log(data);
});
Get a list of the songs saved in the current Spotify user’s “Your Music” library. Requires the user-library-read
scope.
Spotify.getSavedUserTracks(options);
Spotify.getSavedUserTracks().then(function (data) {
console.log(data);
});
Check if one or more tracks is already saved in the current Spotify user’s “Your Music” library. Requires the user-library-read
scope.
Spotify.userTracksContains('comma separated string or array of spotify track ids');
Example:
Spotify
.userTracksContains('0udZHhCi7p1YzMlvI4fXoK,3SF5puV5eb6bgRSxBeMOk9')
.then(function (data) {
console.log(data);
});
Save one or more tracks to the current user’s “Your Music” library. Requires the user-library-modify
scope.
Spotify.saveUserTracks('comma separated string or array of spotify track ids');
Example:
Spotify
.saveUserTracks('0udZHhCi7p1YzMlvI4fXoK,3SF5puV5eb6bgRSxBeMOk9')
.then(function (data) {
console.log(data);
});
Remove one or more tracks from the current user’s “Your Music” library. Requires the user-library-modify
scope.
Spotify.removeUserTracks('comma separated string or array of spotify track ids');
Example:
Spotify
.removeUserTracks('0udZHhCi7p1YzMlvI4fXoK,3SF5puV5eb6bgRSxBeMOk9')
.then(function (data) {
console.log(data);
});
Save one or more albums to the current user’s “Your Music” library. Requires the user-library-modify
scope.
Spotify.saveUserAlbums('comma separated string or array of spotify album ids');
Example:
Spotify
.saveUserAlbums('4iV5W9uYEdYUVa79Axb7Rh,1301WleyT98MSxVHPZCA6M')
.then(function (data) {
console.log(data);
});
Get a list of the albums saved in the current Spotify user’s “Your Music” library. Requires the user-library-read
scope.
Spotify.getSavedUserAlbums(options);
Spotify.getSavedUserAlbums().then(function (data) {
console.log(data);
});
Remove one or more albums from the current user’s “Your Music” library. Requires the user-library-modify
scope.
Spotify.removeUserAlbums('comma separated string or array of spotify album ids');
Example:
Spotify
.removeUserAlbums('4iV5W9uYEdYUVa79Axb7Rh,1301WleyT98MSxVHPZCA6M')
.then(function (data) {
console.log(data);
});
Check if one or more albums is already saved in the current Spotify user’s “Your Music” library. Requires the user-library-read
scope.
Spotify.userAlbumsContains('comma separated string or array of spotify album ids');
Example:
Spotify
.userAlbumsContains('4iV5W9uYEdYUVa79Axb7Rh,1301WleyT98MSxVHPZCA6M')
.then(function (data) {
console.log(data);
});
Endpoints for retrieving information about the user’s listening habits.
Get the current user’s top artists based on calculated affinity.
Spotify.getUserTopArtists(options);
Example:
Spotify.getUserTopArtists({ limit: 50 }).then(function (data) {
console.log(data);
});
Get the current user’s top tracks based on calculated affinity.
Spotify.getUserTopTracks(options);
Example:
Spotify.getUserTopTracks({ limit: 50 }).then(function (data) {
console.log(data);
});
User needs to be logged in to gain access to playlists
Get a list of the playlists owned by a Spotify user. Requires the playlist-read-private
scope
Spotify.getUserPlaylists('user_id', options);
Example:
Spotify.getUserPlaylists('wizzler').then(function (data) {
console.log(data);
});
Get a playlist owned by a Spotify user.
Spotify.getPlaylist('user_id', 'playlist_id', options);
Spotify
.getPlaylist('1176458919', '6Df19VKaShrdWrAnHinwVO')
.then(function (data) {
console.log(data);
});
Get full details of the tracks of a playlist owned by a Spotify user. Requires the playlist-read-private
scope.
Spotify.getPlaylistTracks('user_id', 'playlist_id', options);
Example:
Spotify
.getPlaylistTracks('1176458919', '6Df19VKaShrdWrAnHinwVO')
.then(function (data) {
console.log(data);
});
Create a playlist for a Spotify user. (The playlist will be empty until you add tracks.) Creating a public playlist requires the playlist-modify-public
scope. Creating a private playlist requires the playlist-modify-private
scope.
Spotify.createPlaylist('user_id', options);
Example:
Spotify
.createPlaylist('1176458919', { name: 'Awesome Mix Vol. 1' })
.then(function (data) {
console.log('playlist created');
});
Add one or more tracks to a user’s playlist. Adding tracks to a public playlist requires the playlist-modify-public
scope. Adding tracks to a private playlist requires the playlist-modify-private
scope.
Spotify.addPlaylistTracks('user_id', 'playlist_id', 'comma separated string or array of spotify track uris');
Example:
Spotify
.addPlaylistTracks('1176458919', '2TkWjGCu8jurholsfdWtG4', 'spotify:track:4iV5W9uYEdYUVa79Axb7Rh, spotify:track:1301WleyT98MSxVHPZCA6M')
.then(function (data) {
console.log('tracks added to playlist');
});
Remove one or more tracks from a user’s playlist. Removing tracks from a public playlist requires the playlist-modify-public
scope. Removing tracks from a private playlist requires the playlist-modify-private
scope.
Spotify.removePlaylistTracks('user_id', 'playlist_id', 'comma separated string or array of spotify track ids or uris');
Example:
Spotify
.removePlaylistTracks('1176458919', '2TkWjGCu8jurholsfdWtG4', 'spotify:track:4iV5W9uYEdYUVa79Axb7Rh, spotify:track:1301WleyT98MSxVHPZCA6M')
.then(function (data) {
console.log('tracks removed from playlist');
});
Reorder a track or a group of tracks in a playlist.
Spotify.reorderPlaylistTracks('user_id', 'playlist_id', options);
Example:
Spotify.reorderPlaylistTracks('1176458919', '2TkWjGCu8jurholsfdWtG4', {
range_start: 8,
range_length: 5,
insert_before: 0
}).then(function (data) {
console.log(data);
});
Replace all the tracks in a playlist, overwriting its existing tracks. This powerful request can be useful for replacing tracks, re-ordering existing tracks, or clearing the playlist. Replacing tracks in a public playlist requires the playlist-modify-public
scope. Replacing tracks in a private playlist requires the playlist-modify-private
scope.
Spotify.replacePlaylistTracks('user_id', 'playlist_id', 'comma separated string or array of spotify track ids or uris');
Example:
Spotify
.replacePlaylistTracks('1176458919', '2TkWjGCu8jurholsfdWtG4', 'spotify:track:4iV5W9uYEdYUVa79Axb7Rh, spotify:track:1301WleyT98MSxVHPZCA6M')
.then(function (data) {
console.log('tracks removed from playlist');
});
Change a playlist’s name and public/private state. (The user must, of course, own the playlist.) Changing a public playlist requires the playlist-modify-public
scope. Changing a private playlist requires the playlist-modify-private
scope.
Spotify.updatePlaylistDetails('user_id', 'playlist_id', options);
Example:
Spotify
.updatePlaylistDetails('1176458919', '2TkWjGCu8jurholsfdWtG4', { name: 'Updated Playlist Title' })
.then(function (data) {
console.log('Updated playlist details');
});
User needs to be logged in to gain access to user profiles
Get public profile information about a Spotify user.
Spotify.getUser('user_id');
Example:
Spotify.getUser('wizzler').then(function (data) {
console.log(data);
});
Get detailed profile information about the current user (including the current user’s username).
Spotify.getCurrentUser();
Example:
Spotify.getCurrentUser().then(function (data) {
console.log(data);
});
Get Spotify catalog information about artists, albums, or tracks that match a keyword string.
Spotify.search('Search Query', 'type', options);
Example:
Spotify.search('Nirvana', 'artist').then(function (data) {
console.log(data);
});
Get Spotify catalog information for a single track identified by its unique Spotify ID or Spotify URI.
Spotify.getTrack('Track Id or Spotify Track URI');
Example:
Spotify.getTrack('0eGsygTp906u18L0Oimnem').then(function (data) {
console.log(data);
});
Get Spotify catalog information for multiple tracks based on their Spotify IDs.
Spotify.getTracks('Comma separated list or array of Track Ids');
Example:
Spotify.getTracks('0eGsygTp906u18L0Oimnem,1lDWb6b6ieDQ2xT7ewTC3G').then(function (data) {
console.log(data);
});
Get audio feature information for a single track identified by its unique Spotify ID.
Spotify.getTrackAudioFeatures('Track Id or Spotify Track URI');
Example:
Spotify.getTrackAudioFeatures('0eGsygTp906u18L0Oimnem').then(function (data) {
console.log(data);
});
Get audio features for multiple tracks based on their Spotify IDs.
Spotify.getTracksAudioFeatures('Comma separated list or array of Track Ids');
Example:
Spotify.getTracksAudioFeatures('0eGsygTp906u18L0Oimnem,1lDWb6b6ieDQ2xT7ewTC3G').then(function (data) {
console.log(data);
});
Will open login window. Requires user to initiate as it will open a pop up window. Requires client id, callback uri and scope to be set in config.
Spotify.login();
Example:
$scope.login = function () {
Spotify.login();
};
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title></title>
<script>
window.onload = function () {
var hash = window.location.hash;
if (window.location.search.substring(1).indexOf("error") !== -1) {
// login failure
window.close();
} else if (hash) {
// login success
var token = window.location.hash.split('&')[0].split('=')[1];
localStorage.setItem('spotify-token', token);
}
}
</script>
</head>
<body>
</body>
</html>
FAQs
Angular Service to connect to Spotify Web API
The npm package angular-spotify receives a total of 9 weekly downloads. As such, angular-spotify popularity was classified as not popular.
We found that angular-spotify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.