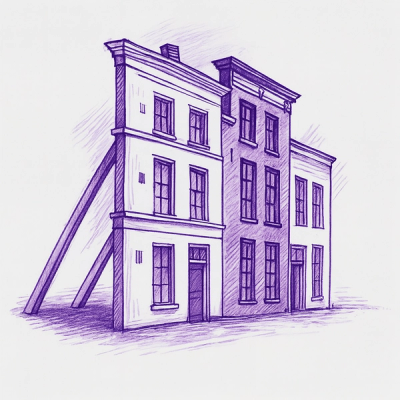
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
angular-tellform
Advanced tools
This module gives you the standalone front-end of tellform. It handles form rendering and form ui. For you to use this module you must have an API endpoint, such as FormKeep or Formlets.
<section class="content" ng-controller="MyFormCtrl">
<angular-tellform myform="form" endPoint="https://myurl.com/endpoint"></angular-tellform>
</section>
via bower
bower install angular-tellform
This serves as a quick guide to setting up and using angular-tellform in a small angular project. If you are looking for API documentation, please refer to the next section instead.
First add angular-tellform as a dependency to your angular module/app.
app.js
'use strict';
angular.module('myModule', ['angular-tellform']);
Set $scope.form in your controller to the JSON object of your form.
app.js
...
angular.module('myModule').controller('MyFormCtrl', function MyFormCtrl($scope) {
$scope.form = {
"title": "Job Application Example",
"design": {
"colors": {
"buttonTextColor": "#5C780A",
"buttonColor": "#C5F044",
"answerColor": "#333",
"questionColor": "#6D8524",
"backgroundColor": "#E4F8A8"
}
},
"hideFooter": false,
"startPage": {
"buttons": [],
"introButtonText": "Apply Now",
"introTitle": "We're looking for a great Growth Hacker",
"showStart": true
},
"form_fields": [{
"lastModified": "2016-04-22T23:02:46.017Z",
"title": "How would you rate your experience and knowledge of running split tests?",
"fieldType": "rating",
"fieldValue": 1,
"_id": "571940102aa1f3ff5e205b5d",
"validFieldTypes": ["textfield", "date", "email", "link", "legal", "url", "textarea", "statement", "welcome", "thankyou", "file", "dropdown", "scale", "rating", "radio", "checkbox", "hidden", "yes_no", "natural", "number"],
"deletePreserved": false,
"disabled": false,
"required": true,
"ratingOptions": {
"shape": "thumbs-up",
"steps": 5
},
"description": ""
}, {
"lastModified": "2016-04-22T23:02:46.017Z",
"title": "And how would you rate your creativity and ability to find innovative solutions?",
"fieldType": "rating",
"fieldValue": 1,
"_id": "571940222aa1f3ff5e205b5e",
"validFieldTypes": ["textfield", "date", "email", "link", "legal", "url", "textarea", "statement", "welcome", "thankyou", "file", "dropdown", "scale", "rating", "radio", "checkbox", "hidden", "yes_no", "natural", "number"],
"deletePreserved": false,
"disabled": false,
"required": true,
"ratingOptions": {
"shape": "thumbs-up",
"steps": 5
},
"description": ""
}],
"language": "english"
};
$scope.endPoint="https://myurl.com/endpoint";
});
Then add the angular-tellform directive to your controller's html view
view.html
...
<body ng-app="myModule">
<section class="content" ng-controller="MyFormCtrl">
<angular-tellform myform="form" endPoint="endPoint"></angular-tellform>
</section>
</body>
...
After this you will have a form displayed inside your section element. Since the directive is bounded to $scope.form, if you want to update the form all you need to do is change $scope.form to a new value.
A typical JSON object for form data will look like this:
{
"title": "Job Application Example",
"design": {
"colors": {
"buttonTextColor": "#5C780A",
"buttonColor": "#C5F044",
"answerColor": "#333",
"questionColor": "#6D8524",
"backgroundColor": "#E4F8A8"
}
},
"hideFooter": false,
"startPage": {
"buttons": [],
"introButtonText": "Apply Now",
"introTitle": "We're looking for a great Growth Hacker",
"showStart": true
},
"form_fields": [{
"lastModified": "2016-04-22T23:02:46.017Z",
"title": "How would you rate your experience and knowledge of running split tests?",
"fieldType": "rating",
"fieldValue": 1,
"_id": "571940102aa1f3ff5e205b5d",
"validFieldTypes": ["textfield", "date", "email", "link", "legal", "url", "textarea", "statement", "welcome", "thankyou", "file", "dropdown", "scale", "rating", "radio", "checkbox", "hidden", "yes_no", "natural", "number"],
"deletePreserved": false,
"disabled": false,
"required": true,
"ratingOptions": {
"shape": "thumbs-up",
"steps": 5
},
"description": ""
}, {
"lastModified": "2016-04-22T23:02:46.017Z",
"title": "And how would you rate your creativity and ability to find innovative solutions?",
"fieldType": "rating",
"fieldValue": 1,
"_id": "571940222aa1f3ff5e205b5e",
"validFieldTypes": ["textfield", "date", "email", "link", "legal", "url", "textarea", "statement", "welcome", "thankyou", "file", "dropdown", "scale", "rating", "radio", "checkbox", "hidden", "yes_no", "natural", "number"],
"deletePreserved": false,
"disabled": false,
"required": true,
"ratingOptions": {
"shape": "thumbs-up",
"steps": 5
},
"description": ""
}],
"language": "english"
Required
Type: String
The title of the form
Required
Type: Object
The object containing the CSS styles and colors for the form
####design.colors.buttonTextColor
**Required**
Type: `String`
The hex color of the button text
####design.colors.buttonColor
**Required**
Type: `String`
The hex color of the button background
####colors.anwserColor
**Required**
Type: `String`
The hex color of the answer (input field) text.
####design.colors.questionColor
**Required**
Type: `String`
The hex color of the question text.
####design.colors.backgroundColor
**Required**
Type: `String`
The hex color of the form background.
Recommended
Type: Boolean
If it is true, form footer will be hidden and vice-versa if it is false
Required
Type: Object
Stores form's startPage data
"startPage": {
"buttons": [], //Array of Buttons
"introButtonText": "Apply Now", //Continue button text
"introTitle": "We're looking for a great Growth Hacker", //Intro title
"showStart": true //Hides Start Page if false
}
Required
Type: Array
of Object
The array of form fields that your form will display.
Reccomended
Type: String
Specifies the language that your form will be rendered in
english
, spanish
, french
, german
or italian
english
if specifiedFirst clone the repository and run bower install
.
When you want to test your code, run
grunt
bower install
and then open the demo/index.html file for an example form.
When pushing submitting a PR, please make sure to delete your /dist directory.
Uses MIT License. See LICENSE.md for full license terms.
FAQs
Frontend UI for Tellform
The npm package angular-tellform receives a total of 7 weekly downloads. As such, angular-tellform popularity was classified as not popular.
We found that angular-tellform demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.