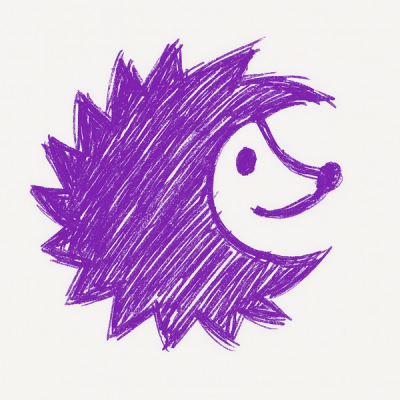
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
angular-youtube-api-factory
Advanced tools
angular-youtube-api-factory is an angularjs module with a youtube api factory.
Author: Jonathan Hornung (JohnnyTheTank)
bower install --save angular-youtube-api-factory
npm install --save angular-youtube-api-factory
jtt_youtube
to your application's module dependencies.<script src="bower_components/angular-youtube-api-factory/dist/angular-youtube-api-factory.min.js"></script>
<script src="node_modules/angular-youtube-api-factory/dist/angular-youtube-api-factory.min.js"></script>
<script src="angular-youtube-api-factory.min.js"></script>
youtubeFactory
via dependency injection//docs: https://developers.google.com/youtube/v3/docs/videos/list
youtubeFactory.getVideoById({
videoId: "<VIDEO_ID>",
part: "<YOUR_PART>", // (optional) default: 'id,snippet,contentDetails,statistics'
key: "<YOUR_API_KEY>",
}).then(function (_data) {
//on success
}).catch(function (_data) {
//on error
});
//docs: https://developers.google.com/youtube/v3/docs/channels/list
youtubeFactory.getVideosFromChannelById({
channelId: "<CHANNEL_ID>", // converter: http://johnnythetank.github.io/youtube-channel-name-converter/
q: "<SEARCH_STRING>", // (optional) search string
location: "<SEARCH_LOCATION>", // (optional) The parameter value is a string that specifies latitude/longitude coordinates e.g. '37.42307,-122.08427'.
locationRadius: "<LOCATION_RADIUS>", // (optional) valid values e.g. '1500m', '5km', '10000ft', and '0.75mi' | default: '5000m'
order: "<ORDER_TYPE>", // (optional) valid values: 'date', 'rating', 'relevance', 'title', 'videoCount', 'viewCount' | default: 'date'
maxResults: "<MAX_RESULTS>", // (optional) valid values: 0-50 | default: 5
publishedAfter: "<PUBLISHED_AFTER>", // (optional) RFC 3339 formatted date-time value (1970-01-01T00:00:00Z)
publishedBefore: "<PUBLISHED_AFTER>", // (optional) RFC 3339 formatted date-time value (1970-01-01T00:00:00Z)
regionCode: "<REGION_CODE>", // (optional) ISO 3166-1 alpha-2 country code
relevanceLanguage: "<RELEVANCE_LANGUAGE>", // (optional) ISO 639-1 two-letter language code
safeSearch: "<SAFE_SEARCH>", // (optional) valid values: 'moderate','none','strict' | defaut: 'moderate'
maxResults: "<MAX_RESULTS>", // (optional) valid values: 0-50 | default: 5
videoEmbeddable: "<VIDEO_EMBEDDABLE>", // (optional) valid values: 'true', 'any' | default: 'true'
videoLicense: "<VIDEO_LICENSE>", // (optional) valid values: 'any','creativeCommon','youtube'
videoSyndicated: "<VIDEO_SYNDICATED>", // (optional) restrict a search to only videos that can be played outside youtube.com. valid values: 'any','true' | default: 'any'
fields: "<FIELDS>", // (optional) Selector specifying which fields to include in a partial response
nextPageToken: "<NEXT__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
prevPageToken: "<PREV__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
part: "<PART>", // (optional) default: 'id,snippet'
key: "<YOUR_API_KEY>",
}).then(function (_data) {
//on success
}).catch(function (_data) {
//on error
});
//docs: https://developers.google.com/youtube/v3/docs/search/list
youtubeFactory.getVideosFromSearchByParams({
q: "<SEARCH_STRING>", // (optional) search string
location: "<SEARCH_LOCATION>", // (optional) The parameter value is a string that specifies latitude/longitude coordinates e.g. '37.42307,-122.08427'.
locationRadius: "<LOCATION_RADIUS>", // (optional) valid values e.g. '1500m', '5km', '10000ft', and '0.75mi' | default: '5000m'
order: "<ORDER_TYPE>", // (optional) valid values: 'date', 'rating', 'relevance', 'title', 'videoCount', 'viewCount' | default: 'date'
maxResults: "<MAX_RESULTS>", // (optional) valid values: 0-50 | default: 5
publishedAfter: "<PUBLISHED_AFTER>", // (optional) RFC 3339 formatted date-time value (1970-01-01T00:00:00Z)
publishedBefore: "<PUBLISHED_AFTER>", // (optional) RFC 3339 formatted date-time value (1970-01-01T00:00:00Z)
regionCode: "<REGION_CODE>", // (optional) ISO 3166-1 alpha-2 country code
relevanceLanguage: "<RELEVANCE_LANGUAGE>", // (optional) ISO 639-1 two-letter language code
safeSearch: "<SAFE_SEARCH>", // (optional) valid values: 'moderate','none','strict' | defaut: 'moderate'
maxResults: "<MAX_RESULTS>", // (optional) valid values: 0-50 | default: 5
videoEmbeddable: "<VIDEO_EMBEDDABLE>", // (optional) valid values: 'true', 'any' | default: 'true'
videoLicense: "<VIDEO_LICENSE>", // (optional) valid values: 'any','creativeCommon','youtube'
videoSyndicated: "<VIDEO_SYNDICATED>", // (optional) restrict a search to only videos that can be played outside youtube.com. valid values: 'any','true' | default: 'any'
fields: "<FIELDS>", // (optional) Selector specifying which fields to include in a partial response
nextPageToken: "<NEXT__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
prevPageToken: "<PREV__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
part: "<PART>", // (optional) default: 'id,snippet'
key: "<YOUR_API_KEY>",
}).then(function (_data) {
//on success
}).catch(function (_data) {
//on error
});
//docs: https://developers.google.com/youtube/v3/docs/playlists/list
youtubeFactory.getVideosFromPlaylistById({
playlistId: "<PLAYLIST_ID>",
maxResults: "<MAX_RESULTS>", // (optional) valid values: 0-50 // default: 5
nextPageToken: "<NEXT__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
prevPageToken: "<PREV__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
part: "<PART>", // (optional) default: 'id,snippet'
key: "<YOUR_API_KEY>",
}).then(function (_data) {
//on success
}).catch(function (_data) {
//on error
});
// docs: https://developers.google.com/youtube/v3/docs/search/list
youtubeFactory.getChannelById({
channelId: "<CHANNEL_ID>", // converter: http://johnnythetank.github.io/youtube-channel-name-converter/
nextPageToken: "<NEXT__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
prevPageToken: "<PREV__PAGE_TOKEN>", // (optional) either `nextPageToken` or `prevPageToken`
part: "<PART>", // (optional) default: 'id,snippet'
key: "<YOUR_API_KEY>",
}).then(function (_data) {
//on success
}).catch(function (_data) {
//on error
});
bandsintown - dailymotion - facebook - flickr - footballdata - github - openweathermap - tumblr - vimeo - wikipedia - youtube
MIT
FAQs
angularjs factory for youtube json rest api requests
The npm package angular-youtube-api-factory receives a total of 12 weekly downloads. As such, angular-youtube-api-factory popularity was classified as not popular.
We found that angular-youtube-api-factory demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.