announcekit-react
Advanced tools
Comparing version 2.1.3 to 2.1.4
@@ -26,14 +26,14 @@ import * as React from "react"; | ||
private widgetHandlers; | ||
constructor(props: any); | ||
shouldComponentUpdate(props: any): boolean; | ||
componentDidUpdate(prevProps: any): void; | ||
constructor(props: Props); | ||
shouldComponentUpdate(props: Props): boolean; | ||
componentDidUpdate(prevProps: Props): void; | ||
componentDidMount(): void; | ||
private loaded; | ||
private withWidget; | ||
open(): Promise<{}>; | ||
close(): Promise<{}>; | ||
instance(): Promise<{}>; | ||
unread(): Promise<{}>; | ||
open(): Promise<unknown>; | ||
close(): Promise<unknown>; | ||
instance(): Promise<unknown>; | ||
unread(): Promise<unknown>; | ||
render(): JSX.Element; | ||
} | ||
export {}; |
"use strict"; | ||
var __extends = (this && this.__extends) || (function () { | ||
var extendStatics = Object.setPrototypeOf || | ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || | ||
function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; }; | ||
var extendStatics = function (d, b) { | ||
extendStatics = Object.setPrototypeOf || | ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || | ||
function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; }; | ||
return extendStatics(d, b); | ||
}; | ||
return function (d, b) { | ||
@@ -12,15 +15,30 @@ extendStatics(d, b); | ||
})(); | ||
var __assign = (this && this.__assign) || Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
var __assign = (this && this.__assign) || function () { | ||
__assign = Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
var __createBinding = (this && this.__createBinding) || (Object.create ? (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
Object.defineProperty(o, k2, { enumerable: true, get: function() { return m[k]; } }); | ||
}) : (function(o, m, k, k2) { | ||
if (k2 === undefined) k2 = k; | ||
o[k2] = m[k]; | ||
})); | ||
var __setModuleDefault = (this && this.__setModuleDefault) || (Object.create ? (function(o, v) { | ||
Object.defineProperty(o, "default", { enumerable: true, value: v }); | ||
}) : function(o, v) { | ||
o["default"] = v; | ||
}); | ||
var __importStar = (this && this.__importStar) || function (mod) { | ||
if (mod && mod.__esModule) return mod; | ||
var result = {}; | ||
if (mod != null) for (var k in mod) if (Object.hasOwnProperty.call(mod, k)) result[k] = mod[k]; | ||
result["default"] = mod; | ||
if (mod != null) for (var k in mod) if (k !== "default" && Object.prototype.hasOwnProperty.call(mod, k)) __createBinding(result, mod, k); | ||
__setModuleDefault(result, mod); | ||
return result; | ||
@@ -38,5 +56,3 @@ }; | ||
var _this = _super.call(this, props) || this; | ||
_this.selector = ".ak-" + Math.random() | ||
.toString(36) | ||
.substring(10); | ||
_this.selector = ".ak-" + Math.random().toString(36).substring(10); | ||
_this.widgetHandlers = []; | ||
@@ -46,6 +62,26 @@ return _this; | ||
AnnounceKit.prototype.shouldComponentUpdate = function (props) { | ||
return !react_fast_compare_1.default(this.props, props); | ||
var oldProps = { | ||
data: this.props.data, | ||
user: this.props.user, | ||
lang: this.props.lang, | ||
}; | ||
var newProps = { | ||
data: props.data, | ||
user: props.user, | ||
lang: props.lang, | ||
}; | ||
return !react_fast_compare_1.default(oldProps, newProps); | ||
}; | ||
AnnounceKit.prototype.componentDidUpdate = function (prevProps) { | ||
if (!react_fast_compare_1.default(this.props, prevProps)) { | ||
var oldProps = { | ||
data: this.props.data, | ||
user: this.props.user, | ||
lang: this.props.lang, | ||
}; | ||
var newProps = { | ||
data: prevProps.data, | ||
user: prevProps.user, | ||
lang: prevProps.lang, | ||
}; | ||
if (!react_fast_compare_1.default(oldProps, newProps)) { | ||
if (this.widgetInstance) { | ||
@@ -58,2 +94,3 @@ this.widgetInstance.destroy(); | ||
AnnounceKit.prototype.componentDidMount = function () { | ||
var _a; | ||
if (!window["announcekit"]) { | ||
@@ -67,3 +104,3 @@ window["announcekit"] = window["announcekit"] || { | ||
window["announcekit"].queue.push([n, x]); | ||
} | ||
}, | ||
}; | ||
@@ -74,3 +111,3 @@ var scripttag = document.createElement("script"); | ||
var scr = document.getElementsByTagName("script")[0]; | ||
scr.parentNode.insertBefore(scripttag, scr); | ||
(_a = scr === null || scr === void 0 ? void 0 : scr.parentNode) === null || _a === void 0 ? void 0 : _a.insertBefore(scripttag, scr); | ||
} | ||
@@ -84,10 +121,10 @@ this.loaded(); | ||
badge: { | ||
style: style | ||
style: style, | ||
}, | ||
line: { | ||
style: style | ||
style: style, | ||
}, | ||
float: { | ||
style: style | ||
} | ||
style: style, | ||
}, | ||
}; | ||
@@ -99,6 +136,4 @@ if (this.props.floatWidget) { | ||
} | ||
this.name = Math.random() | ||
.toString(36) | ||
.substring(10); | ||
window["announcekit"].push(__assign({ widget: this.props.widget, name: this.name, version: 2, framework: "react", framework_version: "2.0.0", selector: this.selector, embed: this.props.embedWidget }, styleParams, { onInit: function (_widget) { | ||
this.name = Math.random().toString(36).substring(10); | ||
window["announcekit"].push(__assign(__assign({ widget: this.props.widget, name: this.name, version: 2, framework: "react", framework_version: "2.0.0", selector: this.selector, embed: this.props.embedWidget }, styleParams), { onInit: function (_widget) { | ||
if (_widget.conf.name !== _this.name) { | ||
@@ -105,0 +140,0 @@ return _widget.destroy(); |
{ | ||
"name": "announcekit-react", | ||
"version": "2.1.3", | ||
"version": "2.1.4", | ||
"description": "Use AnnounceKit widgets in your React App", | ||
@@ -8,3 +8,4 @@ "main": "dist/index.js", | ||
"scripts": { | ||
"build": "tsc" | ||
"build": "tsc", | ||
"postinstall": "husky install" | ||
}, | ||
@@ -23,3 +24,6 @@ "repository": "https://github.com/announcekitapp/announcekit-react", | ||
"@types/react": ">=15.0.0", | ||
"typescript": "^2.7.2" | ||
"husky": "^5.0.9", | ||
"prettier": "^2.2.1", | ||
"pretty-quick": "^3.1.0", | ||
"typescript": "^4.1.3" | ||
}, | ||
@@ -26,0 +30,0 @@ "peerDependencies": { |
@@ -34,3 +34,3 @@ 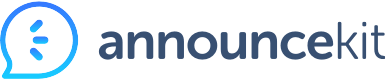 | ||
<AnnounceKit widget="https://announcekit.app/widgets/v2/34MmKA"> | ||
<span>What's new</span> | ||
<span>Whats new</span> | ||
</AnnounceKit> | ||
@@ -65,7 +65,6 @@ </li> | ||
- `open()` | ||
- `close()` | ||
- `close()` | ||
- `unread()` | ||
- `instance()` | ||
```js | ||
@@ -78,3 +77,3 @@ function App() { | ||
}); | ||
return ( | ||
@@ -86,3 +85,3 @@ <div> | ||
<AnnounceKit ref={ref} widget="https://announcekit.app/widgets/v2/3739N6"> | ||
<span>What's new</span> | ||
<span>Whats new</span> | ||
</AnnounceKit> | ||
@@ -93,3 +92,1 @@ </div> | ||
``` | ||
Sorry, the diff of this file is not supported yet
Install scripts
Supply chain riskInstall scripts are run when the package is installed. The majority of malware in npm is hidden in install scripts.
Found 1 instance in 1 package
18406
8
430
5
88
1