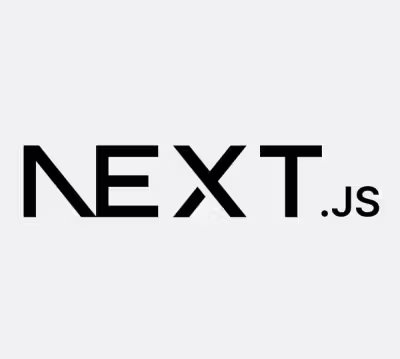
Security News
Next.js Patches Critical Middleware Vulnerability (CVE-2025-29927)
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
api-examples
Advanced tools
Common utils for api examples
Add to your package.json
npm install api-examples
We lean on convention to minimise code.
New up ApiExamples with your service name.
Then when you want to produce an api example from an e2e test:
This will write a request and response json output to:
__apiExamples/${serviceName}/${example-name}
For example the below:
it('saves hotel config', async () => {
const postResponse = await request.post(`${baseUrl}/hotels`, {
json: { apiKey: 'hotel api key', client: 'hotel client' },
resolveWithFullResponse: true,
});
expect(postResponse.statusCode).toBe(201);
apiExamples.save('hotel-config', postResponse.request, postResponse.request.response); // [1]
});
will publish to a file called __apiExamples/figgy/hotel-config.json
:
{
"request": {
"method": "POST",
"path": "/hotels"
},
"response": {
"statusCode": 201,
"statusMessage": "Created",
"headers": {
"content-type": "application/json; charset=utf-8",
"content-length": "50",
"date": "Thu, 07 Jun 2018 16:08:11 GMT",
"connection": "close"
},
"body": {
"apiKey": "hotel api key",
"client": "hotel client"
}
}
}
As part of your build you rsync the folder to gcp bucket api-examples:
gsutil -m rsync -cr __apiExamples gs://api-examples/
New up ApiExamples with the service name you're testing against.
You can then pull api examples from a gcloud bucket called api-examples. [1]
Then match against the examples to get a response [2]
You can match on:
method
path
body
Then we can assert on the response [3]
const ApiExamples = require('api-examples');
const apiExamples = new ApiExamples('figgy');
const expect = require('expect.js');
describe('figgy', ()=>{
before(async function() {
this.timeout(10000);
await apiExamples.pullApiExamples(); // [1] <--
});
it('gives us a hotel from client and property id', async () => {
const response = await apiExamples.findMatchingExample({ // [2] <--
method: 'POST'
});
expect(response.statusCode).to.be(201); // [3] <--
})
});
FAQs
Common utils for api examples
The npm package api-examples receives a total of 2 weekly downloads. As such, api-examples popularity was classified as not popular.
We found that api-examples demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Next.js has patched a critical vulnerability (CVE-2025-29927) that allowed attackers to bypass middleware-based authorization checks in self-hosted apps.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.