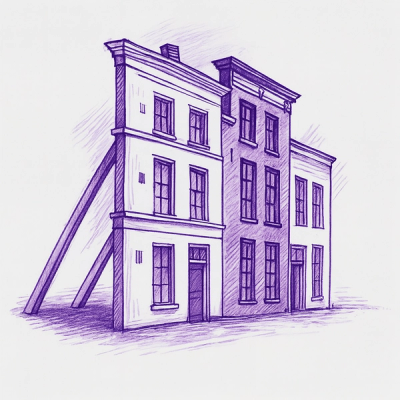
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
A collection of APIs for various media downloads, including TikTok, Twitter, and more. Provides easy access to download HD Wallpapers, mod apks, and metadata from various platforms.
> npm i api-qasim
Or
> yarn add api-qasim
Import In Common js
const Qasim = require('api-qasim');
Import In ESM Module
import pkg from 'api-qasim';
const { ringtone } = pkg;
const Qasim = require('api-qasim');
(async () => {
// === Google Image ===
const searchQuery = "cat"; // Example search query
let googleImageResponse = await Qasim.googleImage(searchQuery); // Fetch image URLs for the search query
console.log('Google Image Search Results:', googleImageResponse); // Log image URLs
// === GitHub Clone ===
const gitUrl = "https://github.com/GlobalTechInfo/ULTRA-MD"; // Example GitHub URL
let gitcloneResponse = await Qasim.gitclone(gitUrl); // Fetch GitHub clone data
console.log('GitHub Clone Data:', gitcloneResponse); // Log GitHub clone response
// === Facebook Usage ===
const fbtext = "Facebook Url";
let fbResponse = await Qasim.fbdl(fbtext);
let fbData = fbResponse.data;
console.log('Facebook Data:', fbData);
// === Instagram Usage ===
const instatext = "Instagram Url";
let igResponse = await Qasim.igdl(instatext);
let igData = igResponse.data;
console.log('Instagram Data:', igData);
// === Mediafire Dl ===
const mediafireUrl = "Mediafire Url";
let mediafireResponse = await Qasim.mediafire(mediafireUrl);
let mediafireData = mediafireResponse;
console.log('MediaFire Data:', mediafireData);
} catch (error) {
console.error('Error:', error);
}
}
();
const Qasim = require('api-qasim');
(async () => {
try {
// === Ringtones Dl ===
const ringtoneResult = await Qasim.ringtone('Nokia');
console.log('Ringtones:', ringtoneResult);
// === Apk Search ===
const apksearchResult = await Qasim.apksearch('Telegram');
console.log('Android1 APK:', apksearchResult);
// === Weather Info ===
const weatherResult = await Qasim.weather('Karachi');
console.log('Weather of Karachi:', weatherResult);
} catch (error) {
console.error('Error:', error);
}
})();
Usage Islamic Features
const Qasim = require('api-qasim');
// Use any of the functions, for example, NiatAshar:
Qasim.NiatAshar().then(result => {
console.log(result);
}).catch(error => {
console.error('Error:', error);
});
Islamic Features
Qasim.Tahlil();
Qasim.Wirid();
Qasim.AyatKursi();
Qasim.DoaHarian();
Qasim.NiatSalaht();
Qasim.NiatFajar();
Qasim.NiatZuhur();
Qasim.NiatAshar();
Qasim.NiatMaghrib();
Qasim.NiatIsha();
Qasim.BacaanSalaht();
Qasim.AsmaulHusna();
Qasim.QisaNabi();
Downloads Features
Qasim.ytsearch('query') // search query for YouTube
Qasim.ytmp4('url') // YouTube Video Url
Qasim.ytmp3('url') // url of YouTube video/audio
Qasim.googleImage('query'); // search query for downloading image from google
Qasim.gitclone('git url'); // Some Github Repository Url
Qasim.igdl('Instagram url'); // Instagram Post Url, e.g link of reel,image, video etc.
Qasim.fbdl('facebook url'); // Facebook Post Url e.g reel, image, video etc.
Qasim.mediafire('mediafire url'); // url of some file on mediafire
Qasim.wallpapercraft('query'); // wallpaper search query e.g 'sky'
Qasim.wallpaper('query'); //wallpapers search query e.g 'technology'
Qasim.ringtone('title'); // title of the ringtone that you wana search e.g 'Shape of You'
Qasim.mediaumma('url'); // some media url from mediaumma website
Qasim.wikimedia('query'); // search query for images from Wikimedia e.g 'Laptop'
Qasim.tiktokDl('url'); // url of tiktok media, complete tiktok scraper
Qasim.xdown('url'); // url of twitter media, complete twitter scraper
Qasim.stickersearch('query'); // query for sticker search e.g 'Babar Azam'
Qasim.facebook('url'); // works well with reels
Qasim.pinterest('query'); // image search query e.g 'Electronics'
Qasim.Pinterest2('query'); // image search query e.g 'Cat'
Qasim.zerochan('query'); // some anime name e.g 'itachi'
Qasim.cariresep('url'); // Food Recipes web url e.g https://resepkoki.id/resep-nasi-daun-jeruk-praktis-untuk-menu-sehari-hari
Qasim.webtoons('query'); // some search query e.g 'ignite'
Stalk Features
Qasim.githubStalk('user') // Github Username e.g 'GlobalTechInfo'
Qasim.tiktokStalk('user') // TikTok Username e.g. 'discoverpakistantv'
Qasim.freefireStalk('userId') // Free Fire User Id
Qasim.igStalk('userName') // Instagram Username e.g 'truepakistanofficial'
Qasim.npmStalk('query') // npm package name e.g 'api-qasim'
Some Tools
Qasim.konachan('query'); // anime search query e.g 'neko'
Qasim.styletext('teks'); // text that you wana convert in various styles e.g 'hello'
Qasim.trendtwit('country'); // Trending Twitter Tags. use Country e.g 'Pakistan'
Qasim.bitly('url'); // url that you wana convert/shorten to bit.ly
Qasim.ssweb('url'); // url of the page from where you wana get screenshot
Qasim.gempa(); // Earthquake info only for Indonesia
Qasim.tinyurl('url'); // Link shortener , url that you wana shorten
Apk Search
Qasim.playstore('search'); // some apk search query e.g 'whatsapp'
Qasim.apkmirror('querry'); // some apk search query e.g 'whatsapp'
Qasim.apksearch('query'); // some apk search query e.g 'facebook'
Qasim.happymod('query'); // some apk search query e.g 'Telegram'
Information
Qasim.weather('city'); // weather info query e.g 'Lahore'
Qasim.mangatoon('search'); // some anime name e.g 'nezoku'
Qasim.quotesanime();
Qasim.artinama('query'); // some name for its meaning
Qasim.wattpad('query'); // wattpad search query e.g 'japan'
Qasim.wikisearch('query'); // wikipedia search query e.g 'heroku'
Random Wallpapers
Qasim.Game();
Qasim.Technology();
Qasim.Programming();
Qasim.Mountain();
Qasim.Islamic();
Qasim.CyberSpace();
Ā© GlobalTechInfo 2025
FAQs
A collection of APIs for various media downloads, including TikTok, Twitter, and more. Provides easy access to download HD Wallpapers, mod apks, and metadata from various platforms.
The npm package api-qasim receives a total of 143 weekly downloads. As such, api-qasim popularity was classified as not popular.
We found that api-qasim demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Ā It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.