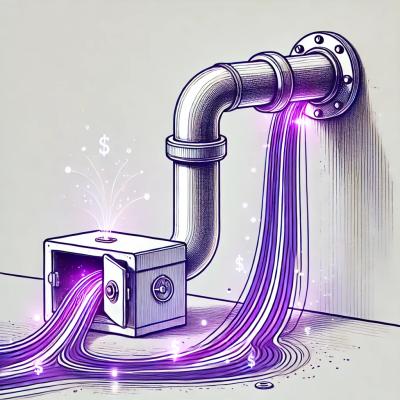
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
apollo-link-error
Advanced tools
The apollo-link-error package is used in Apollo Client to handle GraphQL errors. It allows developers to intercept and respond to errors that occur during GraphQL operations, such as network errors or GraphQL errors returned by the server.
Error Handling
This feature allows you to handle both GraphQL and network errors. The onError function takes a callback that receives an object containing graphQLErrors and networkError. You can then log or handle these errors as needed.
const { onError } = require('apollo-link-error');
const errorLink = onError(({ graphQLErrors, networkError }) => {
if (graphQLErrors) {
graphQLErrors.forEach(({ message, locations, path }) => {
console.log(`GraphQL error: Message: ${message}, Location: ${locations}, Path: ${path}`);
});
}
if (networkError) {
console.log(`Network error: ${networkError}`);
}
});
Retry Logic
This feature allows you to implement retry logic for network errors. If a network error occurs, the operation is retried after a specified delay (e.g., 3000 milliseconds).
const { onError } = require('apollo-link-error');
const { ApolloLink, Observable } = require('apollo-link');
const retryLink = onError(({ networkError, operation, forward }) => {
if (networkError) {
return new Observable(observer => {
setTimeout(() => {
forward(operation).subscribe({
next: observer.next.bind(observer),
error: observer.error.bind(observer),
complete: observer.complete.bind(observer),
});
}, 3000);
});
}
});
Custom Error Handling
This feature allows you to customize error handling based on specific error messages or types. For example, you can handle 'UNAUTHENTICATED' errors differently by logging a specific message or taking other actions.
const { onError } = require('apollo-link-error');
const customErrorLink = onError(({ response, operation }) => {
if (response.errors) {
response.errors = response.errors.map(error => {
if (error.message === 'UNAUTHENTICATED') {
// Custom handling for unauthenticated errors
console.log('User is unauthenticated');
}
return error;
});
}
});
The apollo-link-retry package provides retry logic for failed GraphQL operations. It allows you to specify retry policies, such as the number of retries and the delay between retries. Unlike apollo-link-error, it focuses specifically on retrying failed operations rather than handling errors in general.
The apollo-link-context package allows you to set context for your GraphQL operations, such as adding authentication tokens or other headers. While it doesn't handle errors directly, it can be used in conjunction with apollo-link-error to manage authentication-related errors.
The apollo-link-logger package logs the details of GraphQL operations and their results. It can be useful for debugging and monitoring, but it doesn't provide error handling capabilities like apollo-link-error.
Use this link to do some custom logic when a GraphQL or network error happens:
import { onError } from "apollo-link-error";
const link = onError(({ graphQLErrors, networkError }) => {
if (graphQLErrors)
graphQLErrors.forEach(({ message, locations, path }) =>
console.log(
`[GraphQL error]: Message: ${message}, Location: ${locations}, Path: ${path}`
)
);
if (networkError) console.log(`[Network error]: ${networkError}`);
});
Apollo Link is a system of modular components for GraphQL networking. Read the docs to learn how to use this link with libraries like Apollo Client and graphql-tools, or as a standalone client.
Error Link takes a function that is called in the event of an error. This function is called with an object containing the following keys:
operation
: The Operation that erroredresponse
: The result returned from lower down in the link chaingraphQLErrors
: An array of errors from the GraphQL endpointnetworkError
: Any error during the link execution or server response, that wasn't delivered as part of the errors
field in the GraphQL resultforward
: A reference to the next link in the chain. Calling return forward(operation)
in the callback will retry the request, returning a new observable for the upstream link to subscribe to.Returns: Observable<FetchResult> | void
The error callback can optionally return an observable from calling forward(operation)
if it wants to retry the request. It should not return anything else.
An error is passed as a networkError
if a link further down the chain called the error
callback on the observable. In most cases, graphQLErrors
is the errors
field of the result from the last next
call.
A networkError
can contain additional fields, such as a GraphQL object in the case of a failing HTTP status code from apollo-link-http
. In this situation, graphQLErrors
is an alias for networkError.result.errors
if the property exists.
An error handler might want to do more than just logging errors. You can check for a certain failure condition or error code, and retry the request if rectifying the error is possible. For example, when using some form of token based authentication, there is a need to handle re-authentication when the token expires. Here is an example of how to do this using forward()
.
onError(({ graphQLErrors, networkError, operation, forward }) => {
if (graphQLErrors) {
for (let err of graphQLErrors) {
switch (err.extensions.code) {
case 'UNAUTHENTICATED':
// error code is set to UNAUTHENTICATED
// when AuthenticationError thrown in resolver
// modify the operation context with a new token
const oldHeaders = operation.getContext().headers;
operation.setContext({
headers: {
...oldHeaders,
authorization: getNewToken(),
},
});
// retry the request, returning the new observable
return forward(operation);
}
}
}
if (networkError) {
console.log(`[Network error]: ${networkError}`);
// if you would also like to retry automatically on
// network errors, we recommend that you use
// apollo-link-retry
}
}
);
Here is a diagram of how the request flow looks like now:
One caveat is that the errors from the new response from retrying the request does not get passed into the error handler again. This helps to avoid being trapped in an endless request loop when you call forward() in your error handler.
If you want to conditionally ignore errors, you can set response.errors = undefined;
within the error handler:
onError(({ response, operation }) => {
if (operation.operationName === "IgnoreErrorsQuery") {
response.errors = undefined;
}
});
FAQs
Error Apollo Link for GraphQL Network Stack
The npm package apollo-link-error receives a total of 322,150 weekly downloads. As such, apollo-link-error popularity was classified as popular.
We found that apollo-link-error demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.