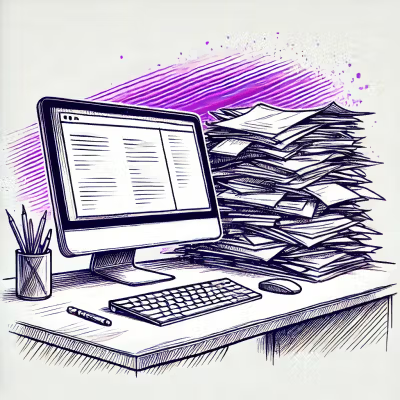
Security News
High Salaries No Longer Enough to Attract Top Cybersecurity Talent
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Wrap a filesystem to provide a read-only fs
api that adapts files according to an active flagset
new AdaptiveFS(options);
options.fs
(optional): a filesystem that conforms to the node.js fs
api. Defaults to the built-in fs
moduleoptions.flags
(required): an Object
where each key represents a flag and the value is a boolean indicating whether that flag is active or Array
of strings that represent the active flagsresolveSync(path)
Returns the resolved path based on the active flagset
getMatchesSync(path)
Returns a MatchSet
for the path
isAdaptiveSync(path)
Returns a boolean indicating if the path can be adapted
clearCache()
Clears the adaptive resolver's cache
fs
stat
statSync
readdir
readdirSync
readFile
readFileSync
readlink
readlinkSync
exists
existsSync
import fs from 'fs';
import AdaptiveFS from 'arc-fs';
const afs = new AdaptiveFS({ flags:['test'] });
fs.writeFileSync('message[test].txt', 'Hello Test');
afs.readFileSync('message.txt'); // Hello Test
import MemoryFS from 'memory-fs';
import AdaptiveFS from 'arc-fs';
const mfs = new MemoryFS();
const afs = new AdaptiveFS({ fs:mfs, flags:['test'] });
mfs.writeFileSync('message[test].txt', 'Hello Test');
afs.readFileSync('message.txt'); // Hello Test
FAQs
<img src="https://img.shields.io/github/license/eBay/ar
The npm package arc-fs receives a total of 52 weekly downloads. As such, arc-fs popularity was classified as not popular.
We found that arc-fs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.
Security News
Corepack will be phased out from future Node.js releases following a TSC vote.