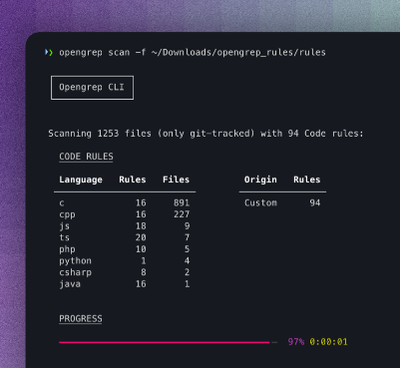
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
are-we-there-yet
Advanced tools
Keep track of the overall completion of many disparate processes
The 'are-we-there-yet' npm package is a progress tracker for complex operations where multiple steps are involved. It can be used to track the progress of individual items and groups of items, and it can report on the overall progress of the entire set of operations.
Tracking individual items
This feature allows you to track the progress of individual items by creating a new Tracker instance and specifying the total work units. You can then mark work as completed and check the progress.
{"var Tracker = require('are-we-there-yet').Tracker; var item = new Tracker('item', 10); item.completeWork(1); console.log(item.completed()); // Output: 1"}
Tracking groups of items
This feature allows you to track the progress of groups of items. You can create a new TrackerGroup instance, add items to the group, and then mark work as completed on individual items. The group's overall progress is calculated based on the progress of all items.
{"var TrackerGroup = require('are-we-there-yet').TrackerGroup; var group = new TrackerGroup('group'); var item1 = group.newItem('item1', 10); var item2 = group.newItem('item2', 20); item1.completeWork(5); item2.completeWork(10); console.log(group.completed()); // Output: 0.5"}
Reporting overall progress
This feature allows you to report the overall progress of the entire set of operations. You can add units of work to a TrackerGroup and mark them as completed. The group's completed method will return the overall progress as a fraction of the total work.
{"var TrackerGroup = require('are-we-there-yet').TrackerGroup; var group = new TrackerGroup('group'); group.addUnit('item1', 10); group.addUnit('item2', 20); group.completeUnit('item1'); group.completeUnit('item2', 10); console.log(group.completed()); // Output: 0.5"}
The 'progress' package provides a simple way to create a text-based progress bar in the console. It is similar to 'are-we-there-yet' in that it tracks the progress of operations, but it does not provide the same level of granularity for tracking groups and individual items.
The 'cli-progress' package is another tool for creating customizable progress bars in the command line interface. It offers a variety of options for styling and updating progress bars but does not include the hierarchical tracking features found in 'are-we-there-yet'.
The 'nanobar' package is designed for creating lightweight progress bars on web pages. While it serves a similar purpose in tracking progress visually, it is intended for client-side use in web applications rather than for Node.js command-line applications like 'are-we-there-yet'.
Track complex hierarchies of asynchronous task completion statuses. This is intended to give you a way of recording and reporting the progress of the big recursive fan-out and gather type workflows that are so common in async.
What you do with this completion data is up to you, but the most common use case is to feed it to one of the many progress bar modules.
Most progress bar modules include a rudimentary version of this, but my needs were more complex.
var TrackerGroup = require("are-we-there-yet").TrackerGroup
var top = new TrackerGroup("program")
var single = top.newItem("one thing", 100)
single.completeWork(20)
console.log(top.completed()) // 0.2
fs.stat("file", function(er, stat) {
if (er) throw er
var stream = top.newStream("file", stat.size)
console.log(top.completed()) // now 0.1 as single is 50% of the job and is 20% complete
// and 50% * 20% == 10%
fs.createReadStream("file").pipe(stream).on("data", function (chunk) {
// do stuff with chunk
})
top.on("change", function (name) {
// called each time a chunk is read from "file"
// top.completed() will start at 0.1 and fill up to 0.6 as the file is read
})
})
Implemented in: Tracker
, TrackerGroup
, TrackerStream
Returns the ratio of completed work to work to be done. Range of 0 to 1.
Implemented in: Tracker
, TrackerGroup
Marks the tracker as completed. With a TrackerGroup this marks all of its components as completed.
Marks all of the components of this tracker as finished, which in turn means
that tracker.completed()
for this will now be 1.
This will result in one or more change
events being emitted.
All tracker objects emit change
events with the following arguments:
function (name, completed, tracker)
name
is the name of the tracker that originally emitted the event,
or if it didn't have one, the first containing tracker group that had one.
completed
is the percent complete (as returned by tracker.completed()
method).
tracker
is the tracker object that you are listening for events on.
var tracker = new TrackerGroup(name)
Creates a new empty tracker aggregation group. These are trackers whose completion status is determined by the completion status of other trackers added to this aggregation group.
Ex.
var tracker = new TrackerGroup("parent")
var foo = tracker.newItem("firstChild", 100)
var bar = tracker.newItem("secondChild", 100)
foo.finish()
console.log(tracker.completed()) // 0.5
bar.finish()
console.log(tracker.completed()) // 1
tracker.addUnit(otherTracker, weight)
Adds the otherTracker to this aggregation group. The weight determines how long you expect this tracker to take to complete in proportion to other units. So for instance, if you add one tracker with a weight of 1 and another with a weight of 2, you're saying the second will take twice as long to complete as the first. As such, the first will account for 33% of the completion of this tracker and the second will account for the other 67%.
Returns otherTracker.
The above is exactly equivalent to:
var subGroup = tracker.addUnit(new TrackerGroup(name), weight)
The above is exactly equivalent to:
var subItem = tracker.addUnit(new Tracker(name, todo), weight)
The above is exactly equivalent to:
var subStream = tracker.addUnit(new TrackerStream(name, todo), weight)
Returns a tree showing the completion of this tracker group and all of its children, including recursively entering all of the children.
var tracker = new Tracker(name, todo)
Ordinarily these are constructed as a part of a tracker group (via
newItem
).
Returns the ratio of completed work to work to be done. Range of 0 to 1. If total work to be done is 0 then it will return 0.
tracker.addWork(todo)
Increases the amount of work to be done, thus decreasing the completion
percentage. Triggers a change
event.
tracker.completeWork(completed)
Increase the amount of work complete, thus increasing the completion percentage.
Will never increase the work completed past the amount of work todo. That is,
percentages > 100% are not allowed. Triggers a change
event.
Marks this tracker as finished, tracker.completed() will now be 1. Triggers
a change
event.
var tracker = new TrackerStream(name, size, options)
The tracker stream object is a pass through stream that updates an internal tracker object each time a block passes through. It's intended to track downloads, file extraction and other related activities. You use it by piping your data source into it and then using it as your data source.
If your data has a length attribute then that's used as the amount of work completed when the chunk is passed through. If it does not (eg, object streams) then each chunk counts as completing 1 unit of work, so your size should be the total number of objects being streamed.
tracker.addWork(todo)
Increases the amount of work to be done, thus decreasing the completion
percentage. Triggers a change
event.
FAQs
Keep track of the overall completion of many disparate processes
The npm package are-we-there-yet receives a total of 11,512,420 weekly downloads. As such, are-we-there-yet popularity was classified as popular.
We found that are-we-there-yet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.