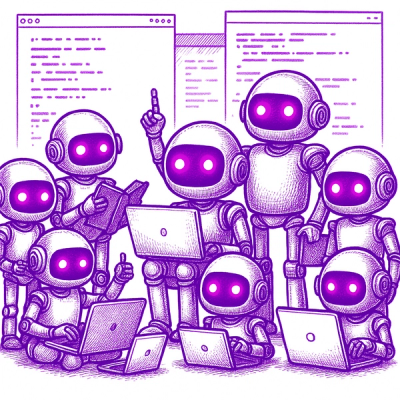
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
ES6 Array.find ponyfill. Return the first array element which satisfies a testing function.
The array-find npm package provides a simple utility to find the first element in an array that satisfies a provided testing function. It is a lightweight alternative to the native Array.prototype.find method, offering similar functionality.
Finding an element in an array
This feature allows you to find the first element in an array that satisfies a given condition. In this example, the condition is that the element should be greater than 3.
const find = require('array-find');
const array = [1, 2, 3, 4, 5];
const found = find(array, function(element) {
return element > 3;
});
console.log(found); // Output: 4
Lodash is a modern JavaScript utility library delivering modularity, performance, and extras. It includes a `find` method that works similarly to array-find but offers a broader range of utility functions for working with arrays, objects, and other data types.
Underscore is a JavaScript library that provides a whole mess of useful functional programming helpers without extending any built-in objects. It includes a `find` method that is similar to array-find but is part of a larger suite of utility functions.
Ramda is a practical functional library for JavaScript programmers. It makes it easy to create functional pipelines and includes a `find` function that works similarly to array-find but is designed with a functional programming approach in mind.
ES 2015 Array.find
ponyfill.
Ponyfill: A polyfill that doesn't overwrite the native method.
Find array elements. Executes a callback for each element, returns the first element for which its callback returns a truthy value.
var find = require('array-find');
var numbers = [1, 2, 3, 4];
find(numbers, function (element, index, array) {
return element === 2;
});
// => 2
var robots = [{name: 'Flexo'}, {name: 'Bender'}, {name: 'Buster'}];
find(robots, function (robot, index, array) {
return robot.name === 'Bender';
});
// => {name: 'Bender'}
find(robots, function (robot, index, array) {
return robot.name === 'Fry';
});
// => undefined
Optionally pass in an object as third argument to use as this
when executing callback.
$ npm install array-find
MIT
FAQs
ES6 Array.find ponyfill. Return the first array element which satisfies a testing function.
The npm package array-find receives a total of 778,948 weekly downloads. As such, array-find popularity was classified as popular.
We found that array-find demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.