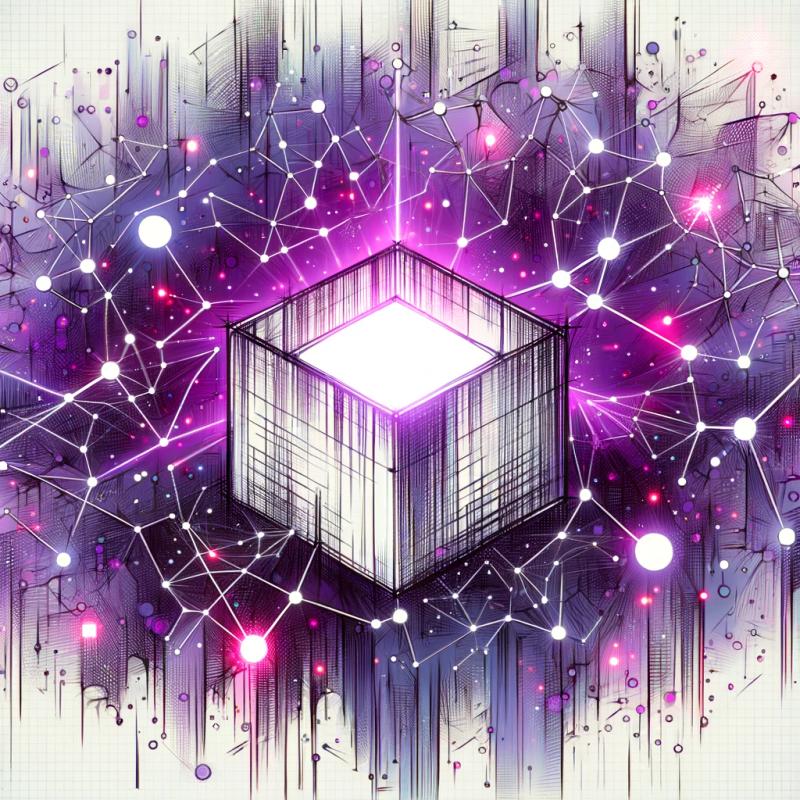
Security News
The AI Advantage: Reshaping Cybersecurity in the Age of Autonomous Threats
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.