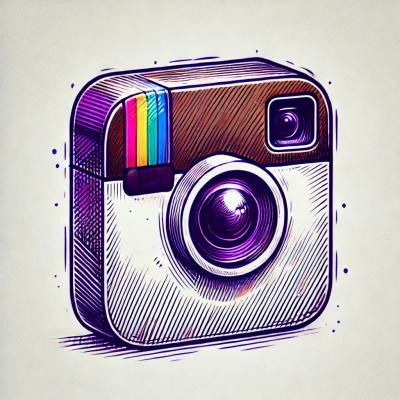
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
array-unique
Advanced tools
The array-unique npm package is a small utility that removes duplicate values from an array. It is useful when you need to ensure that an array contains only unique items without having to write custom deduplication logic.
Deduplicate an array
This feature allows you to remove duplicate values from an array, resulting in an array of only unique items. The `arrayUnique` function is used as a callback for the `filter` method.
[1, 2, 2, 3, 4, 4, 5, 5].filter(arrayUnique)
Lodash is a popular utility library that includes a `uniq` function. This function is similar to array-unique in that it removes duplicate values from an array. Lodash offers a wide range of additional utility functions, which makes it a more comprehensive solution than array-unique, but also larger in size.
Underscore.js is another utility library that provides a `uniq` function, similar to array-unique. It is comparable to Lodash in functionality and size, and it also offers a wide range of utility functions beyond array deduplication.
Ramda is a functional programming library that includes a `uniq` function. It is designed with a focus on functional programming patterns and immutability. Ramda's `uniq` function provides similar functionality to array-unique, but within the context of a larger functional programming toolkit.
Remove duplicate values from an array. Fastest ES5 implementation.
Install with npm:
$ npm install --save array-unique
var unique = require('array-unique');
var arr = ['a', 'b', 'c', 'c'];
console.log(unique(arr)) //=> ['a', 'b', 'c']
console.log(arr) //=> ['a', 'b', 'c']
/* The above modifies the input array. To prevent that at a slight performance cost: */
var unique = require("array-unique").immutable;
var arr = ['a', 'b', 'c', 'c'];
console.log(unique(arr)) //=> ['a', 'b', 'c']
console.log(arr) //=> ['a', 'b', 'c', 'c']
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
(This document was generated by verb-generate-readme (a verb generator), please don't edit the readme directly. Any changes to the readme must be made in .verb.md.)
To generate the readme and API documentation with verb:
$ npm install -g verb verb-generate-readme && verb
Install dev dependencies:
$ npm install -d && npm test
Jon Schlinkert
Copyright © 2016, Jon Schlinkert. Released under the MIT license.
This file was generated by verb-generate-readme, v0.1.28, on July 31, 2016.
FAQs
Remove duplicate values from an array. Fastest ES5 implementation.
The npm package array-unique receives a total of 14,681,005 weekly downloads. As such, array-unique popularity was classified as popular.
We found that array-unique demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.