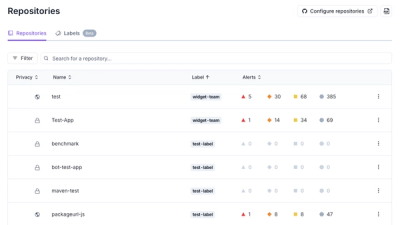
Product
Introducing Repository Labels and Security Policies
Socket is introducing a new way to organize repositories and apply repository-specific security policies.
Promises/A+ micro library to help with asynchronous work flow. The deferred methods match jQuery's when possible for convenience and familiarity, but under the hood it is a Promises/A+ spec!
Example
var deferred = assure();
deferred.done(function (arg) {
console.log("Outcome: " + arg);
});
deferred.always(function (arg) {
...
});
Promises (deferreds/futures/etc.) are a way to create a tangible connection between now
and an eventual outcome. Promises are a good pattern for asynchronous I/O, such as API interaction, AJAX operations, etc., by providing optional success
& failure
handling.
A then()
will return a new Promise which is in a hierarchal relationship. When a "parent" is reconciled, it's "children" inherit the outcome.
Registers a function to execute after Promise is reconciled
param {Function} arg Function to execute
return {Object} Deferred
Example
var deferred = assure();
deferred.always(function() {
console.log("This is always going to run");
});
Registers a function to execute after Promise is resolved
param {Function} arg Function to execute
return {Object} Deferred
Example
var deferred = assure();
deferred.done(function() {
console.log("This is going to run if Promise is resolved");
});
Registers a function to execute after Promise is rejected
param {Function} arg Function to execute
return {Object} Deferred
Example
var deferred = assure();
deferred.fail(function() {
console.log("This is going to run if Promise is rejected");
});
Determines if Deferred is rejected
return {Boolean} `true` if rejected
Example
var deferred = assure();
deferred.isRejected(); // false, it's brand new!
Determines if Deferred is resolved
return {Boolean} `true` if resolved
Example
var deferred = assure();
deferred.isResolved(); // false, it's brand new!
Breaks a Promise
param {Mixed} arg Promise outcome
return {Object} Promise
Example
var deferred = assure();
deferred.then(null, function (e) {
console.error(e);
});
deferred.reject("rejected");
Promise is resolved
param {Mixed} arg Promise outcome
return {Object} Promise
Example
var deferred = assure();
deferred.then(function (arg) {
console.log(arg);
});
deferred.resolve("resolved");
Gets the state of the Promise
return {String} Describes the state
Example
var deferred = assure();
deferred.state(); // `pending`
Registers handler(s) for a Promise
param {Function} success Executed when/if promise is resolved
param {Function} failure [Optional] Executed when/if promise is broken
return {Object} New Promise instance
Example
var deferred = assure();
deferred.then(function (arg) {
console.log("Promise succeeded!");
}, function (e) {
console.error("Promise failed!");
});
deferred.resolve("resolved");
Accepts Deferreds or Promises as arguments or an Array
return {Object} Deferred
Example
var d1 = assure(),
d2 = assure(),
d3 = assure();
...
when(d1,d2,d3).then(function (values) {
...
});
Copyright (c) 2013 Jason Mulligan
Licensed under the BSD-3 license.
FAQs
Promises/A+ micro library
The npm package assure receives a total of 15 weekly downloads. As such, assure popularity was classified as not popular.
We found that assure demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket is introducing a new way to organize repositories and apply repository-specific security policies.
Research
Security News
Socket researchers uncovered malicious npm and PyPI packages that steal crypto wallet credentials using Google Analytics and Telegram for exfiltration.
Product
Socket now supports .NET, bringing supply chain security and SBOM accuracy to NuGet and MSBuild-powered C# projects.