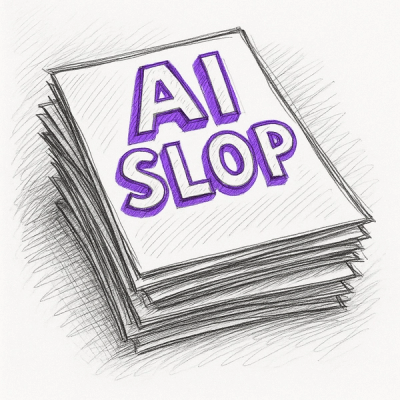
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Run a bunch of asynchronous functions and get all the values at once at the end!
var all = require('./')
function someAsyncThing(cb) {
setTimeout(function() {
cb(null, 'some sweet value')
}, 50)
}
function someOtherAsyncThing(cb) {
setTimeout(function() {
cb(null, 'some other value')
}, 10)
}
all({
someValue: someAsyncThing,
whatever: someOtherAsyncThing
}, function(err, results) {
results.someValue // => 'some sweet value'
results.whatever // => 'some other value'
})
all({
tmp: fs.stat.bind(fs, '/tmp'),
broken: fs.stat.bind(fs, '/tmp/doesntexist/asfarasIknow')
}, function(err, results) {
startsWith(err.message, 'ENOENT') // => true
})
npm install async-all
git clone https://github.com/TehShrike/async-all.git
cd async-all
npm test
FAQs
Like async-each, or q.all(), but with an object instead of an array
The npm package async-all receives a total of 0 weekly downloads. As such, async-all popularity was classified as not popular.
We found that async-all demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.