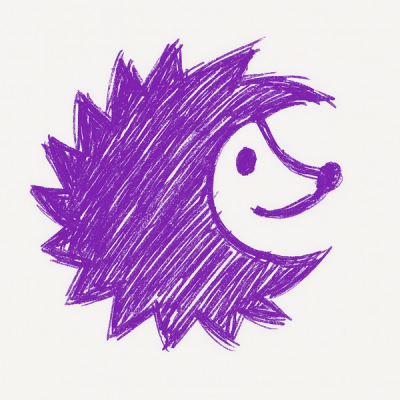
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
This is a wrapper for the popular csv
package in NPM that can be used with the ES7 async-await pattern, instead of using callbacks.
const csv = require('async-csv');
const fs = require('fs').promises;
(async() => {
// Read file from disk:
const csvString = await fs.readFile('./test.csv', 'utf-8');
// Convert CSV string into rows:
const rows = await csv.parse(csvString);
})();
For all documentation, please see the documentation for the csv package.
All parameters are the same as for the functions in the csv
module, except that you need to omit the callback parameter.
If there is any error returned by the csv
package, an exception will be thrown.
const csv = require('async-csv');
// `options` are optional
let result1 = await csv.generate(options);
let result2 = await csv.parse(input, options);
let result3 = await csv.transform(data, handler, options);
let result4 = await csv.stringify(data, options);
Feedback, bug reports and pull requests are welcome. See the linked Github repository.
FAQs
ES7 async-await wrapper for the csv package.
The npm package async-csv receives a total of 158,903 weekly downloads. As such, async-csv popularity was classified as popular.
We found that async-csv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.