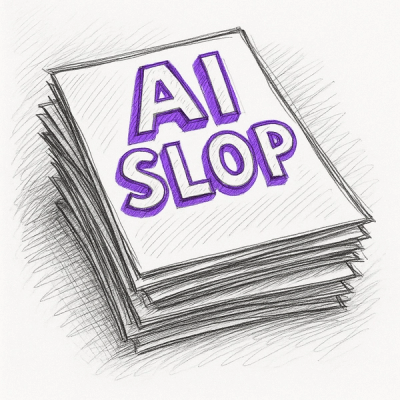
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
async-iterator-to-stream
Advanced tools
Convert an async iterator/iterable to a readable stream
Even though this library is dedicated to async iterators, it is fully compatible with synchronous ones.
Furthermore, generators can be used to create readable stream factories!
Installation of the npm package:
> npm install --save async-iterator-to-stream
const asyncIteratorToStream = require("async-iterator-to-stream");
// sync/async iterators
asyncIteratorToStream(new Set(["foo", "bar"]).values()).pipe(output);
// sync/async iterables
asyncIteratorToStream.obj([1, 2, 3]).pipe(output);
// if you pass a sync/async generator, it will return a factory instead of a
// stream
const createRangeStream = asyncIteratorToStream.obj(function*(n) {
for (let i = 0; i < n; ++i) {
yield i;
}
});
createRangeStream(10).pipe(output);
Let's implement a simpler fs.createReadStream
to illustrate the usage of this
library.
const asyncIteratorToStream = require("async-iterator-to-stream");
// promisified fs
const fs = require("mz/fs");
const createReadStream = asyncIteratorToStream(async function*(file) {
const fd = await fs.open(file, "r");
try {
let size = yield;
while (true) {
const buf = Buffer.alloc(size);
const [n] = await fs.read(fd, buf, 0, size, null);
if (n < size) {
yield buf.slice(0, n);
return;
}
size = yield buf;
}
} finally {
await fs.close(fd);
}
});
createReadStream("foo.txt").pipe(process.stdout);
If your environment does not support async generators, you may use a sync generator instead and
yield
promises to wait for them.
# Install dependencies
> npm
# Run the tests
> npm test
# Continuously compile
> npm run dev
# Continuously run the tests
> npm run dev-test
# Build for production
> npm run build
Contributions are very welcomed, either on the documentation or on the code.
You may:
ISC © Julien Fontanet
FAQs
Convert an async iterator/iterable to a readable stream
The npm package async-iterator-to-stream receives a total of 2,050 weekly downloads. As such, async-iterator-to-stream popularity was classified as popular.
We found that async-iterator-to-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.