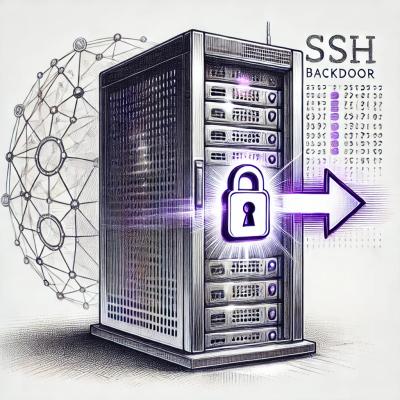
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Time async functions using async-done for execution and completion.
var EE = require('events').EventEmitter;
var createTimer = require('async-time');
var bus = new EE();
var asyncTime = createTimer(bus);
// listen for timing events
bus.on('start', function(evt){
// function has started
console.log(evt);
});
bus.on('stop', function(evt){
// function has stopped
console.log(evt);
});
asyncTime(function(cb){
// do async things
cb(null, 2);
}, function(err, res){
// `error` will be undefined on successful execution of the first function.
// `result` will be the result from the first function.
})
Once a timing function is created, it is used the same as async-done
.
createTimer(EventEmitter)
=> FunctionThe main export is a function that allows you to create a timing function.
You must pass it an EventEmitter
instance (or an object with emit
and on
methods) or it will throw.
The EventEmitter
instance is the bus timing events are published on.
asyncTime(fn, callback)
See async-done
docs.
start
The event fired when a function begins.
Properties:
id
: uuid generated for each function. Useful for tying start and end events together.name
: name property of the function given to asyncTime
.timestamp
: timestamp of when the function started.stop
The event fired when a function finishes.
Properties:
id
: uuid generated for each function. Useful for tying start and end events together.name
: name property of the function given to asyncTime
.timestamp
: timestamp of when the function started.duration
: high resolution time between start and stop events. Generated by process.hrtime(startTime)
FAQs
Time async functions using async-done for execution and completion.
We found that async-time demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.